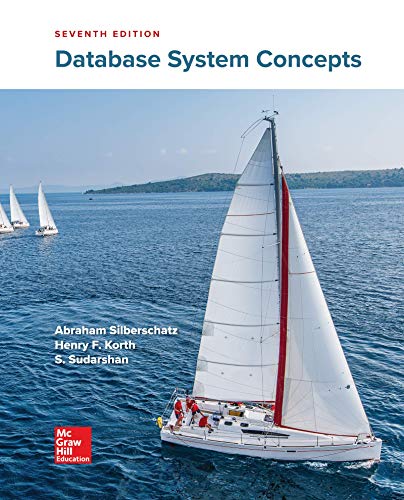
the android code is like this
ublic class PlayerActivity2 extends AppCompatActivity {
ListView simpleList;
String SerialNo[] = {"1", "2", "3", "4", "5", "6", "7", "8", "9", "10"};
int flags[] = {R.drawable.image1, R.drawable.image2, R.drawable.image3, R.drawable.image4, R.drawable.image5, R.drawable.image6, R.drawable.image7, R.drawable.image8, R.drawable.image9, R.drawable.image10};
String Names[] = {"mmm", "nnn", "aaa.", "bbb", "ccc", "ddd", "eee jk", " ijk", "Virgil jk", "gil jklk"};
String Score[] = {"1", "2", "3", "5", "4", "3", "5", "5", "5", "5"};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity2);
simpleList = (ListView) findViewById(R.id.simpleListView);
CustomAdapter customAdapter = new CustomAdapter(getApplicationContext(), simpleList, SerialNo, flags, Names, Score);
simpleList.setAdapter(customAdapter);
simpleList.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> adapterView, View view, int position, long id) {
ImageView imageView = (ImageView) view.findViewById(R.id.imageView);
if (imageView != null) {
saveImage(imageView, Names[position]);
}
}
});
} // add a closing brace here
public void saveImage(View view, String name) {
ImageView imageView = (ImageView) view;
Bitmap bitmap = ((BitmapDrawable) imageView.getDrawable()).getBitmap();
String fileName = name + ".jpg";
File dir = getApplicationContext().getDir("images", Context.MODE_PRIVATE);
File file = new File(dir, fileName);
try {
FileOutputStream fos = new FileOutputStream(file);
bitmap.compress(Bitmap.CompressFormat.JPEG, 100, fos);
fos.flush();
fos.close();
Toast.makeText(getApplicationContext(), "Image saved", Toast.LENGTH_SHORT).show();
} catch (Exception e) {
e.printStackTrace();
}
}
public void showToast(View view) {
Toast.makeText(getApplicationContext(), "Save Image button clicked", Toast.LENGTH_SHORT).show();
}
}
-xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".PlayerActivity2">
<com.google.android.material.appbar.AppBarLayout
android:layout_width="0dp"
android:layout_height="wrap_content"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<androidx.appcompat.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="?attr/colorPrimary"
android:minHeight="?attr/actionBarSize"
android:theme="?attr/actionBarTheme"
tools:layout_editor_absoluteX="0dp"
tools:layout_editor_absoluteY="0dp"
/>
<de.hdodenhof.circleimageview.CircleImageView
android:id="@+id/icon"
android:layout_width="72dp"
android:layout_height="72dp"
android:layout_centerInParent="true"
android:layout_gravity="center"
android:src="@drawable/image1" />
<TextView
android:id="@+id/textNamesView"
android:layout_width="382dp"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="Razi 380"
android:textColor="@color/black" />
</com.google.android.material.appbar.AppBarLayout>
<androidx.constraintlayout.widget.ConstraintLayout
android:id="@+id/linearLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
tools:layout_editor_absoluteX="0dp"
tools:layout_editor_absoluteY="0dp">
<ListView
android:id="@+id/simpleListView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:divider="#BDB6B6"
android:dividerHeight="2dp"
tools:layout_conversion_absoluteHeight="1300dp"
tools:layout_conversion_absoluteWidth="411dp"
tools:layout_editor_absoluteX="0dp"
tools:layout_editor_absoluteY="0dp" />
</androidx.constraintlayout.widget.ConstraintLayout>
<Button
android:id="@+id/saveButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Save Image"
android:onClick="showToast" />
<TextView
