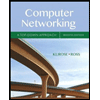
Add the quicksort
# selectionsortanimation.py
"""Animated selection sort visualization."""
from matplotlib import animation
import matplotlib.pyplot as plt
import numpy as np
import seaborn as sns
import sys
#from ch11soundutilities import play_sound
def update(frame_data):
"""Display bars representing the current state."""
# unpack info for graph update
data, colors, swaps, comparisons = frame_data
plt.cla() # clear old contents of current Figure
# create barplot and set its xlabel
bar_positions = np.arange(len(data))
axes = sns.barplot(bar_positions, data, palette=colors) # new bars
axes.set(xlabel=f'Comparisons: {comparisons}; Swaps: {swaps}',
xticklabels=data)
def flash_bars(index1, index2, data, colors, swaps, comparisons):
"""Flash the bars about to be swapped and play their notes."""
for x in range(0, 2):
colors[index1], colors[index2] = 'white', 'white'
yield (data, colors, swaps, comparisons)
colors[index1], colors[index2] = 'purple', 'purple'
yield (data, colors, swaps, comparisons)
#play_sound(data[index1], seconds=0.05)
#play_sound(data[index2], seconds=0.05)
def selection_sort(data):
"""Sort data using the selection sort algorithm and
yields values that update uses to visualize the algorithm."""
swaps = 0
comparisons = 0
colors = ['lightgray'] * len(data) # list of bar colors
# display initial bars representing shuffled values
yield (data, colors, swaps, comparisons)
# loop over len(data) - 1 elements
for index1 in range(0, len(data) - 1):
smallest = index1
# loop to find index of smallest element's index
for index2 in range(index1 + 1, len(data)):
comparisons += 1
colors[smallest] = 'purple'
colors[index2] = 'red'
yield (data, colors, swaps, comparisons)
#play_sound(data[index2], seconds=0.05)
# compare elements at positions index and smallest
if data[index2] < data[smallest]:
colors[smallest] = 'lightgray'
smallest = index2
colors[smallest] = 'purple'
yield (data, colors, swaps, comparisons)
else:
colors[index2] = 'lightgray'
yield (data, colors, swaps, comparisons)
# ensure that last bar is not purple
colors[-1] = 'lightgray'
# flash the bars about to be swapped
yield from flash_bars(index1, smallest, data, colors,
swaps, comparisons)
# swap the elements at positions index1 and smallest
swaps += 1
data[smallest], data[index1] = data[index1], data[smallest]
# flash the bars that were just swapped
yield from flash_bars(index1, smallest, data, colors,
swaps, comparisons)
# indicate that bar index1 is now in its final spot
colors[index1] = 'lightgreen'
yield (data, colors, swaps, comparisons)
# indicate that last bar is now in its final spot
colors[-1] = 'lightgreen'
yield (data, colors, swaps, comparisons)
#play_sound(data[-1], seconds=0.05)
# play each bar's note once and color it darker green
for index in range(len(data)):
colors[index] = 'green'
yield (data, colors, swaps, comparisons)
#play_sound(data[index], seconds=0.05)
def main():
number_of_values = int(sys.argv[1]) if len(sys.argv) == 2 else 10
figure = plt.figure('Selection Sort') # Figure to display barplot
numbers = np.arange(1, number_of_values + 1) # create array
np.random.shuffle(numbers) # shuffle the array
# start the animation
anim = animation.FuncAnimation(figure, update, repeat=False,
frames=selection_sort(numbers), interval=50)
plt.show() # display the Figure
# call main if this file is executed as a script
if __name__ == '__main__':
main()
Example of the quick sore algoritim is below
class QuickSort:
# sort the array in it is location in the memory .
@classmethod
# l > low, h > high
def quickSort(cls,array,l,h):
if l < h :
pi =cls.qsort_helper(array, l, h)
# sort the smaller part
cls.quickSort(array, l, pi - 1)
# sort the greater part
cls.quickSort(array, pi + 1, h)
@classmethod
def qsort_helper(cls,array,l,h):
p = array[h]
# print(p)
low = l - 1
# i > poiter for greater element
for j in range(l, h):
# find the smaller element of the pivot, if found swap it with the low element
if array[j] < p :
low += 1
(array[low], array[j]) = (array[j], array[low])
(array[low + 1], array[h]) = (array[h], array[low + 1])
return low + 1
def main ():
array = [5, 8, 5,4, 6, 978, 9, 0, 47, 0, 4, 9]
QuickSort.quickSort(array, 0, len(array) - 1)
print(array)
main()

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Is there a way you can put the output of the code? And also indent the code.
Is there a way you can put the output of the code? And also indent the code.
