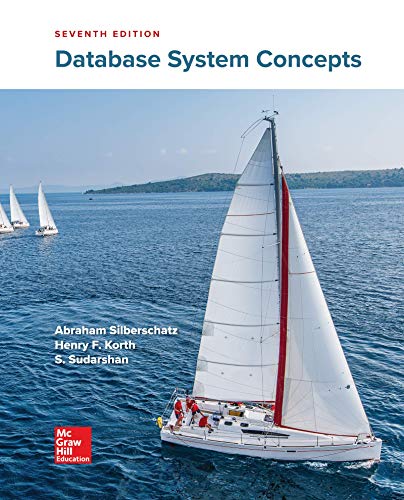
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
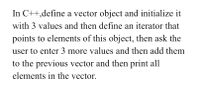
Transcribed Image Text:**C++ Vector Manipulation Tutorial**
In this tutorial, we will learn how to define a vector object in C++ and initialize it with three values. We will also define an iterator to traverse the elements of the vector. Following this, we will prompt the user to enter three additional values, which will be added to the existing vector. Finally, we will print all the elements contained within the vector.
**Steps:**
1. **Define a Vector Object:**
- Initialize the vector with three values.
2. **Create an Iterator:**
- Define an iterator to point to elements of the vector for traversal.
3. **Add User Input:**
- Ask the user to input three additional values.
- Append these values to the existing vector.
4. **Print Vector Elements:**
- Use the iterator to print all elements within the vector.
By following these steps, you will understand basic vector operations in C++, including initialization, iteration, updating, and outputting elements.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write the lines of code to remove the book at the back of "my_vector". Remember, attempting to remove an element from an empty data structure is a logic error. Include code to avoid that.arrow_forwardIn C++, define an integer vector and ask the user to give you values for the vector. Because you used a vector, so you don't need to know the size. user should be able to enter a number until he wants(you can use while loop for termination. for example if the user enters any negative number, but until he enters a positive number, your program should read and push it inside of the vector). the calculate the average of all numbers he entered.arrow_forwardC++ Program For this program you’ll write a simple class and use it in a vector.Begin by writing a Student class. The public section has the following methods: Student::Student()Constructor. Initializes all data elements: name to empty string(s), numeric variables to 0. bool Student::ReadData(istream& in)Data input. The istream should already be open. Reads the following data, in this order: First name (a string, 1 word) Last name (also a string, also 1 word) 5 quiz scores (integers, range 0-20) 3 exam scores (integers, range 0-100) Assumes that all data is separated by whitespace. The method returns true if reading all data was successful, otherwise returns false. Does not need to validate or range-check data; if one of the quiz or exam scores is out of range, just keep going. bool Student::WriteData(ostream& out) constOutput function. Writes data in the following format. Each student’s data is on one line. • First name (left justified, 20 characters)• Last name (left…arrow_forward
- Computer Sciencearrow_forwardEvery data structure that we use in computer science has its weaknesses and strengthsHaving a full understanding of each will help make us better programmers!For this experiment, let's work with STL vectors and STL dequesFull requirements descriptions are found in the source code file Part 1Work with inserting elements at the front of a vector and a deque (30%) Part 2Work with inserting elements at the back of a vector and a deque (30%) Part 3Work with inserting elements in the middle, and removing elements from, a vector and a deque (40%) Please make sure to put your code specifically where it is asked for, and no where elseDo not modify any of the code you already see in the template file This C++ source code file is required to complete this problemarrow_forwardSelect all code lines that creates a vector with content {10, 10, 10, 10). You are also given the C-type array, an_array with content (10, 10, 10, 10). std::vector a_vector (an_array, an_array + sizeof(an_array)/sizeof(int)); std::vector a_vector [10, 10, 10, 10); std::vector a_vector (10, 4); std::vector a_vector = {10, 10, 10, 10); Ostd::vector a_vector (4, 10);arrow_forward
