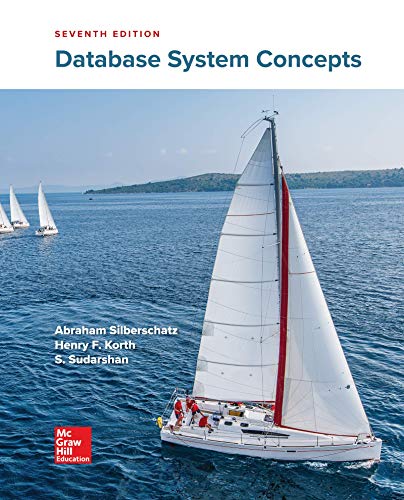
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Topic Video
Question
Code a C++ program , named processes.cpp that receives one argument, (i.e., argv[1]) upon its
invocation and searches how many processes whose name is given in argv[1] are running on
the system where your program has been invoked. To be specific, your program should
demonstrate the same behavior as:
$ ps -A | grep argv[1] | wc -l
Implement processes using the following system calls:
1. pid_t fork( void ); creates a child process that differs from the parent process only in
terms of their process IDs.
2. int execlp( const char *file, const char *arg, ..., (char *)0 ); replaces the current
process image with a new process image that will be loaded from file. The first
argument arg must be the same as file. The last argument is a NULL pointer of type
“char *”.
3. int pipe( int filedes[2] ); creates a pair of file descriptors (which point to a pipe
structure), and places them in the array pointed to by filedes. filedes[0] is for reading
data from the pipe, filedes[1] is for writing data to the pipe.
4. int dup2( int oldfd, int newfd ); creates in newfd a copy of the file descriptor oldfd.
5. pid_t wait( int *status ); waits for process termination
6. int close( int fd ); closes a file descriptor.
For more details, type the man command from the shell prompt line. Use only the system calls
listed above. Do not use the system system call. Imitate how the shell performs "ps -A | grep
argv[1] | wc -l". In other words, your parent process spawns a child that spawns a grand-child
that spawns a great-grand-child. Each process should execute a different command as follows:
Process Command Stdin Stdout
Parent
wait for a
child no change no change
Child wc -l redirected from a grand-child's stdout no change
Grand-child grep argv[1]
redirected from a great-grand-child's
stdout redirected to a child's stdin
Great-grand-
child ps -A no change
redirected to a grand-child's
stdin
4. What to Turn In On Canvas
Program 1 must include:
1. Softcopy:
processes.cpp file
2. A sample of output when running your program vs. actual system calls (to be
included in 3. Report):
invocation and searches how many processes whose name is given in argv[1] are running on
the system where your program has been invoked. To be specific, your program should
demonstrate the same behavior as:
$ ps -A | grep argv[1] | wc -l
Implement processes using the following system calls:
1. pid_t fork( void ); creates a child process that differs from the parent process only in
terms of their process IDs.
2. int execlp( const char *file, const char *arg, ..., (char *)0 ); replaces the current
process image with a new process image that will be loaded from file. The first
argument arg must be the same as file. The last argument is a NULL pointer of type
“char *”.
3. int pipe( int filedes[2] ); creates a pair of file descriptors (which point to a pipe
structure), and places them in the array pointed to by filedes. filedes[0] is for reading
data from the pipe, filedes[1] is for writing data to the pipe.
4. int dup2( int oldfd, int newfd ); creates in newfd a copy of the file descriptor oldfd.
5. pid_t wait( int *status ); waits for process termination
6. int close( int fd ); closes a file descriptor.
