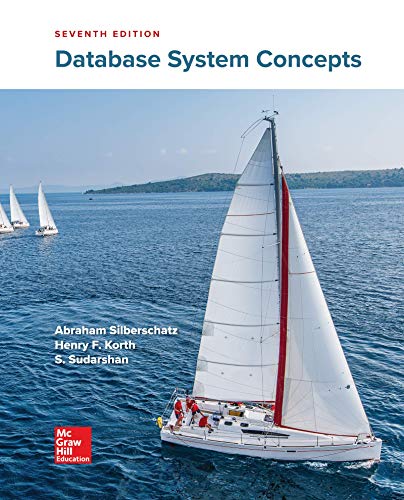
Concept explainers
Convert the given code fragment to assembly code fragment,
using only instructions of the following types.
These instructions are generally discussed in class. Here
X,Y,Z are any memory locations; R, R1, R2 are any general registers; L is a label in the code (you can use any names as labels, ex. L, L1, L2 etc. ).
- load X, R //copy contents of memory location X into R.
- store R, X //Store contents of R into Mem location X
- cmp R1, R2 //Compute R1-R2 and update condition codes;
//throw away result of subtraction.
- jmp L //Jump to location L in the code.
- jmpp L //If P bit is 1, Jump to location L in the code
- add X, R //Add contents of X,R and store result in R;
//Also update the condition codes.
Be careful about what type of argument is allowed in the instruction (Memory or Register). Ex. the first argument of ADD instruction is memory, not register.
Do Not use any other instructions (in this, or some other, assembly language).
I cannot get into syntax and semantics of your assembly language or its presumed cpu architecture.
So follow the instructions in the question.
Ex. do not use JMPZ, JMPN etc. in these questions.
Ex. Do not say: cmp X, Y. The question says that the two arguments in the cmp instruction are registers, not memory locations.
The code segment:
while ((X != Y) X = X + Z;
L:

Answer:
The given code is
if((X + Y ) > 0)
X = X + X;
L:
The assembly code is given below:
load X, R1 / /copy content of the memery location X to R1
load Y, R2 // copy content of the memory location Y to R2
Step by stepSolved in 2 steps

- Example: The Problem Input File Using C programming language write a program that simulates a variant of the Tiny Machine Architecture. In this implementation memory (RAM) is split into Instruction Memory (IM) and Data Memory (DM). Your code must implement the basic instruction set architecture (ISA) of the Tiny Machine Architecture: //IN 5 //OUT 7 //STORE O //IN 5 //OUT 7 //STORE 1 //LOAD O //SUB 1 55 67 30 55 67 1 LOAD 2- ADD 3> STORE 4> SUB 5> IN 6> OUT 7> END 8> JMP 9> SKIPZ 31 10 41 30 //STORE O 67 //OUT 7 11 /LOAD 1 //OUT 7 //END 67 70 Output Specifications Each piece of the architecture must be accurately represented in your code (Instruction Register, Program Counter, Memory Address Registers, Instruction Memory, Data Memory, Memory Data Registers, and Accumulator). Data Memory will be represented by an integer array. Your Program Counter will begin pointing to the first instruction of the program. Your simulator should provide output according to the input file. Along with…arrow_forwardGiven the following code, show step by step and explain how variables are created and eliminated in RAM memory. void p(int& a, float b) { a=1; b=4.0f; } int main(void) { float a=3; int c=4; p(c,a); }arrow_forwardWrite program segments that perform the operation C C+ A x B using each of the instruction classes indicated in Exercise 1 above. Assume that A, B, and C are memory 3. addresses. lleuing data Lin thearrow_forward
- Answser must be in MIPSzy assembly language. Max of 3 - Brancharrow_forwardYou must complete this in Python and the programs should not take any command-line arguments. You also need to make sure your programs will compile and run in at least a Linux environment. In this problem, you need to implement operation bit encoding for Assembly instructions. Given a line of text, your program should check whether it is a valid Assembly instruction type. If it is, your program should print out the opcode and optype of that instruction type; if the line of text is not exactly a valid Assembly instruction, your program should skip it and move to the next line of input without printing