android:id="@+id/textNamesView"
android:layout_width="382dp"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="Razi 380"
android:textColor="@color/black"
app:layout_constraintTop_toBottomOf="@id/icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintBottom_toTopOf="@id/saveButton" />
</androidx.constraintlayout.widget.ConstraintLayout>
how correct these errors ,make this code can run please show me the code
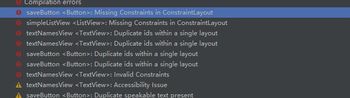
![CustomAdapter customAdapter = new CustomAdapter (getApplicationContext(), simpleList, SerialNo, flags, N
simpleList.setAdapter (customAdapter);
simpleList.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick (AdapterView<?> adapterView, View view, int position, long id) {
ImageView imageView = (ImageView) view.findViewById(R.id.imageView);
if (imageView != null) {
}
}
save Image (imageView, Names [position]);
});
// add a closing brace here](https://content.bartleby.com/qna-images/question/d7770d6e-352d-49c5-bb02-13e122a27c4b/935c1f3e-4678-4176-9dad-67ef6774894e/0tf3a5f_thumbnail.jpeg)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Create a program in Java that does the following: - Reads data from a CSV file (using the Apache Commons CSV library) - Stores the data from the CSV file in an ArrayListarrow_forwardwordBag is type BagInterface<String>. What is printed? wordBag.add("apple");wordBag.add("frog");wordBag.add("banana");wordBag.add("frog");wordBag.add("elephant"); System.out.println(wordBag.getFrequencyOf("frog"));arrow_forwardQuestion 2. package sortsearchassigncodeex1; import java.util.Scanner; import java.io.*; // // public class Sortsearchassigncodeex1 { // public static void fillArray(Scanner inputFile, int[] arrIn){ int indx = 0; //Complete code { arrIn[indx] = inputFile.nextInt(); indx++; } }arrow_forward
- import bridges.base.ColorGrid;import bridges.base.Color;public class Scene {/* Creates a Scene with a maximum capacity of Marks andwith a background color.maxMarks: the maximum capacity of MarksbackgroundColor: the background color of this Scene*/public Scene(int maxMarks, Color backgroundColor) {}// returns true if the Scene has no room for additional Marksprivate boolean isFull() {return false;}/* Adds a Mark to this Scene. When drawn, the Markwill appear on top of the background and previously added Marksm: the Mark to add*/public void addMark(Mark m) {if (isFull()) throw new IllegalStateException("No room to add more Marks");}/*Helper method: deletes the Mark at an index.If no Marks have been previously deleted, the methoddeletes the ith Mark that was added (0 based).i: the index*/protected void deleteMark(int i) {}/*Deletes all Marks from this Scene thathave a given Colorc: the Color*/public void deleteMarksByColor(Color c) {}/* draws the Marks in this Scene over a background…arrow_forwardJava code 12. Given an existing ArrayList named contactList, find the number of contacts in the ArrayList and store it in the existing variable named numContacts.arrow_forward#include <iostream>#include <cstdlib>using namespace std; class IntNode {public: IntNode(int dataInit = 0, IntNode* nextLoc = nullptr); void InsertAfter(IntNode* nodeLoc); IntNode* GetNext(); void PrintNodeData(); int GetDataVal();private: int dataVal; IntNode* nextNodePtr;}; // ConstructorIntNode::IntNode(int dataInit, IntNode* nextLoc) { this->dataVal = dataInit; this->nextNodePtr = nextLoc;} /* Insert node after this node. * Before: this -- next * After: this -- node -- next */void IntNode::InsertAfter(IntNode* nodeLoc) { IntNode* tmpNext = nullptr; tmpNext = this->nextNodePtr; // Remember next this->nextNodePtr = nodeLoc; // this -- node -- ? nodeLoc->nextNodePtr = tmpNext; // this -- node -- next} // Print dataValvoid IntNode::PrintNodeData() { cout << this->dataVal << endl;} // Grab location pointed by nextNodePtrIntNode* IntNode::GetNext() { return this->nextNodePtr;} int IntNode::GetDataVal() {…arrow_forward