- In python, write a function own_filter where it can flip an image vertically or set an image to a red color.arrow_forwardplease help with python program I am providng program that some part u can reuse for question nubmer 2 is attched in image # call the appropriate libraries import numpy as np from numpy import mean from numpy import std from numpy.random import randn from numpy.random import seed from matplotlib import pyplot from numpy import cov from scipy.stats import pearsonr from scipy.stats import spearmanr import scipy.stats as stats import pandas as pd # generate data # seed random number generator seed(1) # prepare data data1 = 20 * randn(1000) + 100 data2 = data1 + (10 * randn(1000) + 50) # print mean and std print('data1: mean=%.3f stdv=%.3f' % (mean(data1), std(data1))) print('data2: mean=%.3f stdv=%.3f' % (mean(data2), std(data2))) # plot the sample data pyplot.scatter(data1, data2) pyplot.show() # calculate covariance covariance = cov(data1, data2) print('The covariance matrix') print(covariance) # calculate Pearson's correlation corr, _ = pearsonr(data1, data2) print('Pearsons…arrow_forwardCan you fix the error in the python code. import numpy as npimport matplotlib.pyplot as plt N = 20 # number of points to discretizeL = 0.15X = np.linspace(0, L, N) # position along the rodh = L / (N - 1) # discretization spacing C0t = 0.200 # concentration at x = 0Cth = 0.00000409D = 0.0000025t_avg = 0n = 0 tfinal = 300Ntsteps = 1000dt = tfinal / (Ntsteps - 1)t = np.linspace(0, tfinal, Ntsteps) alpha =( D * dt / h**2) C_xt = [] # container for all the time steps # initial condition at t = 0C = np.zeros(X.shape)C[0] = C0t C_xt += [C] for j in range(1, Ntsteps): N = np.zeros(C.shape) N[0] = C0t N[1:-1] = alpha*C[2:] + (1 - 2 * alpha) * C[1:-1] + alpha * C[0:-2] N[-1] = N[-2] # derivative boundary condition flux = 0 C[:] = N C_xt += [N] if ((Cth-0.000001) < N[-1]<(Cth+0.000001)): print ('Time=',t[j],'conc=',[N[-1]],'Cthr=',[Cth]) t_avg = t_avg+t[j] n = n + 1 # plot selective solutions if j in…arrow_forward
- x = zeros(uint8(5,5)); for i = 1:5 for j = 1:5 a = 5/i; x(i,j) = 255/a; end end Which of the following best describes the black and white image x? 1. The columns of x will get lighter from left to right 2. The rows of x will get lighter from top to bottom 3. The rows of x will get darker from top to bottom 4. The columns of x will get darker from left to rightarrow_forwardI am trying to loop through several FITS files that are located on my Desktop. This is the code I have so far. The final image that i need to produce is a "single plot". with multiple graphs overlayed (see image) import numpy as npimport matplotlib.pyplot as pltimport globfrom astropy.io import fitsfrom astropy.wcs import WCSfrom pathlib import Path%matplotlib inline%matplotlib widget plt.figure(figsize=(5,5))legends = [] def plot_fits_file(file_path): # used this data to test ---------- # lam = np.random.random(100) # flux = np.random.random(100) # -------------------- # below code will work when you have file # all the plot will be on single chart hdul = fits.open(file_path) data = hdul[1].data h1 = hdul[1].header flux = data[1] w = WCS(h1, naxis=1, relax=False, fix=False) lam = w.wcs_pix2world(np.arange(len(flux)), 0)[0] file_path = Path(file_path)if file_path.exists(): plot_fits_file(file_path) plt.show()else:…arrow_forward27. Continue the source code below to complete the house shape! import turtle t - turtle. Turtle () t.fd (227) t.lt (90) t.fd(100) t.rt (90) t.fd(50) t.lt (145) t.fd (200) #complete the source code Python Turtie Graphics AL.lt (70) t.fa(100) t.rt (90) t.fa (50) t.rt (145) t.fd (200) t.lt (145) t.fd(200) t.lt (90) t.fd(100) B t.rt (90) t. fd(100) xC t.lt (70) t. fd (200) t.lt (145) t. fd (50) t.rt (90) t. fa (100) t.lt (145) t. fd (50) t.lt (90) t. fd(100) t.rt (90) t.fd(100) Darrow_forward
- Using code in C u can open image in new tab so can you can see clearly because I can only post 2 images SONGS LIST IDGAF - Dua LipaFRIENDS - Marshmello, Anne-MarieThe Middle - Zedd, Maren Morris, GreyBest Part - H.E.R., Daniel CaesarAll The Stars (with SZA) - Kendrick Lamar, LZAWolves - Selena Gomez, MarshmelloGod's Plan - DrakeRewrite The Stars - Zac Efron, ZendayaHavana - Camila Cabello, Young ThugPerfect - Ed Sheeranarrow_forwardWrite an algorithm that outline how to calculate the room area for the image belowarrow_forwardUsing python at jupiter lab make a code for image classification with animal images and execute it Please, i would be grateful if you attach output screenshoot Thank youarrow_forward
- For this code please add these features making it be able to : #generate a blank image with black background, picture size is the same as frame #allow it to calculate center point #Draw white circles into the image generated at line 6, diameter is 6arrow_forwardHow do we add text, shapes, and other annotations to Matplotib piots? O By passing an indcator variable indicating the quadrant of the gyraph for annotation to the correspor function or method By passing the (xy) coordinates to the corresponding function or method O By passing the asis labets for each object to the corresponding function or method O By passing a lst of strings to the corresponding function or methodarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
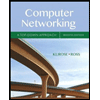
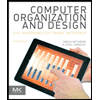
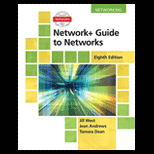
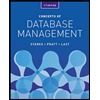
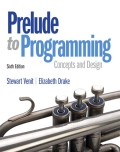
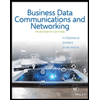