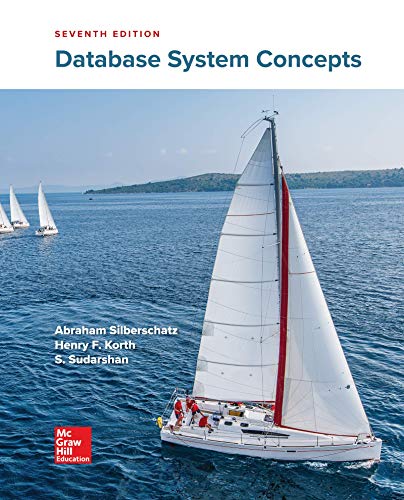
Concept explainers
Java
Conway’s game of life is a simple simulation of life forms in a two dimensional grid. The ConwayCell implements the standard behavior of a call in Conway’s Game of Life. You only need to implement and test two new classes. You will create two different cells, derived from AbstractCell.
1. Alternating Cell
The first class that you should create is a class named AlternatingCell. This is a cell that should alternate between being alive and dead with each generation. Add one or more of your AlternatingCells to your ConwayWorld. You can see how to add a cell in line 15 - 20 of Main.java. Give your AlternatingCell a unique display character when it is alive so that you can see it in your conway world.
2. Your own cell
For the second cell you should feel free to implement any behavior that you want. If you are having trouble thinking of ideas then post up a question.
You can add your custom cell in just a few places in the world, or you might choose to replace all of the ConwayCells with your own cell. If you would like to replace all of the ConwayCells then you'll need to modify the line in the ConwyWorld constructor that instantiates the first ConwayCells:
public ConwayWorld() {
for (int r = 0; r < ROWS; r++) {
for (int c = 0; c < COLS; c++) {
grid[r][c] = new MyCustomCell(r, c, this);
}
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Class Triangle ï A Triangle will have 3 sides. The class will be able to keep track of the number of Triangle objects created. It will also hold the total of the perimeters of all the Triangle objects created. ï It will allow a client to create a Triangle, passing in integer values for the three sides into the Triangle constructor. If the values for the sides that are passed in do not represent a valid Triangle (read below for the requirements for a Triangle to be valid) , then all sides will be set to a value of 1. The constructor should add 1 to the count of the number of Triangles created. The constructor should call a method to calculate the perimeter and then add the perimeter for that object to an accumulator. In addition to the constructor, the Triangle class must have the following methods that return a boolean value: isRight () - see note 1 below regarding Acute triangles. isAcute() - see note 1 below regarding Acute triangles. isObtuse() - see note…arrow_forwardPython:Please read the following. The Game class The Game class brings together an instance of the GameBoard class, a list of Player objects who are playing the game, and an integer variable “n” indicating how many tokens must be aligned to win (as in the name “Connect N”). The game class has a constructor and two additional methods. The most complicated of these, play, is provided for you and should not be modified. However, you must implement the constructor and the add_player method. __init__(self,n,height,width) The Game constructor needs to initialize three instance variables. The first, self.n, simply holds the integer value “n” provided as a parameter. The second, self.board, should be a new instance of the GameBoard class (described below), built using the provided height and width as dimensions. The third, self.players, should be an initially empty list. add_player(self,name,symbol) The add_player method is provided a name string and a symbol string. These two values should be…arrow_forwardWrite a class named IceCreamCup that implements the Customizable interface. The class should have the following private instance variables. Do NOT give any method implementations for this question, but only declare the instance variables. Do NOT initialize the instance variables -- except nCups below. A String variable name describing the ice cream name • A List of Flavor s added to the cup. Name it flist Additionally, define the following static variable: • An int nCups describing the total number of ice cream cups instantiated. Initialize this variablearrow_forward
