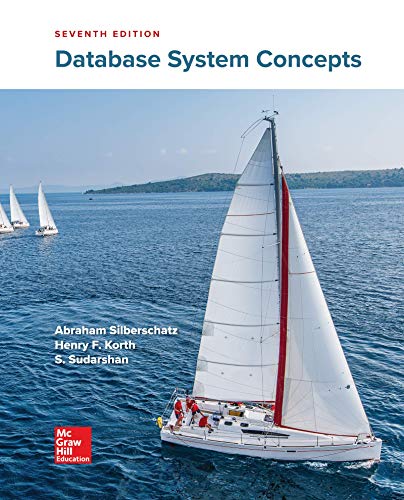
Concept explainers
Linked List, create your own code. (Do not use the build in function or classes of Java or from the textbook).
Create a LinkedList class: Call the class MyLinkedList,
(hint) Create a second class called Node.java and use it, remember in the class I put the Node class inside the LinkedList Class, but you should do it outside. This class should have
o Variables you may need for a Node,
o (optional) Constructor
Your linked list is of an int type. (you may do it as General type as <E>)
For this Linked List you need to have the following methods:
add, addAfter, remove, size, contain, toString, compare, addInOrder.
This is just a suggestion, if you use Generic type, you must modify this
Write a main function or Main class to test all the methods,
o Create a 2 linked list and test all your methods. (Including the compare)

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps

- Assignment1: Analyse the case study given in chapter 3 Linked listAnswer the following questions from Library.java1. Write the different class names with class keyword chapter 3 Linked list : https://drive.google.com/file/d/1NRN_3Fx3smkjwv1jgG8hzaiGfnNfeeDb/view?usp=sharingarrow_forwardAssume you have a class SLNode representing a node in a singly-linked list and a variable called list referencing the first element on a list of integers, as shown below: public class SLNode { private E data; private SLNode next; public SLNode( E e){ data = e; next = null; } public SLNode getNext() { return next; } public void setNext( SLNoden){ next = n; } } SLNode list; Write a fragment of Java code that would append a new node with data value 21 at the end of the list. Assume that you don't know if the list has any elements in it or not (i.e., it may be empty). Do not write a complete method, but just show a necessary fragment of code.arrow_forwardplease fix code to match "enter patients name in lbs" thank you import java.util.LinkedList;import java.util.Queue;import java.util.Scanner; interface Patient { public String displayBMICategory(double bmi); public String displayInsuranceCategory(double bmi);} public class BMI implements Patient { public static void main(String[] args) { /* * local variable for saving the patient information */ double weight = 0; String birthDate = "", name = ""; int height = 0; Scanner scan = new Scanner(System.in); String cont = ""; Queue<String> patients = new LinkedList<>(); // do while loop for keep running program till user not entered q do { System.out.print("Press Y for continue (Press q for exit!) "); cont = scan.nextLine(); if (cont.equalsIgnoreCase("q")) { System.out.println("Thank you for using BMI calculator"); break;…arrow_forward
- Pythong help!arrow_forwardIn Java. The following is a class definition of a linked list Node:class Node{int info;Node next;}Show the instructions required to create a linked list that is referenced by head and stores in order, the int values 13, 6 and 2. Assume that Node's constructor receives no parameters.arrow_forwardGiven the interface of the Linked-List struct Node{ int data; Node* next = nullptr; }; class LinkedList{ private: Node* head; Node* tail; public: display_at(int pos) const; ... }; Write a definition for a method display_at. The method takes as a parameter integer that indicates the node's position which data you need to display. Example: list: 5 -> 8 -> 3 -> 10 display_at(1); // will display 5 display_at(4); // will display 10 void LinkedList::display_at(int pos) const{ // your code will go here }arrow_forward
- This is using Data Structures in Javaarrow_forwardIn Java, Explain when it might be preferable to use a map instead of a set.arrow_forwardSend me your own work not AI generated response or plagiarised response. If I see these things, I'll give multiple downvotes and will definitely report.arrow_forward
- Give me the answer in one Visual Studio file, not Visual Studio Code - Give me full code near and simple Develop a C++ "doubly" linked list class of your own that can hold a series of signed shorts Develop the following functionality: Develop a linked list node struct/class You can use it as a subclass like in the book (Class contained inside a class) You can use it as its own separate class Your choice Maintain a private pointer to a node class pointer (head) Constructor Initialize head pointer to null Destructor Make sure to properly delete every node in your linked list push_front(value) Insert the value at the front of the linked list pop_front() Remove the node at the front of the linked list If empty, this is a no operation operator << Display the contents of the linked list just like you would print a character string operator [] Treat like an array Return the value stored in that element of the linked list If element doesn’t exist, return…arrow_forwardYou will create two programs. The first one will use the data structure Stack and the other program will use the data structure Queue. Keep in mind that you should already know from your video and free textbook that Java uses a LinkedList integration for Queue. Stack Program Create a deck of cards using an array (Array size 15). Each card is an object. So you will have to create a Card class that has a value (1 - 10, Jack, Queen, King, Ace) and suit (clubs, diamonds, heart, spade). You will create a stack and randomly pick a card from the deck to put be pushed onto the stack. You will repeat this 5 times. Then you will take cards off the top of the stack (pop) and reveal the values of the cards in the output. As a challenge, you may have the user guess the value and suit of the card at the bottom of the stack. Queue Program There is a new concert coming to town. This concert is popular and has a long line. The line uses the data structure Queue. The people in the line are objects…arrow_forwardPlease fill in all the code gaps if possible: (java) public class LinkedList { privateLinkedListNode head; **Creates a new instance of LinkedList** public LinkedList () { } public LinkedListNode getHead() public void setHead (LinkedListNode head) **Add item at the front of the linked list** public void insertFront (Object item) **Remove item at the front of linked list and return the object variable** public Object removeFront() }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
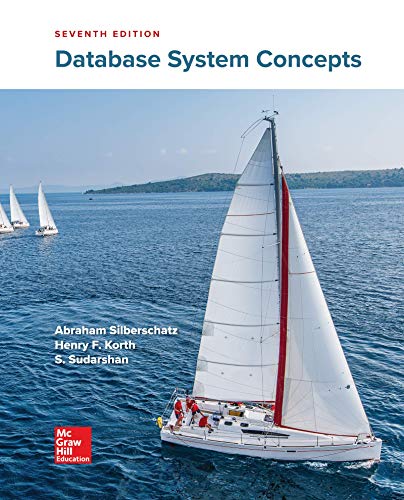
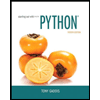
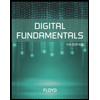
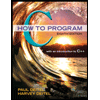
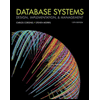
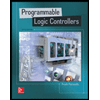