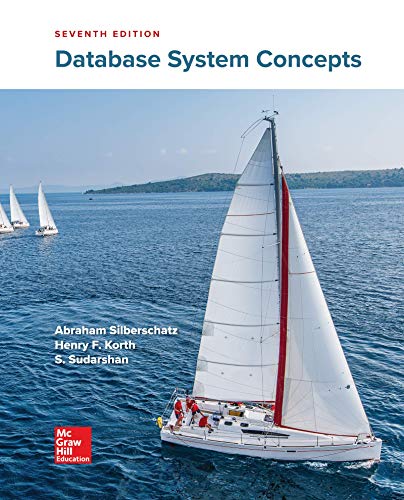
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
thumb_up100%
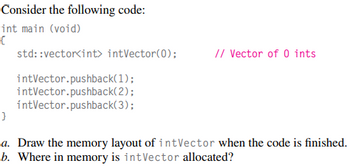
Transcribed Image Text:Consider the following code:
int main (void)
(
std::vector<int> intVector(0);
}
intVector.pushback(1);
intVector.pushback(2);
intVector.pushback(3);
// Vector of 0 ints
a. Draw the memory layout of intVector when the code is finished.
b. Where in memory is intVector allocated?
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Use a member initialization list to make the TeamInfo constructor initialize the vector listOfPointsInSeason with a size of 6. Note: Including a vector in an initialization list causes that vector's constructor to be called with the value in the parens. #include <iostream>#include <vector>using namespace std; class TeamInfo { public: TeamInfo(); void PrintGamesInSeason() const; private: vector<int> listOfPointsInSeason;}; TeamInfo::TeamInfo() : /* Your code goes here */ {} void TeamInfo::PrintGamesInSeason() const { cout << "There are " << listOfPointsInSeason.size() << " games in a season." << endl;} int main() { TeamInfo myTeam; myTeam.PrintGamesInSeason(); return 0;}arrow_forwardIn C++, define an integer vector and ask the user to give you values for the vector. Because you used a vector, so you don't need to know the size. user should be able to enter a number until he wants(you can use while loop for termination. for example if the user enters any negative number, but until he enters a positive number, your program should read and push it inside of the vector). the calculate the average of all numbers he entered.arrow_forwardC++ Program For this program you’ll write a simple class and use it in a vector.Begin by writing a Student class. The public section has the following methods: Student::Student()Constructor. Initializes all data elements: name to empty string(s), numeric variables to 0. bool Student::ReadData(istream& in)Data input. The istream should already be open. Reads the following data, in this order: First name (a string, 1 word) Last name (also a string, also 1 word) 5 quiz scores (integers, range 0-20) 3 exam scores (integers, range 0-100) Assumes that all data is separated by whitespace. The method returns true if reading all data was successful, otherwise returns false. Does not need to validate or range-check data; if one of the quiz or exam scores is out of range, just keep going. bool Student::WriteData(ostream& out) constOutput function. Writes data in the following format. Each student’s data is on one line. • First name (left justified, 20 characters)• Last name (left…arrow_forward
- You are working for a university to maintain a list of grades and some related statistics for a student. Class and Data members: Create a class called Student that stores a student’s grades (integers) in a vector (do not use an array). The class should have data members that store a student’s name and the course for which the grades are earned. Constructor(s): The class should have a 2-argument constructor that receives the student’s name and course as parameters and sets the appropriate data members to these values. Member Functions: The class should have functions as follows: Member functions to set and get the student’s name and course variables. A member function that adds a single grade to the vector. Only positive grades are allowed. Call this function AddGrade. A member function to sort the vector in ascending order. A member function to compute the average (x̄) of the grades in the vector. The formula for calculating an average is x̄ = ∑xi / n where xi is the value of each…arrow_forward#include #include using std::cout; using std::vector; class StatSet { private: HCreate a vector of doubles that is called "numbers." public: //Nothing to do in the constructor. StatSet() } // Add a new number to the set. vòid add_num(double num) { } double mean() { //Sum up the values and return the average. double sum = 0; //Write a loop that would iterate through the vector. sum += numbers[i]; return sum / numbers.size(); } double median() //Before calculating the median, we must sort the //array.arrow_forwardEvery data structure that we use in computer science has its weaknesses and strengthsHaving a full understanding of each will help make us better programmers!For this experiment, let's work with STL vectors and STL dequesFull requirements descriptions are found in the source code file Part 1Work with inserting elements at the front of a vector and a deque (30%) Part 2Work with inserting elements at the back of a vector and a deque (30%) Part 3Work with inserting elements in the middle, and removing elements from, a vector and a deque (40%) Please make sure to put your code specifically where it is asked for, and no where elseDo not modify any of the code you already see in the template file This C++ source code file is required to complete this problemarrow_forward
