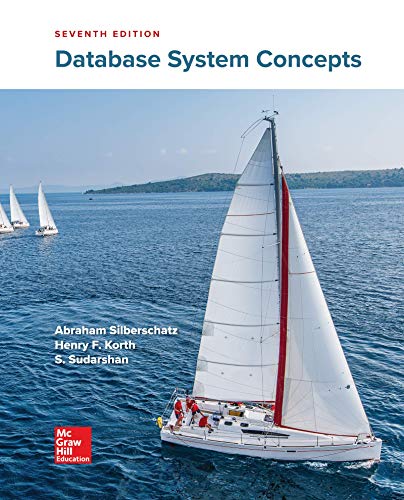
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
How do I create a class Chef, with the following instance attributes:
name: a string
record: a tuple of three positive integers (representing wins, losses, ties)
cuisine: a string ('French', 'Japanese', 'Chinese', etc.)
Also, how do I create a class Dish, with the following instance attributes:
name: a string
ingredients: a list of strings (the ingredients in the dish)
chef: a Chef object
ratings: a list of four zeroes
(The Dish class should also have the following instance method:
rate_dish: Sets the dish’s ratings to a list of four random positive integers from 1 to 10 (representing the four critic’s ratings for this dish).)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Complete the Car class by creating an attribute purchase_price (type int) and the method print_info() that outputs the car's information. Ex: If the input is: 2011 18000 2018 where 2011 is the car's model year, 18000 is the purchase price, and 2018 is the current year, then print_info() outputs: Car's information: Model year: 2011 Purchase price: $18000 Current value: $5770 Note: print_info() should use two spaces for indentation.arrow_forwardpublic class Plant { protected String plantName; protected String plantCost; public void setPlantName(String userPlantName) { plantName = userPlantName; } public String getPlantName() { return plantName; } public void setPlantCost(String userPlantCost) { plantCost = userPlantCost; } public String getPlantCost() { return plantCost; } public void printInfo() { System.out.println(" Plant name: " + plantName); System.out.println(" Cost: " + plantCost); }} public class Flower extends Plant { private boolean isAnnual; private String colorOfFlowers; public void setPlantType(boolean userIsAnnual) { isAnnual = userIsAnnual; } public boolean getPlantType(){ return isAnnual; } public void setColorOfFlowers(String userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; } public String getColorOfFlowers(){ return colorOfFlowers; } @Override public void printInfo(){…arrow_forwardclass Widget: """A class representing a simple Widget === Instance Attributes (the attributes of this class and their types) === name: the name of this Widget (str) cost: the cost of this Widget (int); cost >= 0 === Sample Usage (to help you understand how this class would be used) === >>> my_widget = Widget('Puzzle', 15) >>> my_widget.name 'Puzzle' >>> my_widget.cost 15 >>> my_widget.is_cheap() False >>> your_widget = Widget("Rubik's Cube", 6) >>> your_widget.name "Rubik's Cube" >>> your_widget.cost 6 >>> your_widget.is_cheap() True """ # Add your methods here if __name__ == '__main__': import doctest # Uncomment the line below if you prefer to test your examples with doctest # doctest.testmod()arrow_forward
- Create a class called Staff that has the following attributes: Staff String - FirstName String - LastName String - Department String - Title Integer - UIN Staff() Staff(FirstName, LastName, Department, Title, UIN) getFirstName() - String setFirstName(String) - void getLastName() - String setLastName(String) - void getDepartment() - String setDepartment(String) - void getTitle() - String setTitle(String) - void getUIN() - Integer setUIN(Integer) - void toString() - String compareTo(Staff) - Integer equals(Staff) - Boolean PreviousNextarrow_forwardConsider the following code: class Player { private: string ID; string name; public: Player(string n, string s) { name = n; setID(s); } string getName() const { return name; } string getID() const { return ID; } void setID(string s) { ID = s; } }; class BasketballPlayer : public Player { private: int fieldgoals; int attempts; public: BasketballPlayer(string n, string i, int fg, int a) : Player(n, i) { fieldgoals = fg; attempts = a; } // line 1 void printStats() const { cout << " Pct: " << (double) fieldgoals / attempts << endl; } }; int main() { Player golfer("Tiger Woods", "123456789"); BasketballPlayer pointGuard("Stephen Curry", "567890123", 2585, 5523); } Describe the code : Player(n, i) at the end of the BasketballPlayer constructor. a. calls the Player constructor with parameters n, i c. derives Player from BasketballPlayer d. derives BasketballPlayer from Player e. calls the Player…arrow_forwardDesign a class named Pet, which should have the following fields: • name. The name field holds the name of a pet. • animal. The animal field holds the type of animal that a pet is. Example values are “Dog”, “Cat”, and “Bird”. • age. The age field holds the pet’s age. The Pet class should also have the following methods: • setName. The setName method stores a value in the name field. • setAnimal. The setAnimal method stores a value in the animal field. • setAge. The setAge method stores a value in the age field. • getName. The getName method returns the value of the name field. • getAnimal. The getAnimal method returns the value of the animal field. • getAge. The getAge method returns the value of the age field. Write the Java code for the Pet class and demonstrate it.arrow_forward