- Consider the Speaker interface shown below: public interface Speaker{ public void speak();} For this problem, you will create 2 classes Human and Dog, both of which will implement the Speaker interface. The classes will not contain any properties; they will only implement the speak method, as dictated by the Speaker interface. The speak method will simply print a message in the "native language" of that speaker: For a Human, the speak method will print "Hello!" For a Dog, the speak method will print "Bark!"arrow_forwardThe goal of this coding exercise is to create two classes BookstoreBook and LibraryBook. Both classes have these attributes: author: Stringtiltle: Stringisbn : String- The BookstoreBook has an additional data member to store the price of the book, and whether the book is on sale or not. If a bookstore book is on sale, we need to add the reduction percentage (like 20% off...etc). For a LibraryBook, we add the call number (that tells you where the book is in the library) as a string. The call number is automatically generated by the following procedure:The call number is a string with the format xx.yyy.c, where xx is the floor number that is randomly assigned (our library has 99 floors), yyy are the first three letters of the author’s name (we assume that all names are at least three letters long), and c is the last character of the isbn.- In each of the classes, add the setters, the getters, at least three constructors (of your choosing) and override the toString method (see samplerun…arrow_forwardGoal 1: Update the Fractions Class Here we will overload two functions that will used by the Recipe class later: multipliedBy, dividedBy Previously, these functions took a Fraction object as a parameter. Now ADD an implementation that takes an integer as a parameter. The functionality remains the same: Instead of multiplying/dividing the current object with another Fraction, it multiplies/divides with an integer (affecting numerator/denominator of the returned object). For example; a fraction 2/3 when multiplied by 4 becomes 8/3. Similarly, a fraction 2/3 when divided by 4 becomes 1/6. Goal 2: Update the Recipe Class The Recipe constructors so far did not specify how many servings the recipe is for. We will now add a private member variable of type int to denote the serving size and initialize it to 1. This means all recipes are initially constructed for a single serving. Update the overloaded extraction operator (<<) to include the serving size (see sample output below for an…arrow_forward
- You are given a template to help you follow the required structure. There are 10 files for the base and derived classes with specification and implementation files You are also given a driver MagicalCreaturesMain.cpp that you will complete. So 11 files in all. MagicalCreatureHeader.h // given to you MagicalCreature.cpp // given to you ElfHeader.h // given to you Elf.cpp // you implement methods DragonHeader.h // given to you Dragon.cpp // you implement methods GoblinHeader.h // given to you Goblin.cpp // you implement methods GenieHeader.h // given to you Genie.cpp // you implement methods Specifications: Here are five UML Diagrams: One for the Base class and the others for the derived classes. Use these along with the given template to complete the design of the classes and create the application. The template contains comments and notation as to where you need to fill in the code;…arrow_forwardIn C++ Create a new project named lab8_1. You will be implementing two classes: A Book class, and a Bookshelf class. The Bookshelf has a Book object (actually 3 of them). You will be able to choose what Book to place in each Bookshelf. Here are their UML diagrams Book class UML Book - author : string- title : string- id : int- count : static int + Book()+ Book(string, string)+ setAuthor(string) : void+ setTitle(string) : void+ print() : void+ setID() : void And the Bookshelf class UML Bookshelf - book1 : Book- book2 : Book- book3 : Book + Bookshelf()+ Bookshelf(Book, Book, Book)+ setBook1(Book) : void+ setBook2(Book) : void+ setBook3(Book) : void+ print() : void Some additional information: The Book class also has two constructors, which again offer the option of declaring a Book object with an author and title, or using the default constructor to set the author and title later, via the setters . The Book class will have a static member variable…arrow_forwardGoal 1: