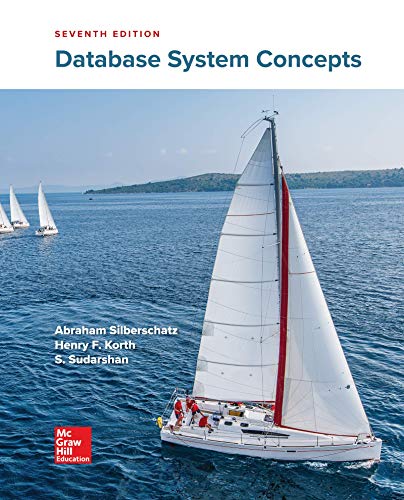
Make a code using Recursion
The countSubstring function will take two strings as parameters and will return an integer that is the count of how many times the substring (the second parameter) appears in the first string without overlapping with itself. This method will be case insensitive.
For example:
countSubstring(“catwoman loves cats”, “cat”) would return 2
countSubstring(“aaa nice”, “aa”) would return 1 because “aa” only appears once without overlapping itself.
public static int countSubstring(String s, String x) {
if (s.length() == 0 || x.length() == 0)
return 1;
if (s.length() == 1 || x.length() == 1){
if (s.substring(0,1).equals(x.substring(0,1))){
s.replaceFirst((x), " ");
return 1 + countSubstring(s.substring(1), x);
}
else {
return 0 + countSubstring(s.substring(1), x);
}
}
return countSubstring(s.substring(0,1), x) + countSubstring(s.substring(1), x);
}
public class Main {
public static void main(String[] args) {
System.out.println(Recursion.countSubstring("catwoman loves cats","cat"));
System.out.println(Recursion.countSubstring("aaa nice","aa"));
}
}

The source code of the program
#Main.java
class Main
{
public static void main(String[] args)
{
String testString3 = "catwoman loves cats";
String testString2 = "aaa nice";
System.out.println(Recursion.countSubstring(testString3, "cat"));
System.out.println(Recursion.countSubstring(testString2, "aa"));
}
}
#Recursion.java
class Recursion
{
public static int countSubstring(String s, String sub)
{
int subLength = sub.length();
int sLength = s.length();
if (sLength < subLength)
{
return 0;
}
else
{
String sLower = s.toLowerCase();
String subLower = sub.toLowerCase();
if (sLower.substring(0, subLength).equals(subLower))
{
return 1 + countSubstring(s.substring(subLength), sub);
}
else
{
return countSubstring(s.substring(1), sub);
}
}
}
}
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- 2arrow_forwardCalling a recursive function. Write a statement that calls the recursive function backwards_alphabet() with input starting_letter.Sample output with input: 'f' f e d c b a def backwards_alphabet(curr_letter): if curr_letter == 'a': print(curr_letter) else: print(curr_letter) prev_letter = chr(ord(curr_letter) - 1) backwards_alphabet(prev_letter) starting_letter = input() ''' Your solution goes here '''arrow_forwardPython Using recursion No loops Print asterisks If k > n, n is negative or zero, or indent is negative, the function does not print anything. It may use string multiplication and formatted strings such s * num for some string s and some integer num def printStars(k, n, indent):arrow_forward
- Write a program named stars.py that has two functions for generating star polygons. One function should be implemented using an iteration (a for loop), and another function should be a recursion. It is optional to fill in your shapes. The functions for generating stars should be named star (size, n, d=2) and star_recursive (size, n, level, d=2), where size is the size of the polygon side (edge), n is the number of sides (or angles), d is a density or winding number that should be set to default value 2, and level is the level of recursion initially equal to n.arrow_forwardHow to attempt? Question: Given a string, write a recursive function which will print one character of a string at one time by removing white spaces and the following special characters [#../?]. Example: Input: Hello User Output: Recursive call 1: r Recursive call 2: e Recursive call 3: s Recursive call 4: U Recursive call 5: o Recursive call 6: 1 Recursive call 7:1 Recursive call 8: e Recursive call 9: Harrow_forwardLab Goal : This lab was designed to teach you more about recursion. Lab Description : Take a string and remove all occurrences of the word chicken and count how many chickens were removed. Keep in mind that removing a chicken might show a previously hidden chicken. You may find substring and indexOf useful. achickchickenen - removing the 1st chicken would leave achicken behindachicken - removing the 2nd chicken would leave a behindSample Data : itatfunitatchickenfunchchickchickenenickenchickchickfunchickenbouncetheballchickenSample Output : 01302arrow_forward