- Select all code lines that creates a vector with content {10, 10, 10, 10). You are also given the C-type array, an_array with content (10, 10, 10, 10). std::vector a_vector (an_array, an_array + sizeof(an_array)/sizeof(int)); std::vector a_vector [10, 10, 10, 10); std::vector a_vector (10, 4); std::vector a_vector = {10, 10, 10, 10); Ostd::vector a_vector (4, 10);arrow_forward#include <iostream>#include <vector>using namespace std; void PrintVectors(vector<int> numsList) { unsigned int i; for (i = 0; i < numsList.size(); ++i) { cout << numsList.at(i) << " "; } cout << endl;} int main() { vector<int> numsList; int userInput; int i; for (i = 0; i < 3; ++i) { cin >> userInput; numsList.push_back(userInput); } numsList.erase(numsList.begin()+1); numsList.insert(numsList.begin()+1, 102); numsList.insert(numsList.begin()+1, 100); PrintVectors(numsList); return 0;} Not all tests passed clearTesting with inputs: 33 200 10 Output differs. See highlights below. Special character legend Your output 33 100 102 10 Expected output 100 33 102 10 clearTesting with inputs: 6 7 8 Output differs. See highlights below. Special character legend Your output 6 100 102 8 Expected output 100 6 102 8 Not sure what I did wrong but the the 33…arrow_forwardplease help me with codingarrow_forward
- Complete the C++ code below#include "std_lib_facilities.h"/*1. Define a function which calculates the outer product of two vectors. The function return is a matrix. */vector<vector<int>> outerProduct(vector<int>& A, vector<int>& B){//implement here}/*2. Define a function which transposes a matrix. */vector<vector<int>> transpose(vector<vector<int>>& A){//implementation}/*3. Define a function which calculates the multiplication of a matrix and it's transpose. */vector<vector<int>> product(vector<vector<int>>& A){// implementation}/*4. Define a print out function that will print out a matrix in the following format:Example: a 3 by 4 matrix should have the print out:1 2 3 42 3 4 53 4 5 6*/void printMatrix(vector<vector<int>>& A){// implementation}int main(){// Define both vector A and vector Bvector<int> A = {1 ,2, 3, 4, 5};vector<int> B = {1, 2, 3};/*Test outerProduct…arrow_forwardInstructions: Turn all instances of classes into pointers. You will also need to combine the player and vector into one vector objects and fix all issues this causes. #ifndef ITEM_H #define ITEM_H class Item { public: Item() {}; enum class Type { sword, armor, shield, numTypes }; Item(Type classification, int bonusValue); Type getClassification() const; int getBonusValue() const; private: Type classification{ Type::numTypes }; int bonusValue{ 0 }; }; std::ostream& operator<< (std::ostream& o, const Item& src); bool operator< (const Item& srcL, const Item& srcR); int& operator+=(int& srcL, const Item& srcR); #endif // !ITEM_H #ifndef MONSTER_H #define MONSTER_H #include "Object.h" class Player; class Monster : public Object { public: Monster() {}; Monster(const Player& player); void update(Player& player, std::vector& monsters) override; int attack() const override; void defend(int damage) override; void print(std::ostream& o)…arrow_forward4arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
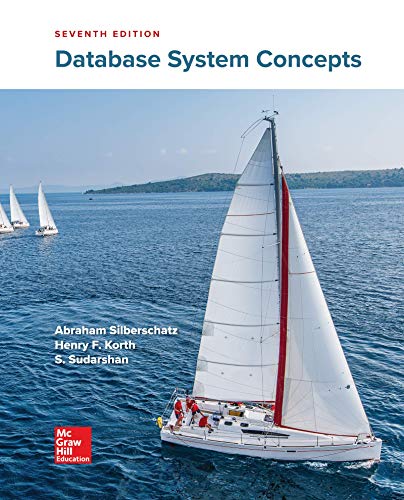
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
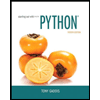
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
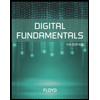
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
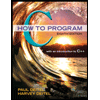
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
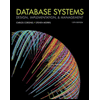
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
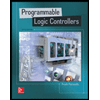
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education