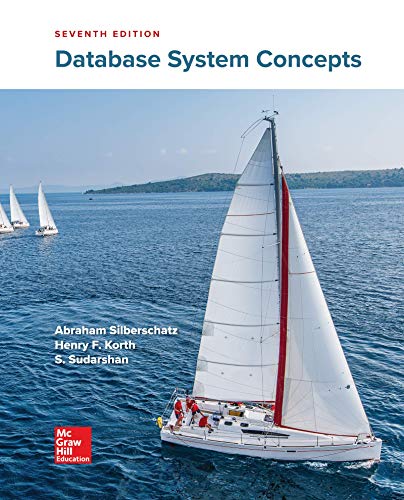
Concept explainers
Use the started code provided with QUEUE Container Adapter methods and provide the implementation of a requested functionality outlined below.
This program has to be in c++, and have to use the already started code below.
Scenario:
A local restaurant has hired you to develop an application that will manage customer orders. Each order will be put in the queue and will be called on a first come first served bases.
Develop the menu driven application with the following menu items:
- Add order
- Next order
- Previous order
- Delete order
- Order Size
- View order list
- View current order
Order management will be resolved by utilization of an STL-queue container’s functionalities and use of the following Queue container adapter functions:
- enQueue: Adds the order in the queue
- DeQueue: Deletes the order from the queue
- Peek: Returns the order that is top in the queue without removing it
- IsEmpty: checks do we have any orders in the queue
- Size: returns the number of orders that are in the queue
While adding a new order in the queue, the program will be capable of collecting the following order information:
- Name on order
- Order description
- Order total
- Order tip
- Date of order
The started code that you have to use:
#include <iostream>
#include <queue>
#include <string>
using namespace std;
class patient {
/ /Creates class for patient variable
public:
string firstName;
string insuranceType;
string lastName;
string ssn;
string address;
string visitDate;
};
queue <patient> p;
void enqueue()
//Enqueues patient details from console input
{
patient pa;
cout << "Please enter Patient First Name:";
cin >> pa.firstName;
cout << "Please enter Patient Last Name:";
cin >> pa.lastName;
cout << "Please enter Patient insurance type:";
cin >> pa.insuranceType;
cout << "Please enter Patient SSN:";
cin >> pa.ssn;
cout << "Please enter Patient Address:";
cin.ignore();
getline(cin, pa.address);
cout << "Please enter Patient Date of Visit:";
cin >> pa.visitDate; p.push(pa);
}
patient dequeue()
//Removes patient from queue
{
patient pa;
if (!p.empty()) {
pa = p.front();
p.pop();
}
return pa;
}
void peek()
//Returns current patient at the front of the queue
{
if (!p.empty()) {
patient tmp = p.front();
cout << "Patient Name is " << tmp.firstName<< endl;
}
}
int main() {
int x = 0;
int n;
patient last;
while (x == 0) {
//Prompts for Menu Selection while selection = 0, which is defaulted to 0
cout << "Welcome to Dental Associates of Kansas City" << endl; cout << "**************************************************" << endl;
cout << "To get started, please select an option from the menu below:" << endl;
cout << "1. Add patient" << endl;
cout << "2. Next patient" << endl;
cout << "3. Previous patient" << endl;
cout << "4. Delete patient" << endl;
cout << "5. View current" << endl;
cout << "6. Exit this program" << endl; cout << "Enter the number of the action you would like to perform: ";
cin >> n; if (n == 1) {
enqueue();
} else if (n == 2) {
last = dequeue();
peek();
} else if (n == 3) {
cout << last.firstName << endl;
} else if (n == 4) {
last = dequeue();
} else if (n == 5) {
peek();
} else if (n == 6) {
exit(0);
}
}
}
Please make sure the user can view the list , view the size, add order, etc. as the commands say.

Step by stepSolved in 4 steps with 3 images

- You are to use the started code provided with QUEUE Container Adapter methods and provide the implementation of a requested functionality outlined below. The program has to be in c++, and have to use the already started code below. Please make sure the code is able to add order, next order, previous order, delete order, order size, view order list, view current order, and exit program. Scenario: A local restaurant has hired you to develop an application that will manage customer orders. Each order will be put in the queue and will be called on a first come first served bases. Develop the menu driven application with the following menu items: Add order Next order Previous order Delete order Order Size View order list View current order Order management will be resolved by utilization of an STL-queue container’s functionalities and use of the following Queue container adapter functions: enQueue: Adds the order in the queue DeQueue: Deletes the order from the queue Peek:…arrow_forwardYou are working with a team creating a grocery shopping app. The app keeps the information of the items to buy in the list grocery list, and the items that were purchased in purchased list. You need to create a function that returns the items that were purchased, but that were not in the grocery list. For example, if the grocery list is milk, eggs, bacon, and flour; and we purchased milk, chocolate, and muffins, the function should return a list with chocolate and muffins. Drag and drop the expressions needed to implement the required function. def get_extra_items(grocery_list, purchased_list): list3 [] for i in range( ) : if not in list3.append( return list3 len(grocery_list) grocery_list grocery_list[i] purchased_list[i] purchased_list len(purchased_list)arrow_forwardA for construct is used in programming to build a loop that processes a list of things. In order to achieve this, it runs endlessly so long as there are things to process. Is this statement true or false? explain yourself.arrow_forward
