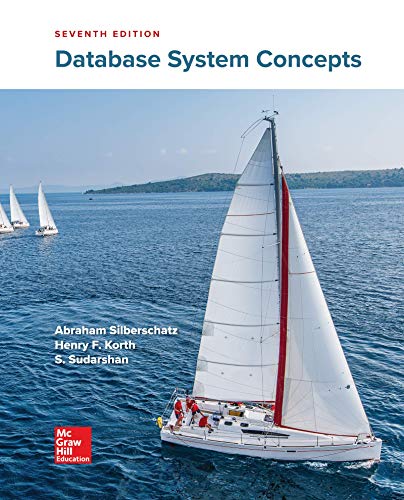
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
public class test{
private static final int MAX = 100;
private int[] sco;
public test(int[] sco){
this.sco = sco;
}
private int comAvg() throws IllegalArgumentException{
int sum = 0;
for (int i = 0; i < sco.length; i++){
int sc = sco[i];
if (sc < 0 || sc > MAX){
throw new IllegalArgumentException("Score (" + sc + ") is not in the range 0-" + MAX);
}
sum += sco[i];
}
int av = sum / sco.length;
return av;
}
public static void main(String[] args){
test test = new test(new int[] { 50, 70, 81 });
try{
int avgSc = test.comAvg();
char letG;
if (avgSc < 60) letG = 'F';
else if (avgSc < 70) letG = 'D';
else if (avgSc < 80) letG = 'C';
else if (avgSc < 90) letG = 'B';
else letG = 'A';
System.out.println("\nAVG Score is " + avgSc + " and Grade is an " +
letG);
}
catch (IllegalArgumentException illegalArgumentException){
System.out.println(illegalArgumentException.getMessage());
}
}
}

Transcribed Image Text:2. TestScores Class Custom Exception
Write an exception class named InvalidTestScore. Modify the TestScores class you
wrote in Programming Challenge 1 so that it throws an InvalidTestScore exception if any
of the test scores in the array are invalid.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- public String getJoblitle() { return joblitle; 11 12 13 44 45 46 47 48 49 50 public void setJoblitle(String joblitle) { this.jobTitle - joblitle; public int getCurrentEmploynent() { return currentEmploynent; 1. public void selCurrentEnployent (int current Fmployment) { this.currentEmploynent - currentEmployment; 53 54 55 56 -57 goverride public String tostring () { return "CurrentEmploynentStatsclass [areaName=" + arealame + 58 + "year=" + year + ", jobtitle-" + joblitle , currentEnploynent-" + currenttmployment + "]": 59 61 62 63 64 } What algorithm does this method perform? public statie Currentiploynentstatsclass mysteryethod (CurrentEmploynentstatsclass] array) ( int holder = array[0).getCurrenttmploynent(); Currenttmploynentstatsclass variable = array[®]; for(int i- 0, i holder) { holder = holderx; variable - array[1]; return variable; 36. What is the above method returning? 37. What is the Big O time of the above method?arrow_forwardint sum = 0; for (int i 0; i < 5; i++){ sum += i; } cout << sum;arrow_forwardPROBLEM STATEMENT: An anagram is a word that has been rearranged from another word, check tosee if the second word is a rearrangement of the first word. public class AnagramComputation{public static boolean solution(String word1, String word2){// ↓↓↓↓ your code goes here ↓↓↓↓return true;}} Can you help me with this java question the language is java please use the code I gavearrow_forward
- public class SeperateDuplicates { public static void main(String[ args) { System.out.printIn(seperateDuplicatesChars("Hello")); System.out.printin(seperateDuplicatesChars ("Bookkeeper"')); System.out.printin(seperateDuplicatesChars("Yellowwood door"')); System.out.printin(seperateDuplicatesChars("Chicago Cubs")); */ public static String seperateDuplicatesChars(String str) { //To be completed } }arrow_forwardLab 9 C balance the same, y, n, why? class CheckingAct { . . . . private int balance; public void processCheck( int amount ) { int charge; if ( balance < 100000 ) charge = 15; else charge = 0; balance = balance - amount - charge ; // change the local copy of the value in "amount" amount = 0 ; } } public class CheckingTester { public static void main ( String[] args ) { CheckingAct act; int check = 5000; act = new CheckingAct( "123-345-99", "Your Name", 100000 ); System.out.println( "check:" + check ); // prints "5000" // call processCheck with a copy of the value 5000 act.processCheck( check ); System.out.println( "check:" + check ); // prints "5000" --- "check" was not changed } }arrow_forward1 a. is the amount the same, yes or no, why? public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { balance = balance + amount ; } // modified toString() method public String toString() { return "Account: " + actNum + "\tName: " + nameOnAct + "\tBalance: " + amount ; } } b. public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { // scope of amount starts here balance = balance + amount ; // scope of amount ends here } public void processCheck( int amount ) { // scope of amount starts here int charge; incrementUse(); if ( balance < 100000 ) charge = 15; else charge = 0; balance = balance - amount - charge ; // scope of amount ends here } } c. is the…arrow_forward