anything. Input Format The input to the program will consist of some number of lines. Each line contains some text, either a valid Assembly instruction type (with no extra whitespace or other characters, e.g. READ on a line by itself) or some other text. Constraints There are no specific constraints on the length or number of lines. They will be in a reasonable limit, as demonstrated by…arrow_forwardYou must complete this in Python and the programs should not take any command-line arguments. You also need to make sure your programs will compile and run in at least a Linux environment. In this problem, you must implement a tokenizer for the Assembly instruction format: an operation type (e.g. SUBI) followed by a comma-separated list of parameters (e.g. R0, R1, 6). If a line has an invalid syntax, it should be skipped in the output. You do not need to check whether the operations and arguments are valid Assembly instructions: you just need to separate them into tokens. Input Format The input to the program will consist of some number of lines. Each line is of the following form: "op arg1, arg2, ..., argn". Your program should terminate upon receiving a blank line or EOF. Constraints There are no specific constraints on the length or number of lines. They will be in a reasonable limit, as demonstrated by the included test cases, all of which are public. This is not a…arrow_forward
- Computer organization and assembly language Please help me with this. I have to write line by line what each line of codes does. CODE IS BELOW: .model small .386 .stack 100h .data msg1 db 13, 10, "Enter any number --> ", "$" msg2 db "Enter an operation +,- * or / --> ",13, 10, "$" msg3 db "The Operation is --> ", "$" msg4 db "The result is --> ", "$" By_base dd 21 by_10 dd 10 ; 32 bits variable with initial value = 10 sp_counter db 0 ; 8 bits variable with initial value of zero disp_number dd 0 ; 32 bits variable with initial value = 0 disp_number2 dd 0 disp_number3 dd 0 op_type db 0 last_key dd 0 ; 32 bits variable with initial value of zero remainder db 0 .code main proc mov ax,@data;set up datasegment movds,ax mov dx,offset msg1 call display_message callm_keyin calloperation mov dx,offset msg1 calldisplay_message callm_keyin cmpop_type, "+" jnz short skip_plus callop_plus skiP_plus: cmp op_type, "-" jnz short skip_minus callop_minus…arrow_forwardAssume a computer system has a main memory of 256 Bytes. The following is a memory byte-access trace history of a program run on this system. For example, as it is shown, the program first accesses memory address 0000 0000, and then it accesses memory address 0000 0001 and so on. Note the memory addresses are represented in binary: 00000000, 00000001, 00000010, 00000011, 00001000, 00010000, 00010001, 00000100, 00000101, 00000110, 00000111, 00001001, 00001010, 00001011, 00001100, 00001000, 00001001, 00001010, 00011100, 00011101.arrow_forwardMicroprocessor8086 Write the assembly of Write on the data segment and create number1 and number2 variables in byte size and initialize the variables Then compute the sum ,subtraction,multiplication and division of the defined variables and load the values to the variables which you create on the data segment Perform the operations below in sequence with defined on the data segment numbers in array Find the sum of array and average of array Find the maximum and minimum numbers of array If it’s possible on emu8086 apparrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
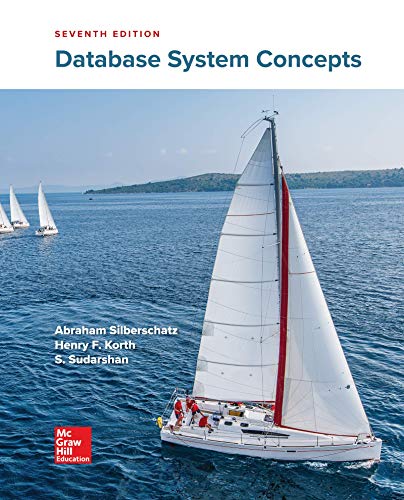
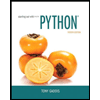
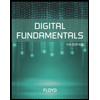
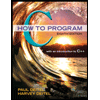
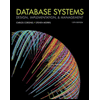
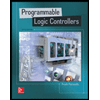