For more details, type the man command from the shell prompt line. Use only the system calls
listed above. Do not use the system system call. Imitate how the shell performs "ps -A | grep
argv[1] | wc -l". In other words, your parent process spawns a child that spawns a grand-child
that spawns a great-grand-child. Each process should execute a different command as follows:
Process Command Stdin Stdout
Parent
wait for a
child no change no change
Child wc -l redirected from a grand-child's stdout no change
Grand-child grep argv[1]
redirected from a great-grand-child's
stdout redirected to a child's stdin
Great-grand-
child ps -A no change
redirected to a grand-child's
stdin
4. What to Turn In On Canvas
Program 1 must include:
1. Softcopy:
processes.cpp file
2. A sample of output when running your program vs. actual system calls (to be
included in 3. Report):
$ ./processes kworker
$ ps -A | grep kworker | wc -l
$ ./processes sshd
$ ps -A | grep sshd | wc -l
$ ./processes scsi
$ ps -A | grep scsi | wc -l
$ ps -A | grep kworker | wc -l
$ ./processes sshd
$ ps -A | grep sshd | wc -l
$ ./processes scsi
$ ps -A | grep scsi | wc -l
SAVE
AI-Generated Solution
info
AI-generated content may present inaccurate or offensive content that does not represent bartleby’s views.
Unlock instant AI solutions
Tap the button
to generate a solution
to generate a solution
Click the button to generate
a solution
a solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- GDB and Getopt Class Activity activity [-r] -b bval value Required Modify the activity program from last week with the usage shown. The value for bval is required to be an integer as is the value at the end. In addition, there should only be one value. Since everything on the command line is read in as a string, these now need to be converted to numbers. You can use the function atoi() to do that conversion. You can do the conversion in the switch or you can do it at the end of the program. The number coming in as bval and the value should be added together to get a total. That should be the only value printed out at the end. Total = x should be the only output from the program upon success. Remove all the other print statements after testing is complete. Take a screenshot of the output to paste into a Word document and submit. Practice Compile the program with the -g option to load the symbol table. Run the program using gdb and use watch on the result so the program stops when the…arrow_forwardPlease write in C++ and run in linux. This assignment is about fork(), exec(), and wait() system calls, and commandline arguments. Write two C++ programs, to be named parent.cc and child.cc and compiled into executable parent and child, respectively that, when run, will work as follows: parent takes in a list of gender-name pairs from the commandline arguments creates as many child processes as there are in the gender-name pairs and passes to each child process a child number and a gender-name pair waits for all child processes to terminate outputs “All child processes terminated. Parent exits.” And terminates. child receives a child number and one gender-name pair arguments from parent outputs “Child # x, a boy (or girl), name xxxxxx.” Note: content of output depends on data received from parent Sample run To invoke the execution: >parent girl Nancy boy Mark boy Joseph parent process does the following: outputs “I have 3 children.” -- Note: the number 3…arrow_forwardPlease write this C++ program using vector of objects as per the following instructions ( read carefully ). Explain all your steps as if any beginner could understand like how many cpp files or text files should be there. Are the 2 txt files w old and new ta indie or only 1? this task needs to be done with vector of TAs object. Please explain how does it work as I don't know anything about it. Any explanation and comment is very needed . please don't copy from any other sources .please write on your own or skip. PLEASE ATTACH PICTURES w te written code and show the output too Thank you so much for your time and helparrow_forward
- This is my main.cpp, if there's anything wrong with it in relation to the assignment, please point it out and how to solve it. I can't get my functions.cpp to connect properly to the main.cpp. I need the functions.cpp first, anything else comes second. Thank you very much. main.cpp: #include <iostream> #include <fstream> #include <iomanip> #include <string> #include "functions.cpp" using namespace std; //struct for menuItemType struct menuItemType { string menuItem; double menuPrice; } void getData(menuItemType menuList[]); void showMenu(menuItemType menuList[], int x); void printCheck(menuItemType menuList[], int menuOrder[], int x); int main(); { // Declare functions and variables const int menuItems = 8; menuItemType menuList[menuItems]; int menuOrder[menuItems] = {0}; int orderChoice = 0; bool ordering = true; int count = 0; // Set the menu getData(menuList);…arrow_forwardWrite a C program to keep records and perform statistical analysis for a class of n students. The information of each student contains Name, CAT-1 score, CAT-2 score and total score. The program will prompt the user to choose the operation of records from a menu as shown below1. Add student records 2. Delete student records 3. Update student records 4. View all student records Implement a Menu Driven Program for the above specification using functions only.arrow_forwardOperating System What is the value of argc and argv(1) with an invocation of a C program like: copyfile text1 text2 a. argc=b. argv(1) =arrow_forward
