Complete a custom singly-linked LinkedList to hold generic objects. The list will use nodes called LinearNodes, which have methods getNext, setNext, getElem, and setElem. It includes methods for adding an element to a specific index or simply to the end, removing or replacing elements at specific indexes, peeking at an element without removing it, and returning the size of the list. NEEDS TESTING Keep the same format of code: private class LinkedList { private int size; private LinearNode first; private LinearNode last; public LinkedList() {
Complete a custom singly-linked LinkedList to hold generic objects. The list will use nodes called LinearNodes, which have methods getNext, setNext, getElem, and setElem. It includes methods for adding an element to a specific index or simply to the end, removing or replacing elements at specific indexes, peeking at an element without removing it, and returning the size of the list. NEEDS TESTING
Keep the same format of code:
private class LinkedList<E> {
private int size;
private LinearNode<E> first;
private LinearNode<E> last;
public LinkedList() {
}
public void add(E elem) {
}
public void add(E elem, int index) {
}
public E remove(int index) {
}
public E peek(int index) {
}
public E replace(int index, E elem) {
}
public int size() {
}
}

Step by step
Solved in 2 steps

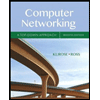
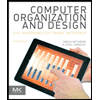
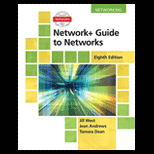
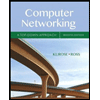
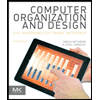
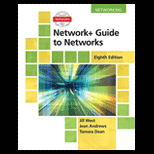
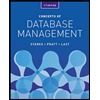
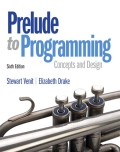
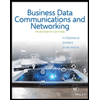