- Create a class Person to represent a person according to the following requirements: A person has two attributes: id Add a constructer to initialize all the attributes to specific values. Add all setter and getter methods. Create a class Product to represent a product according to the following requirements: A product has four attributes: a reference number (can’t be changed) a price an owner (is a person) a shopName (is the same for all the products). Add a constructer without parameters to initialize all the attributes to default values (0 for numbers, "" for a string and null for object). Add a second constructer to initialize all the attributes to specific values. Use the keyword "this". Add the method changePrice that change the price of a product. The method must display an error message if the given price is negative. Add a static method changeShopName to change the shop name. Add all the getter methods. The method getOwner must return an owner. Create the class…arrow_forwardCan someone please help me with the main code? The code for the business class is down below. Part I - Design a class named Business that contains: A String attribute named name for the Business name A String attribute named symbol for the Business’s stock symbol A double attribute named lastPrice that stores the stock price one month ago A double attribute named currentPrice that stores the stock price for the current time A default constructor An overloaded constructor that creates a Business with a specified Business stock symbol and name An overloaded constructor with all attributes Accessors and mutators for each attribute A copy Constructor An Equals Method A toString() method to display current state of the object, formatted! Part 2- Create a BusinessDriver application. You will have at least 4 methods in this application main() – setBusinessData() - this reads the data from the file (call the file businessData.txt) displayBusinessData() - this displays the data to the…arrow_forwardDesign a Ship class that has the following members:• A field for the name of the ship (a string).• A field for the year that the ship was built (a string).• A constructor and appropriate accessors and mutators.• A toString method that displays the ship's name and the year it was built.Disign a CruiseShip class that extends the Ship class. The CruiseShip class should have thefollowing members:• A field for the maximum number of passengers (an int).• A constructor and appropriate accessors and mutators.• A toString method that overrides the toString method in the base class. The CruiseShipclass's toString method should display only the ship's name and the maximum number ofpassengers.Design a CargoShip class that extends the Ship class. The CargoShip class should have thefollowing members:• A field for the cargo capacity in tonnage (an int).• A constructor and appropriate accessors and mutators.• A toString method that overrides the toString method in the base class. The CargoShipclass's…arrow_forward
- ShoppingCart.java - Class definition ShoppingCartManager.java - Contains main() method Build the ShoppingCart class with the following specifications. Private fields String customerName - Initialized in default constructor to "none" String currentDate - Initialized in default constructor to "January 1, 2016" ArrayList cartItems Default constructor Parameterized constructor which takes the customer name and date as parameters Public member methods getCustomerName() accessor getDate() accessor addItem() Adds an item to cartItems array. Has a parameter of type ItemToPurchase. Does not return anything. removeItem() Removes item from cartItems array. Has a string (an item's name) parameter. Does not return anything. If item name cannot be found, output a message: Item not found in cart. Nothing removed. modifyItem() Modifies an item's description, price, and/or quantity. Has a parameter of type ItemToPurchase. Does not return anything. If item can be found (by name) in cart,…arrow_forwardDesign a class named CustomerRecord that holds a customer number, name, and address. Include separate methods to 'set' the value for each data field using the parameter being passed. Include separate methods to 'get' the value for each data field, i.e. "return" the field's value. Pseudocode:arrow_forwardclass IndexItem { public: virtual int count() = 0; virtual void display()= 0; };class Book : public IndexItem { private: string title; string author; public: Book(string title, string author): title(title), author(author){} virtual int count(){ return 1; } virtual void display(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };class Category: public IndexItem { private: /* fill in the private member variables for the Category class below */ ? int count; public: Category(string name, string code): name(name), code(code){} /* Implement the count function below. Consider the use of the function as depicted in main() */ ? /* Implement the add function which fills the category with contents below. Consider the use of the function as depicted in main() */ ? virtualvoiddisplay(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
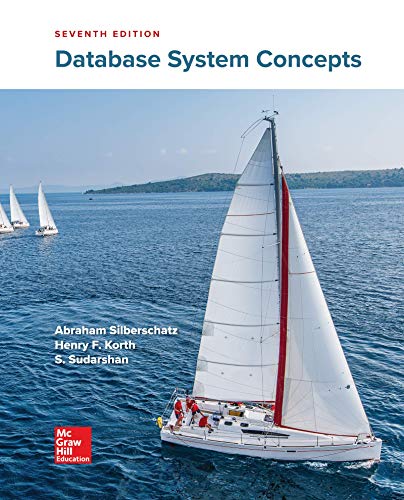
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
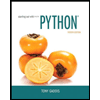
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
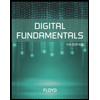
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
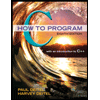
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
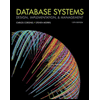
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
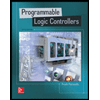
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education