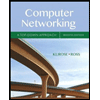
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
' make a hashmap '
import hashlib
import sys
def insert (hashmap, key, value):
' insert a key value pair into the hashmap '
hashmap[key] = value
def remove (hashmap, key):
' remove a key value pair from the hashmap '
del hashmap[key]
def search (hashmap, key):
' search for a key in the hashmap '
if key in hashmap:
return hashmap[key]
else:
return None
def print (hashmap):
' print the hashmap '
for key in hashmap:
print (key, ":", hashmap[key])
'end of print'
def main ():
'make a menu that lets the user call the functions'
hashmap = {}
while True:
print ("""
Menu:
1. Insert
2. Remove
3. Search
4. Print
5. Quit
""")
choice = input ("Enter your choice: ")
if choice == "1":
key = input ("Enter the key: ")
value = input ("Enter the value: ")
insert (hashmap, key, value)
elif choice == "2":
key = input ("Enter the key: ")
remove (hashmap, key)
elif choice == "3":
key = input ("Enter the key: ")
value = search (hashmap, key)
if value:
print ("Value:", value)
else:
print ("Key not found")
elif choice == "4":
print (hashmap)
elif choice == "5":
sys.exit ()
else:
print ("Invalid choice")
if __name__ == "__main__":
main ()
Fix the indentation errors. Im trying to make a python program for a hashmap but am having errors. The code is almost complete.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Similar questions
- a. Hash the following reindeer names, in this order based on the first letter of their name into a hash table of size 8 donner blitzen cupid dancer vixen prancer comet dasher b. this list has an issue called what?arrow_forwardA hash is most equivalent to a __________. encryption double encryption digest two way operationarrow_forwardIn C++ create a hash table that stores char.arrow_forward
- for (String name : likedBy) { String likedUser= name.trim(); Set<String> likes = likesMap.getOrDefault(likedUser, new HashSet<>()); Here you create a new Set for likes. This is in the iteration over the likers though so it results in many missing entries. [ How do i Fix this ] import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; import java.util.ArrayList; import java.util.HashMap; import java.util.HashSet; import java.util.List; import java.util.Map; import java.util.Set; public class FacebookLikeManager { private Map<String, Set<String>> likesMap; public FacebookLikeManager() { likesMap = new HashMap<>(); } public void buildMap(String filePath) { try (BufferedReader reader = new BufferedReader(new FileReader(filePath))) { String line = reader.readLine(); while (line != null) { String[]…arrow_forwardYou may have found it somewhat tedious and unpleasant to use the debugger and visualizer to verify the correctness of your addFirst and addLast methods. There is also the problem that such manual verification becomes stale as soon as you change your code. Imagine that you made some minor but uncertain change to addLast]. To verify that you didn't break anything you'd have to go back and do that whole process again. Yuck. What we really want are some automated tests. But unfortunately there's no easy way to verify correctness of addFirst and addLast] if those are the only two methods we've implemented. That is, there's currently no way to iterate over our list and get bad its values and see that they are correct. That's where the toList method comes in. When called, this method returns a List representation of the Deque. For example, if the Deque has had addLast (5) addLast (9) addLast (10), then addFirst (3) called on it, then the result of toList() should be a List with 3 at the…arrow_forwardJava - Given list: ( 4, 7, 18, 48, 49, 56, 66, 68, 79 )arrow_forward
- def upgrade_stations(threshold: int, num_bikes: int, stations: List["Station"]) -> int: """Modify each station in stations that has a capacity that is less than threshold by adding num_bikes to the capacity and bikes available counts. Modify each station at most once. Return the total number of bikes that were added to the bike share network. Precondition: num_bikes >= 0arrow_forwardThe compareTo method. First you compare this.ticker which is a String, then if they are the same then you compare by the this.purchasePrice which is a double hashCode public int hashCode() The hashCode method using the Code-> Generate equals and hashCode with no changes Overrides: hashCode in class Object Returns: int Representing the hashCode compareTo public int compareTo(Stock another) The compareTo method not auto generated Compares first by ticker and if the tickers are the same secondarily compares by price Specified by: compareTo" in interface Comparable<stock> Parameters: another - the object to be compared. Returns: int Representing order Throws: IllegalArgumentException"- if another is null.arrow_forward8.3.2: HashMap with enhanced for loop. Declare variable scoreToGrade as a HashMap with Character key type and Double value type, representing a grade and the minimum score of the grade. Read integer numToRead from input. Then read numToRead key-value pairs from input and insert each into scoreToGrade. If the key already exists, replace the key's existing value with the new value. Ex: If the input is: 3 D 60.0 A 89.0 A 90.5 then one possible output is: Key: D, Value: 60.0 Key: A, Value: 90.5 Note: The order of the keys is not guaranteed in a HashMap. import java.util.Scanner;import java.util.HashMap; public class GradeScale {public static void main(String[] args) {Scanner scnr = new Scanner(System.in);char assignedGrade;double minScore;int numToRead;int i;/* Any variable declarations go here */ /* Your code goes here */ // For each key in the HashMap, retrieve the value associated with the keyfor (Character key : scoreToGrade.keySet()) {System.out.println("Key: " + key + ",…arrow_forward
- create a script that prompts the user for a number. then create a loop to generate that number of random hash tables. then add these hash tables to an array. powershellarrow_forwardEstimated Completion Time: 2-3 minutes Which of the following situations will lead to an object being aliased? Select all that apply. n Assigning a list to L1, then assigning L1 to L2 n Assigning a string to S1, then assigning the result of slicing S1 (i.e. S1[:]) to S2 n Assigning a list to L1, then assigning the result of a copy() method on L1 to L2 n Assigning a list to L1, then assigning the result of slicing L1 (i.e., L1[:]) to L2 n Assigning a string to S1, then assigning the result of a replace() method on S1 to S2 n Assigning a list to L1, then using L1 in a function call with header "def foo(x: List) -> List" n Assigning a string to S1, then using S1 in a function call with header "def foo(x: str) -> None" n Assigning two lists to L1 and L2, then assigning the result of adding them to L3arrow_forward7- question What keys and values are contained in the following map after execution of the following piece of code? Map map = new HashMap (); map.put (8, "Eight"); map.put (41, "Forty-one"); map.put (8, "Ocho"); map.put (18, "Eighteen"); map.put (50, "Fifty"); map.put (132, "OneThreeTwo"); map.put (28, "Twenty-eight"); map.put (79, "Seventy-nine"); map.remove ( 41); map.remove (28); map.put (28, "18"); map.remove (18); a. {8=Eight, 41=Forty-one, 8=0cho, 18=Eighteen, 50=Fifty, 132=OneThreeTwo, 28=Twenty-eight, 79=Seventy-nine, 28=18} b. {8=Eight, 8=Ocho, 50=Fifty, 132=OneThreeTwo, 79=Seventy-nine, 28=18} c. {50=Fifty, 132=DOneThreeTwo, 8=Ocho, 28=18, 79=Seventy-nine} d. {8=Eight, 50=Fifty, 132=OneThreeTwo, 79=Seventy-nine, 28=18}arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
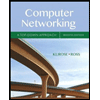
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
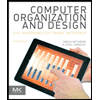
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
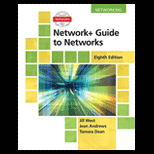
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
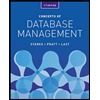
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
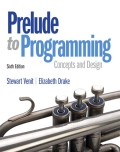
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
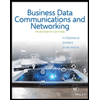
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY