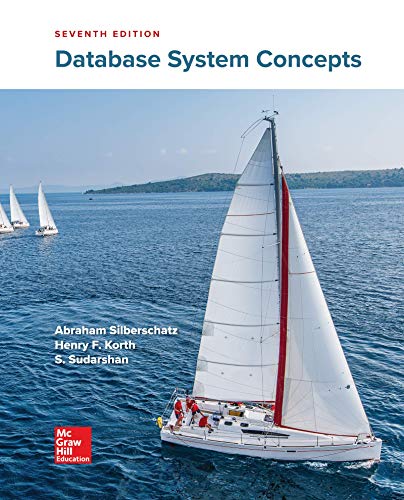
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
C++ CODE PLEASE
Using an array to represent the min-max heap structure , implement the following operations. (in c++ programming language)
1. buildHeap : Builds a min-max heap from a list of naturals read from standard input.
2. findMin and findMax : Returns the minimum (resp the maximum) element.
3. insertHeap : Inserts a new element into the min-max heap.
4. deleteMin and deleteMax : Deletes the minimum (resp the maximum) element.
NOTE: min-max should be in one heap structure only and not separate heap structure for min and max. Only one heap for both min and max. Root should be min level, the level below root should be max level,then level after that should again be min level and so on.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- #include <iostream> #include <queue> //This contains the STL's efficient implementation of a priority queue using a heap using namespace std; /* In this lab you will implement a data structure that supports 2 primary operations: - insert a new item - remove and return the smallest item A data structure that supports these two operations is called a "priority queue". There are many ways to implement a priority queue, with differernt efficiencies for the two primary operations. For this lab, please do not attempt (at least at first) to implement something fancy (like a heap). Just use your mind to do something simple. Analyze the efficiency of both insertion and removal for your implementation. Afterwards, feel free to investigagte "min heaps" to see a very clever and fast priority queue implementation. Feel free to try to implement it. After implementuing your priority queue, use it to implement a sorting algorithm (priorityQueueSort). Analyze the run time of your sorting…arrow_forwardplease helparrow_forwardstruct insert_at_back_of_sll { // Function takes a constant Book as a parameter, inserts that book at the // back of a singly linked list, and returns nothing. void operator()(const Book& book) { /// TO-DO (3) /// // Write the lines of code to insert "book" at the back of "my_sll". Since // the SLL has no size() function and no tail pointer, you must walk the // list looking for the last node. // // HINT: Do not attempt to insert after "my_sll.end()". // ///// END-T0-DO (3) ||||// } std::forward_list& my_sll; };arrow_forward
- Data Structure & Algorithm: Describe and show by fully java coded example how a hash table works. You should test this with various array sizes and run it several times and record the execution times. At least four array sizes are recommended. 10, 15, 20, 25. It is recommended you write the classes and then demonstrate/test them using another classarrow_forward#include <iostream> using namespace std; #define SIZE 5 //creating the queue using array int A[SIZE]; int front = -1; int rear = -1; //function to check if the queue is empty bool isempty() { if(front == -1 && rear == -1) return true; else return false; } //function to enter elements in queue void enqueue ( int value ) { //if queue is full if ((rear + 1)%SIZE == front) cout<<"Queue is full \n"; else { //now the first element is inserted if( front == -1) front = 0; //inserting element at rear end rear = (rear+1)%SIZE; A[rear] = value; } } //function to remove elements from queue void dequeue ( ) { if( isempty() ) cout<<"Queue is empty\n"; else //only one element if( front == rear ) front = rear = -1; else front = ( front + 1)%SIZE; } //function to show the element at front void showfront() { if( isempty()) cout<<"Queue is empty\n"; else cout<<"element at front is:"<<A[front]; } //function to display the queue void…arrow_forwardJava - This project will allow you to compare & contrast different 4 sorting techniques, the last of which will be up to you to select. You will implement the following: Bubble Sort (pair-wise) Bubble Sort (list-wise) [This is the selection sort] Merge Sort Your choice (candidates are the heap, quick, shell, cocktail, bucket, or radix sorts) [These will require independent research) General rules: Structures can be static or dynamic You are not allowed to use built in methods that are direct or indirect requirements for this project – You cannot use ANY built in sorting functions - I/O (System.in/out *) are ok. All compare/swap/move methods must be your own. (You can use string compares) Your program will be sorting names – you need at least 100 unique names (you can use the 50 given in project #3) – read them into the program in a random fashion (i.e. not in any kind of alpha order). *The more names you have, the easier it is to see trends in speed. All sorts will be from…arrow_forward
