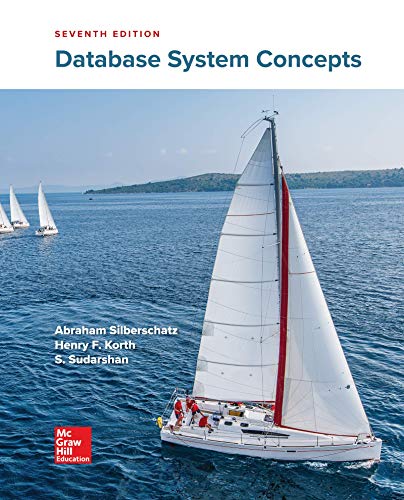
Concept explainers
Write a Java program that repeatedly accepts two integers from the keyboard and prints the quotient to
the console. Your code must adhere to the follow specifications:
• The user must enter 0 for both the numerator and denominator to exit the program.
• You must read the input values using the Scanner’s next() method and then convert to a
primitive integer using Integer.parseInt. In case of an exception (when converting the
string to an integer), the program must (1) print the corresponding stack trace
(printStackTrace) and (2) reset the Scanner object.
• The program must catch division by zero exceptions and print a corresponding message in case
one occurs. The error should not be printed if the user intends to exit.
• The sample input/output is given below for your reference:
Give me an int numerator: 0
Give me an int denominator: 1
The quotient is: 0
Give me an int numerator: 1
Give me an int denominator: 0
An exception occurred. See below for more info:
java.lang.ArithmeticException: / by zero
at DemoClass.main(DemoClass.java:20)
Give me an int numerator: 4
Give me an int denominator: 2
The quotient is: 2
Give me an int numerator: 2
Give me an int denominator: 4
The quotient is: 0
Give me an int numerator: 2
Give me an int denominator: two
An exception occurred. See below for more info:
java.lang.NumberFormatException: For input string: "two"
at
java.base/java.lang.NumberFormatException.forInputString(NumberFormatException.java:68)
at java.base/java.lang.Integer.parseInt(Integer.java:652)
at java.base/java.lang.Integer.parseInt(Integer.java:770)
at DemoClass.main(DemoClass.java:16)
Give me an int numerator: two
An exception occurred. See below for more info:
java.lang.NumberFormatException: For input string: "two"
at
java.base/java.lang.NumberFormatException.forInputString(NumberFormatException.java:68)
at java.base/java.lang.Integer.parseInt(Integer.java:652)
at java.base/java.lang.Integer.parseInt(Integer.java:770)
at DemoClass.main(DemoClass.java:13)
Give me an int numerator: 0
Give me an int denominator: 0
Program over!

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- The while loop reads values from input until an integer is read. Complete the exception handler in the while loop to catch an InputMismatchException exception and: Output "Removed unacceptable input for number of cents". End with a newline. Discard the invalid input. Ex: If the input is 20 60, then the output is: 20 cents = 4 nickels Processed one valid input value Ex: If the input is Aya Tia 20 60, then the output is: New JAVA code can only be added after code given: Removed unacceptable input for number of cents Removed unacceptable input for number of cents 20 cents = 4 nickels Processed one valid input value Note: Each value read from input is on one line. /******************************************************************************************************* New Java Code here: test input: Aya Tia 20 60 *******************************************************************************************************/arrow_forwardWrite a program that prompts the user to enter several integer numbers and store them in a one dimensional array. The size of the array should be read from the keyboard. The program should calculate and display the average of the numbers. If the user enters a value for the array size less than one, throw and handle an appropriate exception (you have to display the message array size cannot be less than one) and prompt the user to enter another array size. This process continue until the user enters the correct array size. In addition, the program should be able to handle exception thrown when there is a division by zero problem, when the index of the array is out of bound, when there is input mismatch. SLOVE IT USING JGRASP PLEASEarrow_forwardQuestion 22 Write a program that reads integers user_num and div_num as input, and output the quotient (user_num divided by div_num). Use a try block to perform all the statements. Use an except block to catch any ZeroDivisionError and output an exception message. Use another except block to catch any ValueError caused by invalid input and output an exception message. Please do it ONLY in Python Language also inlcude the input and output screenshotarrow_forward