- Code: import java.util.Scanner;public class ReceiptMaker { public static final String SENTINEL = "checkout";public final int MAX_NUM_ITEMS;public final int TAX_RATE;private String itemNames;private double [] itemPrices;private int numItemsPurchased; public ReceiptMaker(){MAX_NUM_ITEMS = 10;TAX_RATE = .0875; itemNames = new String[MAX_NUM_ITEMS];itemPrices = new double[MIN_NUM_ITEMS];numItemsPurchased = 0;}public ReceiptMaker(int maxNumItems, double taxRate){if(isValid(maxNumItems)){MAX_NUM_ITEMS = maxNumItems;}else{MAX_NUM_ITEMS= 10;}if(isValid(taxRate)){TAX_RATE = taxRate;}else{TAX_RATE = .0875;}itemNames = new String[MAX_NUM_ITEMS];itemPrices = new double[MAX_NUM_ITEMS];numItemsPurchased = 0;}public void greetUser(){System.out.println("Welcome to the "+MAX_NUM_ITEMS+" items or less checkout line");}public void promptUserForProductEntry(){System.out.println("Enter item #"+(numItemsPurchased+1)+"'s name and price separated by a space, or enter ""+SENTINEL+"\" to end transaction…arrow_forwardStatement that increases numPeople by 5. Ex: If numPeople is initially 10, the output is: There are 15 people.arrow_forwardJavaarrow_forward
- public class arrayOutput { public static void main (String [] args) { final int NUM_ELEMENTS = 3; int[] userVals = new int [NUM_ELEMENTS]; int i; int sumVal; userVals [0] = 3; userVals [1] = 5; userVals [2] = 8; Type the program's output sumVal = 0; for (i = 0; i < userVals.length; ++i) { sumVal= sumVal + userVals [i]; System.out.println (sumVal); } ? ? ??arrow_forwardpublic class KnowledgeCheckTrek { public static final String TNG = "The Next Generation"; public static final String DS9 = "Deep Space Nine"; public static final String VOYAGER = "Voyager"; public static String trek(String character) { if (character != null && (character.equalsIgnoreCase("Picard") || character.equalsIgnoreCase("Data"))) { // IF ONE return TNG; } else if (character != null && character.contains("7")) { // IF TWO return VOYAGER; } else if ((character.contains("Quark") || character.contains("Odo"))) { // IF THREE return DS9; } return null; } public static void main(String[] args) { System.out.println(trek("Captain Picard")); System.out.println(trek("7 of Nine")); System.out.println(trek("Odo")); System.out.println(trek("Quark")); System.out.println(trek("Data").equalsIgnoreCase(TNG));…arrow_forwardusing System; class Program { publicstaticvoid Main(string[] args) { int number = 1; while (number <= 88) { Console.WriteLine(number); number = number + 2; } int[] randNo = newint[88]; Random r = new Random(); int i=0; while (number <= 88) { randNo[i] = number; number+=1; i+=1; } for (i = 0; i < 3; i++) { Console.WriteLine("Random Numbers between 1 and 88 are " + randNo[r.Next(1, 88)]); } } } this code counts from 1-88 in odds and then selects three different random numbers. it keeps choosing 0 as a random number everytime. how can that be fixed?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
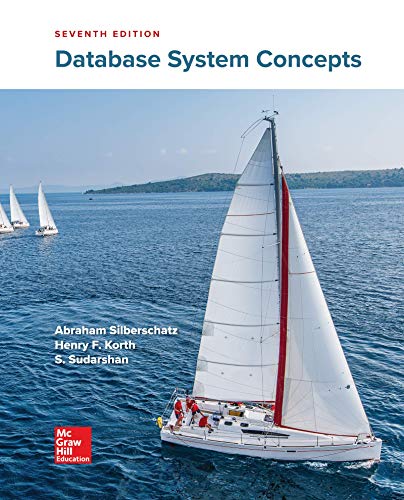
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
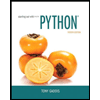
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
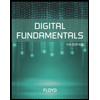
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
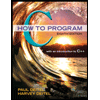
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
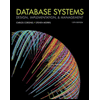
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
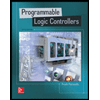
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education