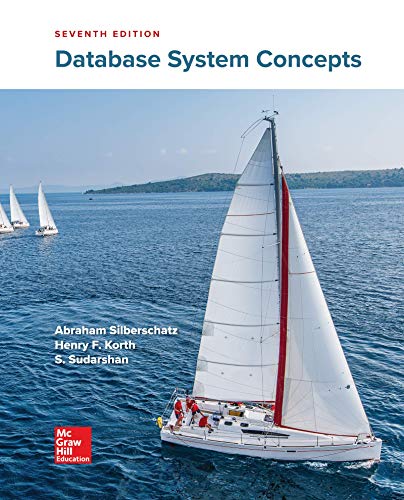
Consider the following class declarations in C++:
class C {
protected: int x;
public: void f(){...};
};
class C1: public C {
protected: int x1;
public: void h(C *obj){...};
};
class C2: public C1 {
public: int x2;
};
class C3: public C {
public: f(){...};
};
i. Draw the class hierarchy
ii. Assume that main contains the following declaration:
C1 obj1;
For each of the following expressions, say whether it is allowed in the code of main or not (they can be forbidden either because they violate the class
definition or the protection
obj1.x , obj1.f() , obj1.x1 , obj1.x2
iii. Assume that the body of
C1::h contains the following declarations
C2 *obj2;
C3 *obj3;
For each of the following expressions, say whether it is allowed in the body
of
C1::h
or not
obj->x , obj2->x , obj3->x
iv. Assume that the body of
C1::h contains the following statement
obj->f();
Assume that we call C1::h with a parameter (pointing to an object) of class
And what would be the method executed if f were declared virtual in C?

Step by stepSolved in 4 steps with 1 images

- This is for C++arrow_forwardIn C++ programing,arrow_forwardQuestion 12 Consider the following program written in C pseudocode: void foo(int x, int y){ y = y + 9; x = x + y; void main(){ int a = 6; int b = 5; foo(a, b); For each of the following parameter-passing methods, what will be the values of a and b after running the program? 1) Passed by value 2) Passed by reference 3) Passed by value-result 1.а - b = 2. a = b = 3. a = b =arrow_forward
- 2. Define a class Math in C++ that has two integer public members x,y and also, define a class member function named "add" that returns the sum of both the numbers x,y.arrow_forwardCan help with this in c++The base class is given Base: class Base { int a;double b;public:Base (int _a, double _b) : a(1), b(2.5) {}Base (int _a, double _b) :a(_a), b (_b) {}double calct () {return 1.5*a+2*b;}string toString(){return "a="+to_string(a) + "b=" + to_string(b);}};Define a class Derived, successor to Base, with an additional field d of type double. For Derived, write constructors, write a new implementation of the calc () method to add to the sum and field d with coefficient 3 and the toString () method, which returns the text description of an object of class Derived.Create objects of class Derived with the written constructors and call the two variants of the methods calc () and toString () (of the class Derived and its base class Base)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
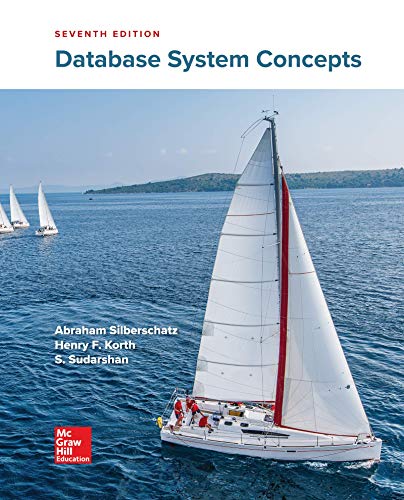
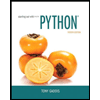
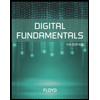
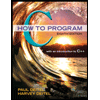
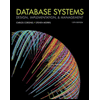
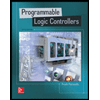