- Language is c++arrow_forward1. Given a global list of users and password like the following, write a function (called login) that takes a user name and password and logs in the user if login is successful:- the user exists in the list- and the password is correct The function should return a True/False users = {'Jane': 'Password123@', 'John': 'Password123!'} 2. Write a function called register that takes a user name and password and adds the user to the list if the user does not already exist in the listThe function should return a True/False Put it all together: 3. Write a showmenu function that shows the following options and reads the choice from user and returns it: 1: register 2: login 0: logout 3. In the main section, based on the user's selected option, ask the required input (user name, passwords) and perform the requested operation. 4. Repeat the above until the user enters 0. (keep showing the menu that asks for another option)arrow_forwardIn c++, please. Thank you! Given a main() that reads user IDs (until -1), complete the BubbleSort() functions to sort the IDs in ascending order using the Bubblesort algorithm, and output the sorted IDs one per line. You may assume there will be no more than 100 user IDs. Ex. If the input is: kaylasimms julia myron1994 kaylajones -1 the output is: julia kaylajones kaylasimms myron1994 The following code is given: #include <string>#include <iostream> using namespace std; // TODO: Write the Bubblesort algorithm that sorts the array of string, with k elementsvoid Bubblesort(string userIDs [ ], int k) { } int main() { string userIDList[100]; string userID; cin >> userID; while (userID != "-1") { //put userID in the array cin >> userID; } // Initial call to quicksort Bubblesort(userIDList, /* ?? */ ); //make this output only the userIDs that were entered, not garbage for (int i = 0; i < 100; ++i) { cout <<…arrow_forward
- C++ LANGUAGE In this assignment, you will be writing two objects to heuristically compare the performance of the bubble sort, selection sort, and insertion sort algorithms. To do this, a “Timer” object will be created and compositionally related to a storage class that will load the file contents, execute the sorts in an unbiased manner, handle any memory allocations that need to be made in order to do this, and reports back the execution times of each algorithm on the provided input files for that machine. First you will need to make a Timer object. This object should function like a traditional stopwatch with methods for starting, stopping, resetting, and reporting back times. The design of the object itself is up to you (it should minimally contain methods for the aforementioned ideas), but it must consist of a solitary object that provides interfaces appropriate for being compositionally included as part of a sorting object to facilitate the timekeeping portion of this exercise.…arrow_forwardUse the started code provided with QUEUE Container Adapter methods and provide the implementation of a requested functionality outlined below. This program has to be in c++, and have to use the already started code below. Scenario: A local restaurant has hired you to develop an application that will manage customer orders. Each order will be put in the queue and will be called on a first come first served bases. Develop the menu driven application with the following menu items: Add order Next order Previous order Delete order Order Size View order list View current order Order management will be resolved by utilization of an STL-queue container’s functionalities and use of the following Queue container adapter functions: enQueue: Adds the order in the queue DeQueue: Deletes the order from the queue Peek: Returns the order that is top in the queue without removing it IsEmpty: checks do we have any orders in the queue Size: returns the number of orders that are in the queue…arrow_forwardwrite the code in C++arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
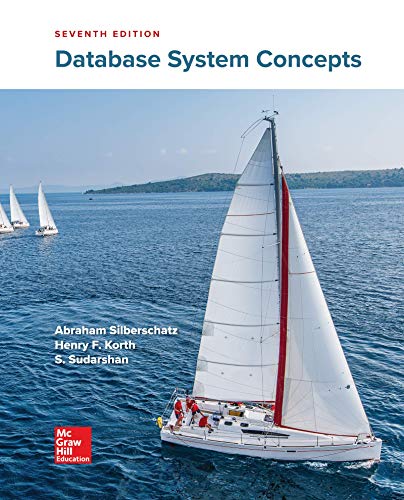
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
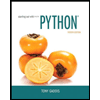
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
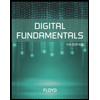
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
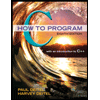
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
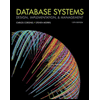
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
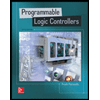
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education