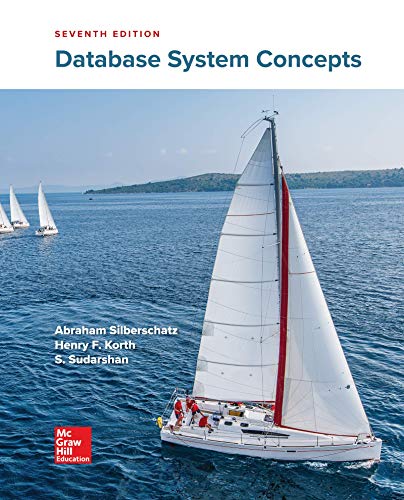
Concept explainers
a Java program that defines and uses 3 overloaded methods (the Java file name
should be “MethodOverload.java” and all the 3 methods should have the same method
name max). The 3 method headers are given below with their definitions:
• int max(int n1, int n2, int n3):
o to return the maximum of the passed 3 integer arguments.
• double max(double d1, double d2, double d3):
o to return the maximum of the passed 3 double arguments with use of
Math.max() method—that is, do not use if or if-else statement in
comparing the numbers to find the smaller/smallest numbers.
• int max(int n):
In the main method of the application, a loop with switch-statement inside is required for
testing the different method calls and you need to generate random numbers for the inputs
as the arguments for the methods (except for die-rolling case where an input is entered
from the keyboard.). Specifically:
• int max(int n1, int n2, int n3): the 3 random integers should be from the sequence
2, 5, 8, 11, 14.
• double max(double d1, double d2, double d3): the 3 random double numbers
should be from the range [10, 20].
o Hint: a random number from the range [min, max] is generated through
min + (max-min) * randomNumbers.nextDouble();
• int max(int n): the input for the number of rolls n is entered from the keyboard.
![Write a Java program that defines and uses 3 overloaded methods (the Java file name
should be “MethodOverload.java" and all the 3 methods should have the same method
name max). The 3 method headers are given below with their definitions:
• int max(int n1, int n2, int n3):
o to return the maximum of the passed 3 integer arguments.
• double max(double d1, double d2, đouble d3):
o to return the maximum of the passed 3 double arguments with use of
Math.max() method-that is, do not use if or if-else statement in
comparing the numbers to find the smaller/smallest numbers.
int max(int n):
In the main method of the application, a loop with switch-statement inside is required for
testing the different method calls and you need to generate random numbers for the inputs
as the arguments for the methods (except for die-rolling case where an input is entered
from the keyboard.). Specifically:
• int max(int n1, int n2, int n3): the 3 random integers should be from the sequence
2, 5, 8, 11, 14.
• double max(double d1, double d2, double d3): the 3 random double numbers
should be from the range [10, 20].
• Hint: a random number from the range [min, max] is generated through
min + (max-min) * randomNumbers.nextDouble();
int max(int n): the input for the number of rolls nis entered from the keyboard.