Update the Fractions Class Here we will overload two functions that will used by the Recipe class later: multipliedBy, dividedBy Previously, these functions took a Fraction object as a parameter. Now ADD an implementation that takes an integer as a parameter. The functionality remains the same: Instead of multiplying/dividing the current object with another Fraction, it multiplies/divides with an integer (affecting numerator/denominator of the returned object). For example; a fraction 2/3 when multiplied by 4 becomes 8/3. Similarly, a fraction 2/3 when divided by 4 becomes 1/6. Goal 2: Update the Recipe Class Now add a private member variable of type int to denote the serving size and initialize it to 1. This means all recipes are initially constructed for a single serving. Update the overloaded extraction operator (<<) to include the serving size (sample output below for an example of formatting) . New Member functions to the Recipe Class: 1. Add four member functions…arrow_forward
- Code: import java.util.*; //Bicycle interface interface Bicycle { abstract void changeCadence(int newValue); //will change value of candence to new value abstract void changeGear (int newValue); //changes gear of car abstract void speedUp(int increment); //increments speed of car by adding new Value to existing speed abstract void applyBrakes(int decrement); } //ACMEBicycle class definition class ACMEBicycle implements Bicycle { int cadence = 0; Â Â int speed = 0; Â Â int gear = 1; //methods of interface public void changeCadence(int newValue) { this.cadence=newValue; } public void changeGear (int newValue) { this.gear=newValue; } public void speedUp(int increment) { this.speed+=increment; } public void applyBrakes(int decrement) { this.speed-=decrement; } //display method void display() { System.out.println("Cadence: "+this.cadence); System.out.println("Gear: "+this.gear); System.out.println("Speed: "+this.speed); } } //KEYOBicycle class definition class KEYOBicycle implements…arrow_forwardThe total cost of a group of items at a grocery store is based on the sum of the individual product prices and the tax (which is 5.75%). Products that are considered “necessities” are not taxed, whereas products that are considered “luxuries” are. The Product class is abstract, and it has a method called getTotalPrice. Your task is to create two subclasses of Product: NecessaryProduct and LuxuryProduct and implement the getTotalPrice method in each of these classes appropriately. Then modify the driver program to instantiate four products (two necessary and two luxury) and store them in the product array, print out each item in the array, and display the total cost of the items. You should not make any changes at all to Product.java, and you should only add to ShoppingTripStartingCode.java. Do not change any code that is already present Example(Cheese and bread are necessities and soda and candy are luxuries)Cheese…arrow_forwardCan you please help me with this, its in java. Thank you. Write classes in an inheritance hierarchy Implement a polymorphic method Create an ArrayList object Use ArrayList methods For this, please design and write a Java program to keep track of various menu items. Your program will store, display and modify salads, sandwiches and frozen yogurts. Present the user with the following menu options: Actions: 1) Add a salad2) Add a sandwich3) Add a frozen yogurt4) Display an item5) Display all items6) Add a topping to an item9) QuitIf the user does not enter one of these options, then display:Sorry, <NUMBER> is not a valid option.Where <NUMBER> is the user's input. All menu items have the following: Name (String) Price (double) Topping (StringBuilder or StringBuffer of comma separated values) In addition to everything that a menu item has, a main dish (salad or sandwich) also has: Side (String) A salad also has: Dressing (String) A sandwich also has: Bread type…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
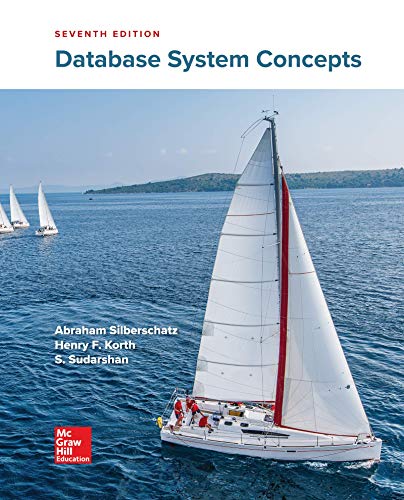
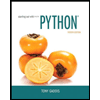
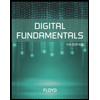
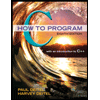
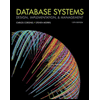
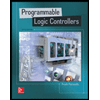