- #include <iostream>#include <vector>using namespace std; void PrintVectors(vector<int> numsList) { unsigned int i; for (i = 0; i < numsList.size(); ++i) { cout << numsList.at(i) << " "; } cout << endl;} int main() { vector<int> numsList; int userInput; int i; for (i = 0; i < 3; ++i) { cin >> userInput; numsList.push_back(userInput); } numsList.erase(numsList.begin()+1); numsList.insert(numsList.begin()+1, 102); numsList.insert(numsList.begin()+1, 100); PrintVectors(numsList); return 0;} Not all tests passed clearTesting with inputs: 33 200 10 Output differs. See highlights below. Special character legend Your output 33 100 102 10 Expected output 100 33 102 10 clearTesting with inputs: 6 7 8 Output differs. See highlights below. Special character legend Your output 6 100 102 8 Expected output 100 6 102 8 Not sure what I did wrong but the the 33…arrow_forwardplease help me with codingarrow_forwardneed help in C++ Problem: You are asked to create a program for storing the catalog of movies at a DVD store using functions, files, and user-defined structures. The program should let the user read the movie through the file, add, remove, and output movies to the file. For this assignment, you must store the information about the movies in the catalog using a single vector. The vector's data type is a user-defined structure that you must define on functions.h following these rules: Identifier for the user-define structure: movie. Member variables of the structure "movie": name (string), year (int), and genre (string). Note: you must use the identifiers presented before when defining the user-defined structure. Your solution will NOT pass the unit test cases if you do not follow the instructions presented above. The main function is provided (you need to modify the code of the main function to call the user-defined functions described below). The following user-defined functions are…arrow_forward
- Add each element in origList with the corresponding value in offsetAmount. Print each sum followed by a space. Ex: If origList = {40, 50, 60, 70} and offsetAmount = {5, 7, 3, 0}, print: 45 57 63 70 #include <iostream>#include <vector>using namespace std; int main() {const int NUM_VALS = 4;vector<int> origList(NUM_VALS);vector<int> offsetAmount(NUM_VALS);unsigned int i; for (i = 0; i < origList.size(); ++i) {cin >> origList.at(i);} for (i = 0; i < offsetAmount.size(); ++i) {cin >> offsetAmount.at(i);} cout << endl; return 0;} Please help me with this problem using c++.arrow_forwardcreate using c++ One problem with dynamic arrays is that once the array is created using the new operator the size cannot be changed. For example, you might want to add or delete entries from the array similar to the behavior of a vector . This project asks you to create a class called DynamicStringArray that includes member functions that allow it to emulate the behavior of a vector of strings. The class should have the following A private member variable called dynamicArray that references a dynamic array of type string. A private member variable called size that holds the number of entries in the array. A default constructor that sets the dynamic array to NULL and sets size to 0. A function that returns size . A function named addEntry that takes a string as input. The function should create a new dynamic array one element larger than dynamicArray , copy all elements from dynamicArray into the new array, add the new string onto the end of the new array, increment size, delete the…arrow_forwardIn C++ please follow the instructions Write two code blocks -- one code block to declare a bag and its companion type-tracking array (both using the STL vector), and another code block to create and declare a Cat object and put it into the bag. Name the arrays and variables as you wish. Use any data type tracking and any designation for cats -- your choices. Assume that struct Cat is already defined and that all required libraries are properly included -- just write the two separate code blocks, separated by one or more blank lines.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
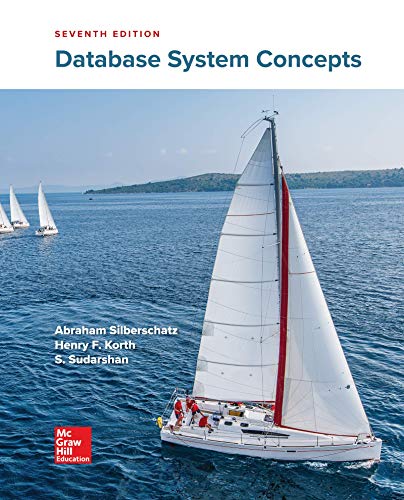
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
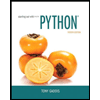
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
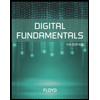
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
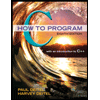
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
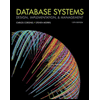
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
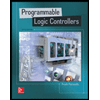
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education