Create a Java program where the user will have to enter two values(Using JOptionPane) to be divided.
Q: Consider a 6-bit two’s complement representation. Fill in the box with question mark "?" in the foll...
A: In the Given Question we represent binary and decimal representation of Given Number
Q: Explain specifically why the following instruction set may not work correctly. mov ах, -10 mov сх, 7...
A: Answer: I have done code and also I have attached code.
Q: Design a class named Complex for representing complex numbers with the methods add, subtract, multip...
A: the answer is given below:-
Q: VHDL Coding (Circuit Diagram is given ~ Create it's Structural and Behavioral VHDL Program Please ma...
A: VHDL Program EDAPlayground is working
Q: Which of these statements is/are true? а)0 € 0 c){0} C Ø e){0} € {0} b)Ø e {0} d)Ø C {0} f){0} c {Ø}...
A: Here, we are going to find out which statements are true for given sets in Q.1 And in Q2, we have to...
Q: -In CMOS gates, what occurs when the frequency of the input signal increas A) The power dissipation ...
A: Question: In CMOS gates, what occurs when the frequency of the input signal increases: A) The power ...
Q: You are configuring a connection between two backbone switches, and you want to make sure the conne...
A: Round-robin DNS is a load balancing technique where the balancing is done by a type of DNS server ca...
Q: Using public Wi-Fi to conduct business is fraught with hazard
A: Dangers of Public Wi-Fi: A wi-fi connection is a kind of tool that electronic devices use to connec...
Q: Is the internet considered to be a local area network?
A: According to the question Local area network is the most common network which we can use to get inte...
Q: What role does WiFi play in computer adoption?
A: Introduction: Wi-Fi is a wireless technology that connects computers, tablets, smartphones, and othe...
Q: What exactly is SQL, and why is it such a popular database language?
A: Introduction SQL stands for Structured Query Language, and it is a programming language used to stor...
Q: Q.1: MIPS is an architecture having its own Assembly Language. You are learning x86 assembly Languag...
A: a) The main tools to write programs in x86 assembly are the processor registers. The registers are l...
Q: Java programming Write a method (void banana(int a)) that draws the following figure: a triangle wi...
A: Given: Java programming Write a method (void banana(int a)) that draws the following figure: a trian...
Q: Consider the following code segment: public void wow (int n) if (n> 1) wow (n/2); System.out print(n...
A: Ans :- option 3 is correct
Q: Which of the following is NOT a strategy for dealing with Porter's competitive forces? OA. Tradition...
A: Below is the answer with explanation :
Q: Q4/ A- Find the complement of F=WX +YZ, then show that F.F=o and F+F=1
A: Logical gates are the basic building blocks of any digital signalling system. It takes one or more i...
Q: Sub:- Multimedia and Application Development Discuss various software tools available for graphics ...
A: The Answer is in below Steps
Q: Write a program that reads the student information from a tab separated values (tsv) file. The progr...
A: Java code is given below
Q: 1. Explore Scilab
A: NOTE: ACCORDING TO COMPANY POLICY WE CAN SOLVE ONLY 1 QUESTION. YOU CAN RESUBMIT THE QUESTION AGAIN ...
Q: For a "word" of 3 bits, make a table that lists all the signed binary numbers representable by the f...
A: ans is given below
Q: Create a program that contains a class that converts dollars to nickels (5 cents), dimes (10 cents) ...
A: public class Total_Dollar { public static void main(String[] args) { int q; int d; ...
Q: Question 1 Flow control is one of the main building blocks that determines how a program should run...
A: TCP Dynamic Allocation (TCP Flowing Regulation): This technique of regulating the speed at which dat...
Q: Write a full class that represents a Phone, described by a name, price, and then whether the phone c...
A: PROGRAM EXPLANATION: Import the java. util package. Create a phone class. Declare the attributes of...
Q: Explain what Zenmap is and discuss how hackers can use Zenmap to attack a network or system.
A: Zenmap is the graohical user interface of Nmap or network mapper It is a vast tool and have many use...