(See a testing sample of this program on next page.)](https://content.bartleby.com/qna-images/question/36b85914-2744-42f7-b8bd-af21a659e2ca/dea41161-952e-4efc-ad08-a8e8d6c0167f/7gxdjtn_thumbnail.png)
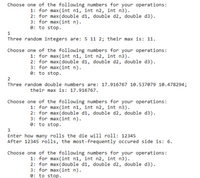

Step by stepSolved in 4 steps with 6 images

- In Java,thank you.arrow_forwardFinancial Application:• Write a program that computes future investment value at a given interest rate for aspecified number of months and prints the report shown in the sample output. • Given the annual interest rate, the interest amount earned each month is computedusing the formula: Interest earned = investment amount * annual interest rate /1200 (=months * 100) • Write a method, computeFutureValue, which receives the investment amount, annualinterest rate and number of months as parameters and does the following: o Prints the interest amount earned each month and the new value of theinvestment (hint: use a loop). o Returns the total interest amount earned after the number of months specified bythe user. The main method will:o Ask the user for all input needed to call the computeFutureValue method.o Call computeFutureValueo Print the total interest amount earned by the investment at the end of thenumber of months entered by the user. Sample Program runningEnter the investment…arrow_forwardJava Scriptarrow_forward
- In java just add code between the comments public class A6 {/*** In this Java file, you will write three methods that will ensure that the calls in the main methods are* properly executed. You do NOT have to change anything in the main() method. You have to write the methods that* will complete the program.** Pay attention to the method name, the method return type, and the method parameters.*/public static void main(String[] args) {/*Your first method will ask user to enter two values and return the exponent where the firstnumber will be the base and the second will be the exponent. Remember to use a mathematical function.If the user enters value 1 as 2 and value 2 as 3, output of your method should be 8 (2 raised to power 3)*/double calculatedExponent = exponentCalculator();System.out.println(calculatedExponent); //this should print out the exponent value/* Your second method will have two parameters. The first is a String and the second is a number. The methodshould return the…arrow_forwardPython Programming ONLY PLEASE NO JAVA Use recursion to determine the number of digits in an integer n. Hint: If n is < 10, it has one digit. Otherwise, it has one more digit than n / 10. Write the method and then write a test program to test the method Your test program must: Ask the user for a number Print the number of digits in that number. (describe the numbers printed to the console) Use program headers and method header comments in your code.arrow_forwardProgram to compute interest = p * r * t, where p = $10K, rate = 6%, t = 10 years; these values are to be passed from main() Besides main() the program has two methods: one that computes the interest and on that rounds interest to two decimal places public class DisplayInterest public static void main(String[] args) call a method that will do the computation with values for the above variables; with the exception of year, they are double main() displays computed interest after rounding computation method() rounding method() Note:- Please type and execute this java program and also need an output for this java program.arrow_forward
- Understanding ifStatements Summary In this lab, you complete a prewritten Java program for a carpenter who creates personalized house signs. The program is supposed to compute the price of any sign a customer orders, based on the following facts: The charge for all signs is a minimum of $35.00. The first five letters or numbers are included in the minimum charge; there is a $4 charge for each additional character. If the sign is made of oak, add $20.00. No charge is added for pine. Black or white characters are included in the minimum charge; there is an additional $15 charge for gold-leaf lettering. Instructions 1. Ensure the file named HouseSign.java is open. 2. You need to declare variables for the following, and initialize them where specified: A variable for the cost of the sign initialized to 0.00 (charge). A variable for the number of characters initialized to 8 (numChars). A variable for the color of the characters initialized to "gold" (color). A variable for the…arrow_forwardProgram63.javaWrite a program that estimates the cost of carpet for one or more rooms with rectangular floors. Begin by prompting for the price of carpet per square yard and the number of rooms needing this carpet. Use a loop to prompt for the floor dimensions of each room in feet. In this loop, call a value-returning method with the dimensions and carpet price as arguments. The method should return the carpet cost for each room to main, where it will be printed and accumulated. After all rooms have been processed, the program should display the total cost of the job.Sample Output (image below) Program64.javaWrite a program that demonstrates method overloading by defining and calling methods that return the area of a triangle, a rectangle, or a square.arrow_forwardNeed help with this java program.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
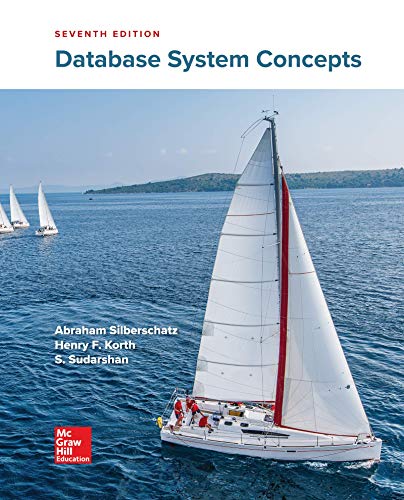
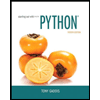
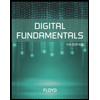
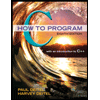
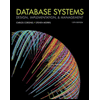
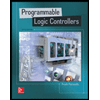