- Python Big-O Notation/Time Complexity Pls answer only if u know big-o Thanks! Item #3.arrow_forwardProject 2: Singly-Linked List The purpose of this assignment is to assess your ability to: ▪Implement sequential search algorithms for linked list structures ▪Implement sequential abstract data types using linked data ▪Analyze and compare algorithms for efficiency using Big-O notation For this assignment, you will implement a singly-linked node class. Use your singly-linked node to implement a singly-linked list class that maintains its elements in ascending order. The SinglyLinkedList class is defined by the following data: ▪A node pointer to the front and the tail of the list Implement the following methods in your class: ▪A default constructor list<T> myList ▪A copy constructor list<T> myList(aList) ▪Access to first elementmyList.front() ▪Access to last elementmyList.back() ▪Insert value myList.insert(val) ▪Remove value at frontmyList.pop_front() ▪Remove value at tailmyList.pop_back() ▪Determine if emptymyList.empty() ▪Return # of elementsmyList.size() ▪Reverse order of…arrow_forwardComputer Sciencearrow_forward
- 4) Priority Queue Application Homework • Unanswered Situation: The order in which patients at a certain emergency room are seen by the doctor is based on arrival time minus 10 minutes for each major wound, minus 5 minutes for each minor wound, and plus 3 minutes for each time the patient annoys the nurse. You can assume a maximum wait time of 2 days. If a priority queue is used in the software at the nurses' station, would you use a minimum heap or a maximum heap to implement the priority queue? (Thought-provoker: Do you think if you annoyed the nurse enough, s/he would smack you around enough for you to move to the head of the line?)arrow_forwardCircular Lists - C PROGRAM A linear list is being maintained circularly in an array c[0…n-1] with front, and rear set up as for circular queues. a) Draft a formula/algorithm in terms of front, rear, and n for the number of elements in the list.arrow_forwardStack: Stacks are a type of container with LIFO (Last In First Out) type of working, where a new element is added at one end and (top) an element is removed from that end only. Your Stack should not be of the fixed sized. It should be able to grow itself. bool empty() : Returns whether the Stack is empty or not. Time Complexity should be: O(1) bool full() : Returns whether the Stack is full or not. Time Complexity should be: O(1)int size() : Returns the current size of the Stack. Time Complexity should be: O(1)Type top () : Returns the last element of the Stack. Time Complexity should be: O(1) void push(Type) : Adds the element of type Type at the top of the stack. Time Complexity should be: O(1) Type pop() : Deletes the top most element of the stack and returns it. Time Complexity should be: O(1) Write non-parameterized constructor for the above class. Write Copy constructor for the above class. Write Destructor for the above class. Now write a global function show stack which…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
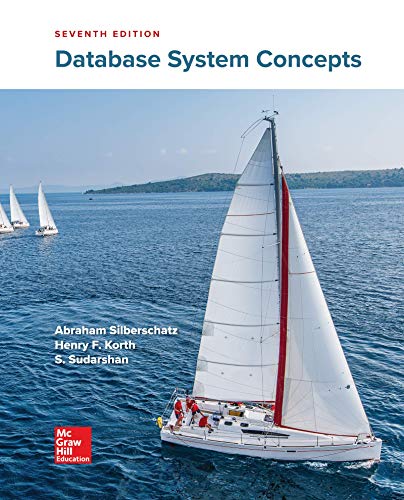
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
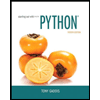
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
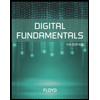
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
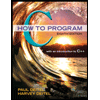
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
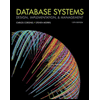
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
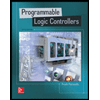
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education