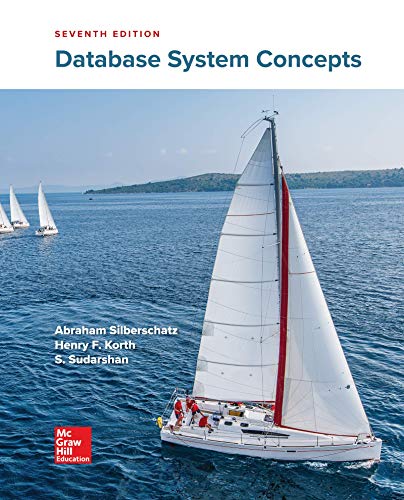
Given hash_function( ) defined in the default template, complete the main function that does the following tasks:
- Create a list called hash_list that contains the five hashing
algorithm names described above. - Read from the user a password to hash.
- Declare a salt variable and initialize the variable to the hex representation of 4458585599393. Hint: Use function hex().
- Use a for loop to iterate over the hash_list and call the hash_function() with the hashing algorithm names in the list. Store the returned value of hash_function() in a variable and output the algorithm name used and the hashed password. Note: Output a new line after each hashed password is printed.
If the input is: secretPass
the output is:
Testing hash algorithm: md5 Hashed Password = bd19f99253c948637d64a4acbd524047:0x40e18692da1
Testing hash algorithm: sha1 Hashed Password = e5fbad38af8ba59c2648e98b9ae4196dfcb9f719:0x40e18692da1
My code is:
import hashlib
def hash_function(password, salt, al):
if al == 'md5':
#md5
hash = hashlib.md5(salt.encode() + password.encode())
hash.update(password.encode('utf-8'))
return hash.hexdigest() + ':' + salt
elif (al == 'sha1'):
#sha1
hash = hashlib.sha1()
hash.update(password.encode('utf-8'))
return hash.hexdigest() + ':' + salt
elif al == 'sha224':
#sha224
hash = hashlib.sha224()
hash.update(password.encode('utf-8'))
return hash.hexdigest() + ':' + salt
elif al == 'sha256':
#sha256
hash = hashlib.sha256()
hash.update(password.encode('utf-8'))
return hash.hexdigest() + ':' + salt
elif al == 'blake2b':
#blake2b512
hash = hashlib.blake2b()
hash.update(password.encode('utf-8'))
return hash.hexdigest() + ':' + salt
else:
print("Error: No Algorithm!")
#return a null string because no encoding is done
return ""
if __name__ == "__main__":
hash_list = ['md5', 'shal', 'sha224', 'sha256', 'blake2b']
password = str(input())
salt = hex(78246391246824)
for i in range (len(hash_list)):
print("Testing hash algorithm: " + hash_list[i])
temp=hash_function(password, salt, hash_list[i])
if temp!="":
# to accomadate the case of no algorithm
print("Hashed Password = " + hash_function(password, salt, hash_list[i]) + "\n")
else:
print("\n")
But I'm not getting the correct output. I don't know what I did wrong.

Step by stepSolved in 3 steps

- data Query = Pipe Query Query | Field String | Elements | Select Query | ConstInt Int | ConstString String | Equal Query Query deriving Show this is a haskell question: Executes a 'Query' by translating it into a `Transformer`. Each of the constructors of 'Uery' is turned into its corresponding 'Transformer' . execute :: Query -> Transformer execute = (answer here) sample output: example: > execute Elements (Array [Number 1, Number 2]) returns: > [Number 1, Number 2] answer in haskell form please.arrow_forwardcreate a function in python parameters: 1. dict[str, list[str]] 2. list[str] return type: dict[str, list[str]] must do like this: 1. establish an empty dictionary that will serve as the dictionary returned at the end 2. loop thorugh each of the columns in the second parameter of the function 2a. asssign to the column key of the result dictionary the list of values stored in the input dictionary at the same column 3. return the dictionary produced do not import other libraries such as pandas, .select, .head, .iloc or anything this function is being used to work in a dataframearrow_forwardQuestion 2 One of the following statement is false about ArrayList class: You can create an ArrayList object of any capacity. You can convert any collection object to ArrayList type. ArrayList has a method and addFirst() to add an element at the beginning. ArrayList implements the Collection Interface.arrow_forward
- def get_task(task_collection, ...): """ param: task_collection (list) - holds all tasks; maps an integer ID/index to each task object (a dictionary) param: ... (str) - a string that is supposed to represent an integer ID that corresponds to a valid index in the collection returns: 0 - if the collection is empty. -1 - if the provided parameter is not a string or if it is not a valid integer Otherwise, convert the provided string to an integer; returns None if the ID is not a valid index in the list or returns the existing item (dict) that was requested. """arrow_forwarddef get_column_sum(index: int, stations: List["Station"]) -> int: """Return the sum of the values at the given index from each inner list of stations. Precondition: the items in stations at the position that index refers to are ints. >>> get_column_sum(BIKES_AVAILABLE, SAMPLE_STATIONS) 23 >>> get_column_sum(DOCKS_AVAILABLE, SAMPLE_STATIONS) 34 """arrow_forwardImplement the following function. Hint: You should use a dictionary. def word_pattern (pattern: str, words: List [str]) -> bool: I ''Given a pattern and a word list, determine if the word list follows the same pattern. That is to say, ensure that pattern [j] pattern [k] only when words [j] == words [k] pattern [j] = pattern [k] means words [j] != words [k] Precondition: Assume pattern is all lowercase letters. >>> word_pattern ('aaaa', ['cat', 'cat', 'cat', 'cat']) True and == >>> word_pattern ('yoox', ['dog', 'cat', 'cat', 'fish']) True >>> word_pattern ('xy', ['cat', 'cat']) False >>> word_pattern('xoox', ['dog', 'cat', 'cat', 'fish']) Falsearrow_forward
