Create a new Java project named Program 4 Three Dimensional Shapes. In this project, create a package named threeDimensional and put all 3 of the classes discussed below in this package Javadoc requires that every class, every field, every constructor, and every method have a general (useful) comment and all relevant tags. This includes @param tags for methods and constructors and @param and @return tags for methods.
Create a new Java project named Program 4 Three Dimensional Shapes. In this project, create a package named threeDimensional and put all 3 of the classes discussed below in this package Javadoc requires that every class, every field, every constructor, and every method have a general (useful) comment and all relevant tags. This includes @param tags for methods and constructors and @param and @return tags for methods.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Create a new Java project named Program 4 Three Dimensional Shapes. In this project, create a package named threeDimensional and put all 3 of the classes discussed below in this package
Javadoc requires that every class, every field, every constructor, and every method have a general (useful) comment and all relevant tags. This includes @param tags for methods and constructors and @param and @return tags for methods.
- Create a Cube class with the following:
- 1 data member: an integer named lengthOfSide
- 2 constructors: a no-argument constructor that set lengthOfSide to 1 and one that takes lengthOfSide as an argument
- A getter and a setter for lengthOfSide
- A toString() method for Cube that simply returns the string "Cube: [lengthOfSide]"
- A getSurfaceArea() method that returns the surface area of this cube.
- A getVolume() method that returns the volume of this cube.
- Make sure to Javadoc EVERYTHING!
- Create a Sphere class that has everything that the cube class has except:
- Instead of lengthOfSide, Sphere has radius.
- Note that if you choose to copy and paste, nearly everything will change because of radius
- Make sure to Javadoc EVERYTHING! This means that if you copy and paste from Cube, there should be no mention of Cube or lengthOfSide in the javadoc.
- Create a third class named Program4 which has a main method. It should make use of Cube and Sphere to do the following:
- Create a Scanner named scanner off of standard input.
- Create a Cube named firstCube and use the default (no-argument) constructor to populate the reference
- Print out firstCube
- Prompt the user with "Please enter the length of the side for secondCube: "
- Read in the next integer and use it to set the length of the side of secondCube. DO NOT USE A CONSTRUCTOR TO SET THE LENGTH OF THE SIDE.
- Make new Cube named thirdCube with sides equal to 3. USE THE ARGUMENT CONSTRUCTOR TO DO THIS.
- Make a new integer named accessorValue and have it store the getter value for firstCube.
- Output "First Cube side length: ", "Second Cube surface area: ", and "Third Cube volume: " along with their values (use accessorValue for First Cube)
- Output all three Cubes in order along with the labels "First Cube: ", "Second Cube: ", and "Third Cube: " in the appropriate places. Then print out a new line
- Repeat steps 1-9 above for Spheres. All variable names, all prompts, and all outputs should make sense.
- Remember to Javadoc Program4 and the main method!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
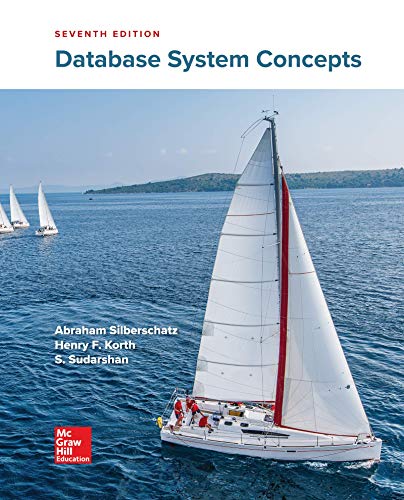
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
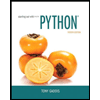
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
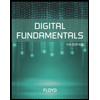
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
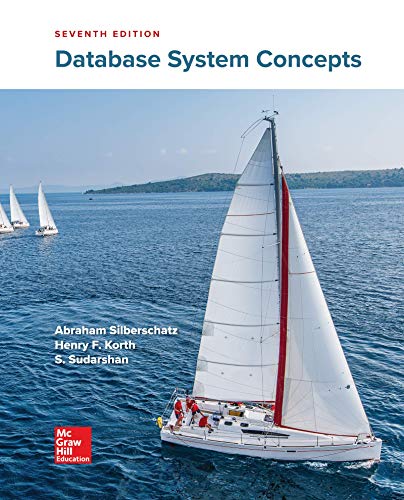
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
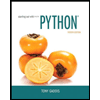
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
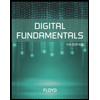
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
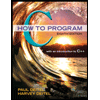
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
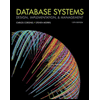
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
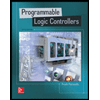
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education