Q: apter 26 – Exercise 2 from book Database and Application Security by Bhavani Thuraisingham Develop a...
A: The term "workflow" describes a work process that consists of several individual and often repeatabl...
Q: Use an example to explain how to use ravel(), reshape() and resize() on a 3D NumPy array
A: the answer is
Q: Description Write a Java program, called LinearSearch1 that contains a static method called findEle...
A:
Q: B- Answer the following questions? 1. Multiply (10010), (11110), using binary multiplication method?...
A: Binary numbers are those numbers that are converted from decimal to binary by dividing by 2 and taki...
Q: Given the following sets: A = {2, 3, 4}, B = {1, 5, 10}, C = {-1, 1, 2}, D = {2, 5, 10} What is the ...
A: Note: This is multiple questions-based problem. As per company guidelines, only the first question i...
Q: What protocol is used to assign dynamic IP addresses to computers on a local area network?
A: We are going to understand which protocol is used to assign dynamic IP addresses to computers on a l...
Q: I'm wondering what the distinctions are between commercial and personal cloud service providers. Exa...
A: Introduction: Data storage management and security are critical goals for cloud service providers to...
Q: Explain the function of a programmer-defined break point.
A: A point in the processing of a program that the programmer wants to observe more closely by stopping...
Q: Use the "it-else To design, develop enter three numbers and the product of those three numbers (the ...
A: First program - import java.util.*; public class Main{ public static void main(String[] args) { Sca...
Q: The metrics that are calculated for the training set measures the goodness of fit of the fitted mode...
A: Training data is the initial data used to train machine learning models.
Q: For the following instruction: sltu $s0, $t2, $t3 what the content of the register ($s0) after exe...
A: - We need to show the value of $s0 register.
Q: What's the difference between event bubbling and capturing an event?
A: Introduction: HTML DOM API event bubbling and event capturing are two methods of propagating events ...
Q: Read a variable n from the user and generate a series of Fibonacci numbers up to the given n. The di...
A: The Fibonacci numbers are the numbers in the following integer sequence.0, 1, 1, 2, 3, 5, 8, 13, 21,...
Q: For a "word" of 3 bits, make a table that lists all the signed binary numbers representable by the f...
A: A) Signed magnitude:- The maximum number that can be represented by 3 bit is +3 and minimum number...
Q: What is the number of states required in minimal DFA to accept the strings of a regular language tha...
A: Given: Goal: Design minimum state DFA and report the minimum number of states.
Q: A summary on computer crime, viruses, and worms.
A: Computer crime: The Internet is a fantastic place full of fascinating possibilities. However, there ...
Q: Briefly explain the stages of BIG DATA BUSINESS ANALYTICS
A: INTRODUCTION: Big data analytics is essentially a catch-all term for processing enormous amounts of...
Q: Write the commands required to configure the IPv6 address 2001:db8:acad:1::1/64 on a router interfac...
A: Command used to configure the IPv6 address on an interface is: ipv6 address address/prefix-length e...
Q: OP? a) Andrea Ferro b) Adele Goldberg c) Alan Kay d) Dennis Ritchie
A: given - Who invented OOP?a) Andrea Ferrob) Adele Goldbergc) Alan Kayd) Dennis Ritchie
Q: 14. What will be the output of the following Python code? x = 'abcd' for i in x: print (i.upper ()) ...
A: #14#lets analyse the code for outputx='abcd'for i in x:#for each character in x print(i.upper())#...
Q: 5. Define a function in PHP language that takes a sorted list as the parameter and returns a list af...
A: Answer: I have done code and also I have attached code and code screenshot as well as output
Q: numbers = (34, 42, 55, 53, 30, 40, 93, 65, 57, 86) Partition(numbers, 0, 5) is called. Assume quic...
A: The solution to the given problem is below.
Q: Which of the ff. input string/s is/are accepted by the automaton? 1. S2 So Is 1
A: Given , a Automata We have given multiple long input string and we have asked to find which input...
Q: Computer Science Provide a recursive definition for the following set S. ?={2k3m5n ∈ ? | ?, ?, ? ∈ ...