- This code is only for python. this is a game- transfer rings there are 3 problems which you are solving: A: failed transfer - make sure the progam dont take more than what the user inputed by raising an exceptionB: codE the trade_money function C: if sonic doesnt have as much as the user inputed, there will be an error pop up 'ERROR TRADE FAILED'. make it so the progam can run normally rather than turning it down. CODE: class user:def __init__(self, name, initial_money):self.name = nameself.money = initial_moneydef give_money(self, amount):self.money = self.money + amountdef take_money(self, amount):# Part A: Raise an exception as appropriateself.money = self.money - amountdef print_users(users):total_money = 0for user in users:print(f'{user.name:s} has {user.money:d} rings')total_money = total_money + user.moneyprint(f'There are a total of {total_money:d} rings')# B: code the trade_money functionsonic = user('sonic', 80)tails = user('tails', 20)print_users([sonic,…arrow_forwardThe given program reads a list of single-word first names and ages (ending with-1), and outputs that list with the age incremented. The program fails and throws an exception if the second input on a line is a string rather than an integer. At FIXME in the code, add try and except blocks to catch the ValueError exception and output 0 for the age. Ex: If the input is: Lee 18 Lua 21 Mary Beth 19 Stu 33 -1 then the output is: Lee 19 Lua 22 Mary 0 Stu 34arrow_forwardWrite a program that reads integers user_num and div_num as input, and output the quotient (user_num divided by div_num). Use a try block to perform all the statements. Use an except block to catch any ZeroDivisionError as a variable and output "Zero Division Exception: " followed by the exception message from the variable. Use another except block to catch any ValueError caused by invalid input as a variable and output "Input Exception: " followed by the exception message from the variable. Note: ZeroDivisionError is raised when a division by zero happens. ValueError is raised when a user enters a value of different data type than what is defined in the program. Do not include code to raise any exception in the program. (in Python)arrow_forward
- Again --4. Write a program that calculates an adult's fat-burning heart rate, which is 70% of 220 minus the person's age. Complete fat_burning_heart_rate() to calculate the fat burning heart rate. The adult's age must be between the ages of 18 and 75 inclusive. If the age entered is not in this range, raise a ValueError exception in get_age() with the message "Invalid age." Handle the exception in __main__ and print the ValueError message along with "Could not calculate heart rate info." Ex: If the input is: 35 the output is: Fat burning heart rate for a 35 year-old: 129.5 bpm If the input is: 17 the output is: Invalid age. Could not calculate heart rate info.?.arrow_forward1. When does the + operator concatenate instead of add? What happens if you try to use + on a string with an integer? Why do you think this happens? 2. If a string has 10 characters, what is the index of the last character? 3. Explain why this code causes an exception: Animal = "Tiger" Animal[0] = "L"arrow_forwardIn Java: Forms often allow a user to enter an integer. Write a program that takes in a string representing an integer as input, and outputs yes if every character is a digit 0-9. Ex: If the input is: 1995 the output is: yes Ex: If the input is: 42,000 or 1995! the output is: no Hint: Use a loop and the Character.isDigit() function.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
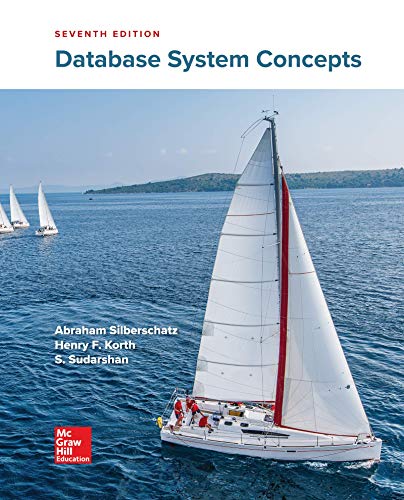
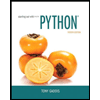
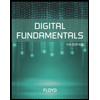
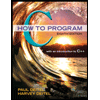
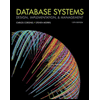
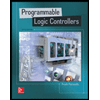