- #include <stdio.h>#include <stdlib.h>#include <string.h>#define capacity 101 // Size of Hash Table int hash_function(char* str) { int i = 0; for (int j=0; str[j]; j++) i += str[j]; return i % capacity;} struct Ht_item { char* name; int numberCourses;}; // Hash Table Definitionstruct HashTable { struct Ht_item** items; int size; int count;}; struct Ht_item* create_item(char* key, int value) { struct Ht_item* item = (struct Ht_item*) malloc (sizeof(struct Ht_item)); item->name = (char*) malloc (strlen(key) + 1); item->numberCourses = value; strcpy(item->name, key); item->numberCourses = value; return item;} struct HashTable* create_table(int size) { // Creates a New Hash Table struct HashTable* table = (struct HashTable*) malloc (sizeof(struct HashTable)); table->size = size; table->count = 0; table->items = (struct Ht_item**) calloc (table->size, sizeof(struct Ht_item*));…arrow_forward5. The following function indexCounter aims to find and return all the indices of the element x in a list L. if x is not in the list L, return an empty list. However, the implementation has a flaw, so that many times it will return error message. Can you fix the flaw? def indexCounter(L, x): indexList [] startIndex = 0 while startIndex < len(L): indexList.append (L.index (x, startIndex)) startIndex = L.index (x, startIndex) + 1 return indexListarrow_forwardlinked_list_stack_shopping_list_manager.py This is a file that includes the class of linked list based shopping list manager. This class contains such methods as init, insert_item, is_list_empty, print_item_recursive_from_top, print_items_from_top, print_item_recursive_from_bottom, print_items_from_bottom, getLastItem, removeLastItem. In addition, this class requires the inner class to hold onto data as a linked list based on Stack. DO NOT use standard python library, such as deque from the collections module. Please keep in mind the following notes for each method during implementation: Init(): initializes linked list based on Stack object to be used throughout object life. insert_item(item): inserts item at the front of the linked list based on Stack. Parameters: item name. is_list_empty (): checks if the current Stack object is empty (ex. check if head is None). print_item_recursive_from_top(currentNode): a helper method to print linked list based on Stack item recursively. Note:…arrow_forward
- get_top_regions() that takes three input parameters; the 2-D list (similar to the database), the dictionary that stores information of all regions, and a non-zero positive integer x. This function returns a new dictionary that contains the information of top-x regions in terms of the total number of hospitalization cases recorded in these regions. That means these top-x regions have the largest numbers of hospitalization cases. Each {key:value} pair in this resulting dictionary stores the name of a top region as the key and the value is the total number of hospitalization cases reported in this region. The result does not need to be sorted, the regions of the resulting dictionary can appear in any order as long as the top-x regions have been identified correctly. If the value of x is less than 1, or more than the total number of unique regions, your function should print a message informing the user and return an empty dictionary. See sample outputs below. >>> topx_regions…arrow_forwardA date-to-event dictionary is a dictionary in which the keys are dates (as strings in the format 'YYYY-MM-DD'), and the values are lists of events that take place on that day. Complete the following function according to its docstring. Your code must not mutate the parameter! def group_by_year (events: Dict[str, List[str]]) -> Dict [str, Dict [str, List[str]]]: """Return a dictionary in which in the keys are the years that appear in the dates in events, and the values are the date-to-event dictionaries for all dates in that year. >>> date_to_event = {'2018-01-17': ['meeting', 'lunch'], '2018-03-15': ['lecture'], '2017-05-04': ['gym', 'dinner']} >>> group_by_year (date_to_event) {'2018': {'2018-01-17': ['meeting', 'lunch'], '2018-03-15': ['lecture']}, '2017': {'2017-05-04': ['gym', 'dinner']} } || || ||arrow_forwardIn C++.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
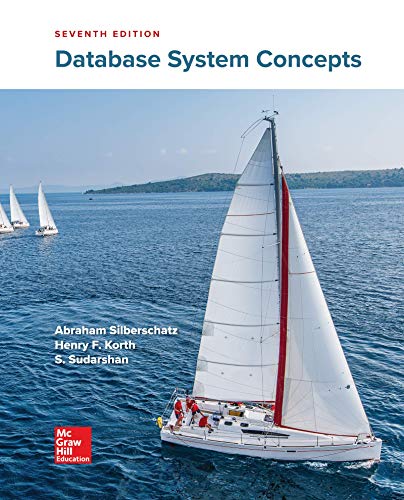
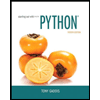
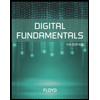
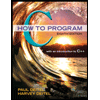
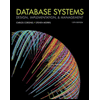
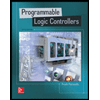