- Need help with this Java review If you can also send a screenshot will be helpful to understan Objective: The purpose of this lab exercise is to create a Singly Linked List data structure . Please note that the underlying implementation should follow with the descriptions listed below. Instructions : Create the following Linked List Data Structure in your single package and use "for loops" for your repetitive tasks. Task Check List ONLY "for" loops should be used within the data structure class. Names of identifiers MUST match the names listed in the description below. Deductions otherwise. Description The internal structure of this Linked List is a singly linked Node data structure and should have at a minimum the following specifications: data fields: The data fields to declare are private and you will keep track of the size of the list with the variable size and the start of the list with the reference variable data. first is a reference variable for the first Node in…arrow_forwardIn C++ language. Please look at the instructions and help with program. This is for intermediate not advanced. so help me understand pleasearrow_forwardYou are writing an application for the 911 dispacher office for a city. You need a data structure that will allow you to store all the residents' phone numbers and addresses. The common operations performed on the set of objects are: lookup an address based on the calling phone number. The client wants the program to be as efficient as possible. Which of the following data structures should you use in your design? Question 43 options: Hash table Binary search tree Heap Sorted linked Listarrow_forward
- For this assignment, you are to use the started code provided with QUEUE Container Adapter methods and provide the implementation of a requested functionality outlined below. Scenario: A local restaurant has hired you to develop an application that will manage customer orders. Each order will be put in the queue and will be called on a first come first served bases. Develop the menu driven application with the following menu items: Add order Next order Previous order Delete order Order Size View order list View current order Order management will be resolved by utilization of an STL-queue container’s functionalities and use of the following Queue container adapter functions: enQueue: Adds the order in the queue DeQueue: Deletes the order from the queue Peek: Returns the order that is top in the queue without removing it IsEmpty: checks do we have any orders in the queue Size: returns the number of orders that are in the queue While adding a new order in the queue, the program…arrow_forwardwrite a program for a library automation which gets the isbn number, name, author and publication year of the books in the library. the status will be filled by the program as follows: if publication year before 1985 the status is reference else status is available, the information about the books should be stored inside a linked list. the program should have a menu and the user inserts, displays, and deletes the elements from the menu by write a selecting options. the following data structure should be used. struct list char isbn[ 20 ]: char namei 20 ]. char authori 20 ]: int year; char status[200j: struct list "next, jinfo, wedoad n un posn ag pinogs nuu sulnogoj l press 1. to insert a book press 2. to display the book list press 3. to delete a book from listarrow_forwardYou are going to write a program (In Python) called BankApp to simulate a banking application.The information needed for this project are stored in a text file. Those are:usernames, passwords, and balances.Your program should read username, passwords, and balances for each customer, andstore them into three lists.userName (string), passWord(string), balances(float)The txt file with information is provided as UserInformtion.txtExample: This will demonstrate if file only contains information of 3 customers. Youcould add more users into the file.userName passWord Balance========================Mike sorat1237# 350Jane para432@4 400Steve asora8731% 500 When a user runs your program, it should ask for the username and passwordfirst. Check if the user matches a customer in the bank with the informationprovided. Remember username and password should be case sensitive.After asking for the user name, and password display a menu with the…arrow_forward
- You are to use the started code provided with QUEUE Container Adapter methods and provide the implementation of a requested functionality outlined below. The program has to be in c++, and have to use the already started code below. Scenario: A local restaurant has hired you to develop an application that will manage customer orders. Each order will be put in the queue and will be called on a first come first served bases. Develop the menu driven application with the following menu items: Add order Next order Previous order Delete order Order Size View order list View current order Order management will be resolved by utilization of an STL-queue container’s functionalities and use of the following Queue container adapter functions: enQueue: Adds the order in the queue DeQueue: Deletes the order from the queue Peek: Returns the order that is top in the queue without removing it IsEmpty: checks do we have any orders in the queue Size: returns the number of orders that are in…arrow_forwardIn C++,arrow_forwardYou will create two programs. The first one will use the data structure Stack and the other program will use the data structure Queue. Keep in mind that you should already know from your video and free textbook that Java uses a LinkedList integration for Queue. Stack Program Create a deck of cards using an array (Array size 15). Each card is an object. So you will have to create a Card class that has a value (1 - 10, Jack, Queen, King, Ace) and suit (clubs, diamonds, heart, spade). You will create a stack and randomly pick a card from the deck to put be pushed onto the stack. You will repeat this 5 times. Then you will take cards off the top of the stack (pop) and reveal the values of the cards in the output. As a challenge, you may have the user guess the value and suit of the card at the bottom of the stack. Queue Program There is a new concert coming to town. This concert is popular and has a long line. The line uses the data structure Queue. The people in the line are objects…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
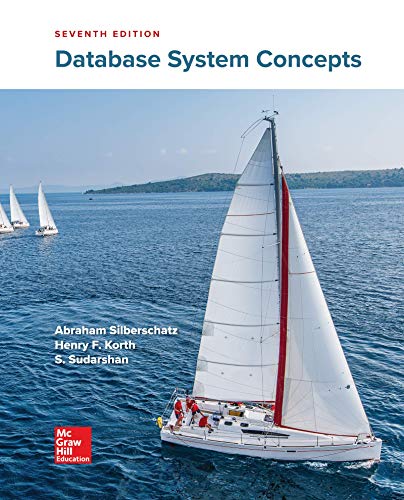
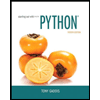
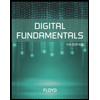
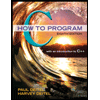
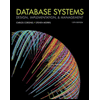
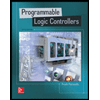