A: Given :- Provide a recursive definition for thefollowing set S.S={2k3m5n E N | k, m, n E Z+}Z+ is th...
Q: You are configuring a connection between two backbone switches, and you want to make sure the conne...
A: You are configuring a connection between two backbone switches, and you want to make sure the connec...
Q: What error might we get when running the following code? Assume obj is an instance of some class. ob...
A: b. TypeError: robo() takes 3 positional arguments but 2 were given.
Create a Java program where the user will have to enter two values(Using JOptionPane) to be divided. The application catches an exception if one of the entered values is not an integer. Declare three integers—two to be input by the user and a third to hold the result after dividing the first two.
Add a try block that prompts the user for two values, converts each entered String to an integer, and divides the values, producing result.
Add a catch block that catches an ArithmeticException object if division by 0 is attempted. If this block executes, display an error message, and force result to 0.
catch(ArithmeticException exception)
{
JOptionPane.showMessageDialog(null, exception.getMessage());
result = 0;
}

Step by step
Solved in 2 steps with 3 images

- Write a Java program to read the mobile number of an Employee. If the mobile number does not start with 9 or doesn't contain exactly 8 digits, throw a user defined exception InvalidNumberException. If the number entered is valid, display the message “correct mobile number is entered!” otherwise display “Entered mobile number is invalid!!” [Hint: Use String.valueOf(num).length() methods to first convert the gsm number (num) to string and then find the length of the string]Design and implement a program that reads an integer value (let's say x) as a command line argument. Further, the program reads a series of 'x' integer values from the user and prints their average. Read each input value as a String, and then attempt to convert it to an integer. If this process throws an exception (meaning that the input is not a valid number), print the appropriate error message and prompt for the number again. Continue reading values until 'x' valid integers have been entered. Hint: Think of all possible errors that this process could cause, and incorporate appropriate exception handlers in your programs. Note: You cannot use the root/general exception class 'Exception'Write a program that reads integers user_num and div_num as input, and output the quotient (user_num divided by div_num). Use a try block to perform all the statements. Use an except block to catch any ZeroDivisionError and output an exception message. Use another except block to catch any ValueError caused by invalid input and output an exception message. Note: ZeroDivisionError is thrown when a division by zero happens. ValueError is thrown when a user enters a value of different data type than what is defined in the program. Do not include code to throw any exception in the program. Ex: If the input of the program is: 15 3 the output of the program is: 5 Ex: If the input of the program is: 10 0 the output of the program is: Zero Division Exception: integer division or modulo by zero Ex: If the input of the program is: 15.5 5 the output of the program is: Input Exception: invalid literal for int() with base 10: '15.5' My code: # Type your code here.user_num = input()div_num =…
- Write a program that reads integers user_num and div_num as input, and output the quotient (user_num divided by div_num). Use a try block to perform all the statements. Use an except block to catch any ZeroDivisionError and output an exception message. Use another except block to catch any ValueError caused by invalid input and output an exception message. Note: ZeroDivisionError is thrown when a division by zero happens. ValueError is thrown when a user enters a value of different data type than what is defined in the program. Do not include code to throw any exception in the program. Ex: If the input of the program is: 15 3 the output of the program is: 5 Ex: If the input of the program is: 10 0 the output of the program is: Zero Division Exception: integer division or modulo by zero Ex: If the input of the program is: 15.5 5 the output of the program is: Input Exception: invalid literal for int() with base 10: '15.5' My code: # Type your code here.user_num = int(input())div_num =…PYTHON: Write a program that reads integers user_num and div_num as input, and output the quotient (user_num divided by div_num). Use a try block to perform all the statements. Use an except block to catch any ZeroDivisionError and output an exception message. Use another except block to catch any ValueError caused by invalid input and output an exception message. Note: ZeroDivisionError is thrown when a division by zero happens. ValueError is thrown when a user enters a value of different data type than what is defined in the program. Do not include code to throw any exception in the program. Ex: If the input of the program is: 15 3 the output of the program is: 5 Ex: If the input of the program is: 10 0 the output of the program is: Zero Division Exception: integer division or modulo by zero Ex: If the input of the program is: 15.5 5 the output of the program is: Input Exception: invalid literal for int() with base 10: '15.5'Write a Java application with comments, that throws and catches an ArithmeticException when you attempt to take the square root of a negative value. Prompt the user for an input value and try the Math.sqrt() method on it. The application either displays the square root or catches the thrown Exception and displays an appropriate message.