- ModalProp detailsanimationType it's an enum of ('none', 'slide', 'fade') and it controls modal animation.visible its a bool that controls modal visiblity.onShow it allows passing a function that will be called once the modal has been shown.transparent bool to set transparency.onRequestClose (android) it always defining a method that will be called when user tabs back buttononOrientationChange (IOS) it always defining a method that will be called when orientation changessupportedOrientations (IOS) enum('portrait', 'portrait-upside-down', 'landscape', 'landscape-left', 'landscape-right')Modal component is a simple way to present content above an enclosing view.using given Modal make a Basic Example?arrow_forwardWhat are the Javadoc comments for each class? I am strugglingarrow_forwardFor any element in keysList with a value greater than 50, print the corresponding value in itemsList, followed by a comma (no spaces). Ex: If the input is: 32 105 101 35 10 20 30 40 the output is: 20,30, 1 #include 2 3 int main(void) { const int SIZE_LIST = 4; int keysList[SIZE_LIST]; int itemsList[SIZE_LIST]; int i; 4 6 7 8 scanf("%d", &keysList[0]); scanf ("%d", &keysList[1]); scanf("%d", &keysList[2]); scanf("%d", &keysList[3]); 10 11 12 13 scanf ("%d", &itemsList[0]); scanf ("%d", &itemsList[1]); scanf("%d", &itemsList[2]); scanf ("%d", &itemsList[3]); 14 15 16 17 18 19 /* Your code goes here */ 20 21 printf("\n"); 22 23 return 0; 24 }arrow_forward
- Fix any error in this code import org.jfree.chart.ChartFactory;import org.jfree.chart.ChartPanel;import org.jfree.chart.JFreeChart;import org.jfree.data.category.DefaultCategoryDataset;import org.jfree.ui.ApplicationFrame;import org.jfree.ui.RefineryUtilities;public class BarChartExample extends ApplicationFrame {public BarChartExample(String title) {super(title);Object ChartFactory;JFreeChart barChart = ChartFactory.createBarChart("Referral Sources Count","Referral Source","Count",createDataset(),org.jfree.chart.plot.PlotOrientation.VERTICAL,true, true, false);ChartPanel chartPanel = new ChartPanel(barChart);chartPanel.setPreferredSize(new java.awt.Dimension(560, 367));setContentPane(chartPanel);}private DefaultCategoryDataset createDataset() {DefaultCategoryDataset dataset = new DefaultCategoryDataset();String series1 = "Referral Sources";dataset.addValue(250, series1, "Website");dataset.addValue(400, series1, "Word of Mouth");dataset.addValue(300, series1,…arrow_forwardJava Given main(), complete the SongNode class to include the printSongInfo() method. Then write the Playlist class' printPlaylist() method to print all songs in the playlist. DO NOT print the dummy head node.arrow_forwardMake a class named ClassList that stores an arraylist of strings and create an add method that adds Strings to the arraylistarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
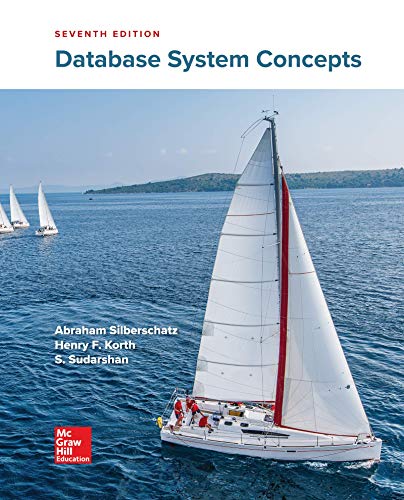
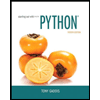
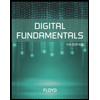
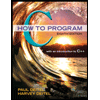
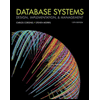
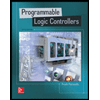