2. Write a class named MonthDays. The class's constructor should accept two arguments: • An integer for the month (1 = January, 2 = February, etc.). • An integer for the year The class should have a method named getNumberOfDays that returns the number of days in the specified month. The method should use the following criteria to identify leap years: 1. Determine whether the year is divisible by 100. If it is, then it is a leap year if and if only it is divisible by 400. For example, 2000 is a leap year but 2100 is not. 2. If the year is not divisible by 100, then it is a leap year if and if only it is divisible by 4. For example, 2008 is a leap year but 2009 is not. Demonstrate the class in a program that asks the user to enter the month (letting the user enter an integer in the range of 1 through 12) and the year. The program should then display the number of days in that month. Here is a sample run of the program: Enter a month (1-12): 2 [Enter] Enter a year: 2008 [Enter] 29 days
2. Write a class named MonthDays. The class's constructor should accept two arguments: • An integer for the month (1 = January, 2 = February, etc.). • An integer for the year The class should have a method named getNumberOfDays that returns the number of days in the specified month. The method should use the following criteria to identify leap years: 1. Determine whether the year is divisible by 100. If it is, then it is a leap year if and if only it is divisible by 400. For example, 2000 is a leap year but 2100 is not. 2. If the year is not divisible by 100, then it is a leap year if and if only it is divisible by 4. For example, 2008 is a leap year but 2009 is not. Demonstrate the class in a program that asks the user to enter the month (letting the user enter an integer in the range of 1 through 12) and the year. The program should then display the number of days in that month. Here is a sample run of the program: Enter a month (1-12): 2 [Enter] Enter a year: 2008 [Enter] 29 days
Chapter6: Looping
Section: Chapter Questions
Problem 3GZ
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
![2. Write a class named MonthDays. The class's constructor should accept two arguments:
• An integer for the month (1 = January, 2= February, etc.).
• An integer for the year
The class should have a method named getNumberOfDays that returns the number of
days in the specified month. The method should use the following criteria to identify leap
years:
1. Determine whether the year is divisible by 100. If it is, then it is a leap year if and if only
it is divisible by 400. For example, 2000 is a leap year but 2100 is not.
2. If the year is not divisible by 100, then it is a leap year if and if only it is divisible by 4.
For example, 2008 is a leap year but 2009 is not.
Demonstrate the class in a program that asks the user to enter the month (letting the
user enter an integer in the range of 1 through 12) and the year. The program should then
display the number of days in that month. Here is a sample run of the program:
Enter a month (1-12): 2 [Enter]
Enter a year: 2008 [Enter]
29 days](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F66b15059-8d41-4db3-96d9-e8acaa80bca2%2F5a4bf295-de07-45d2-a6be-a71bb5f849ae%2Fk4g6z9_processed.jpeg&w=3840&q=75)
Transcribed Image Text:2. Write a class named MonthDays. The class's constructor should accept two arguments:
• An integer for the month (1 = January, 2= February, etc.).
• An integer for the year
The class should have a method named getNumberOfDays that returns the number of
days in the specified month. The method should use the following criteria to identify leap
years:
1. Determine whether the year is divisible by 100. If it is, then it is a leap year if and if only
it is divisible by 400. For example, 2000 is a leap year but 2100 is not.
2. If the year is not divisible by 100, then it is a leap year if and if only it is divisible by 4.
For example, 2008 is a leap year but 2009 is not.
Demonstrate the class in a program that asks the user to enter the month (letting the
user enter an integer in the range of 1 through 12) and the year. The program should then
display the number of days in that month. Here is a sample run of the program:
Enter a month (1-12): 2 [Enter]
Enter a year: 2008 [Enter]
29 days
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
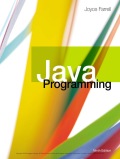
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
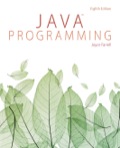
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
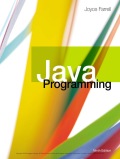
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
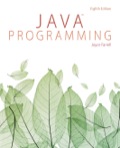
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
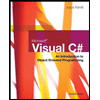
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,