- Please written by computer source Implement try/except exception handler to catch all errors (from the following source code) separately: my_string = 'Hello World' print(my_string) num = int(my_string) print(my_string + 100) num = 1/0 print(total) print('Done') Submission Instructions: 1. Write all the code in one module (in one .py file), and save it as Firstname_Lastname_hw6.py (e.g., John Adam’s file name should be John_ Adam_hw6.py).Write a JAVA program to calculate percentage of a student for 3 different subjects. Create an exception if the marks entered greater than 100.Write a program that reads integers user_num and div_num as input, and output the quotient (user_num divided by div_num). Use a try block to perform all the statements. Use an except block to catch any ZeroDivisionError as a variable and output "Zero Division Exception: " followed by the exception message from the variable. Use another except block to catch any ValueError caused by invalid input as a variable and output "Input Exception: " followed by the exception message from the variable. Note: ZeroDivisionError is raised when a division by zero happens. ValueError is raised when a user enters a value of different data type than what is defined in the program. Do not include code to raise any exception in the program. (in Python)
- Write a program that reads integers user_num and div_num as input, and output the quotient (user_num divided by div_num). Use a try block to perform all the statements. Use an except block to catch any ZeroDivisionError as a variable and output "Zero Division Exception: " followed by the exception message from the variable. Use another except block to catch any ValueError caused by invalid input as a variable and output "Input Exception: " followed by the exception message from the variable. Note: ZeroDivisionError is raised when a division by zero happens. ValueError is raised when a user enters a value of different data type than what is defined in the program. Do not include code to raise any exception in the program. Ex: If the input of the program is: 15 3 the output of the program is: 5 Ex: If the input of the program is: 10 0 the output of the program is: Zero Division Exception: integer division or modulo by zero Ex: If the input of the program is: 15.5 5 the output of…i keep getting the errors of InputBook1Author110.99200Book2Author219.99100Book3Author225.00600Book4Author35.0020Book5Author48.00120OutputEnter the title of the book: Enter the name of the author: Enter the price of the book: Unhandled Exception:BookException: For Book1, ratio is invalid. Price is ¤10.99 for 0 pages. at Book.set_Price (System.Decimal value) [0x0005a] in <b0c20e1170ce40c5aed6ba11d9a91b3c>:0 at BookExceptionDemo.Main () [0x0006e] in <b0c20e1170ce40c5aed6ba11d9a91b3c>:0 [ERROR] FATAL UNHANDLED EXCEPTION: BookException: For Book1, ratio is invalid. Price is ¤10.99 for 0 pages. at Book.set_Price (System.Decimal value) [0x0005a] in <b0c20e1170ce40c5aed6ba11d9a91b3c>:0 at BookExceptionDemo.Main () [0x0006e] in <b0c20e1170ce40c5aed6ba11d9a91b3c>:0 ResultsFor Book2, ratio is invalid....Price is $19.99 for 100 pages.For Book4, ratio is invalid....Price is $5.00 for 20 pages.Book1 by Author1 Price $10.99 200 pages.Book2 by Author2 Price $10.00 100…Using JavaFX, create a simple calculator application, like the following: The operators is ( + : " addition process" , -:subtraction process", *:"multiplication process" , /:"division process" , C : "Clear" , H: "History"); Validate that the first number and second is a numeric value if it is not throwing an exception. When clicking the operators (+, -, *, /): the result will display at the bottom, and stored in history. When clicking C, clear the first, second number and the history5 When clicking H, the history will display at the bottom.6 When the user click / and the second number is 0 throw an arithmetic exception.
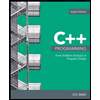
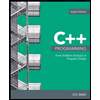