- Part (1) Write a recursion method named CalculateSeries, that will receive one integer valuen as parameter. The method will calculate and return the term n in the following series: T(0)= 0 T(n)= 2 T(n-1) +1 Part (2) Write the main method to test your method. Enter n >> 5 T(5) = 31 %3Darrow_forwardThe following recursive method get Number Equal searches the array x of 'n integers for occurrences of the integer val. It returns the number of integers in x that are equal to val. For example, if x contains the 9 integers 1, 2, 4, 4, 5, 6, 7, 8, and 9, then getNumberEqual(x, 9, 4) returns the value 2 because 4 occurs twice in x. public static int getNumberEqual(int x[], int n, int val) { if (n< 0) ( return 0; } else { if (x[n-1) == val) { return getNumberEqual(x, n-1, val) +1; } else { return getNumber Equal(x, n-1, val); } // end if ) // end if } // end get Number Equal Demonstrate that this method is recursive by listing the criteria of a recursive solution and stating how the method meets each criterion.arrow_forwardJava, I am not displaying my results correct. It should add up the digits in the string. input string |result for the sumIt Recursion functions and a findMax function that finds the largest number in a string "1d2d3d" | 6 total "55" |10 total "xx" | 0 total "12x8" |12 Max number "012x88" |88 Max Number "012x88ttttt9xe33ppp100" |100 Max Number public class Finder { //Write two recursive functions, both of which will parse any length string that consists of digits and numbers. Both functions //should be…arrow_forward
- Given a long string use recursion to traverse the string and replace every vowel (A,E,I,O,U) with an @ sign. You cannot change the method header. public String encodeVowels(String s){ // Your code here }arrow_forwardConsider a network of streets laid out in a rectangular grid, In a northeast path from one point in the grid to another, one may walk only to the north (up) and to the east (right). Write a program that must use a recursive function to count the number of northeast paths from one point to another in a rectangular grid. Your program should prompt the user to input the numbers of points to the north and to east respectively, and then output the total number of paths. Notes: 1. Here is a file (timer.h download and timer.cpp download) which should be included in your program to measure time in Window or Unix (includes Mac OS and Linux) systems (use start() for the beginning of the algorithm, stop() for the ending, and show() for printing). 2. The computing times of this algorithm is very high, and the number of paths may be overflow, don't try input numbers even over 16. 3. Name your recursive function prototype as calcPath(int north, int east) to ease grading. 4. Paste your outputarrow_forwardUsing recursion, write a function sum that takes a single argument n and computes the sum of all integers between 0 and n inclusive. Do not write this function using a while or for loop. Assume n is non-negative. def sum(n): """Using recursion, computes the sum of all integers between 1 and n, inclusive. Assume n is positive. >>> sum(1) 1 >>> sum(5) # 1 + 2 + 3+ 4+ 5 15 "*** YOUR CODE HERE ***"arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
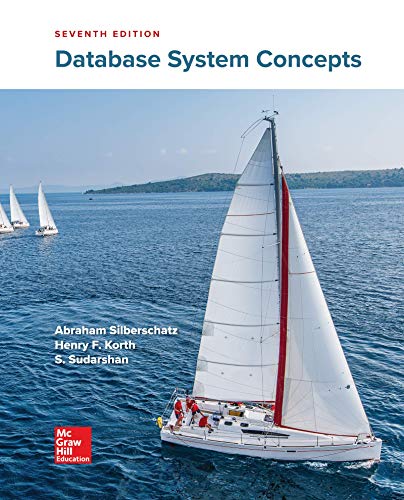
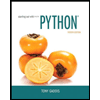
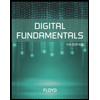
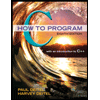
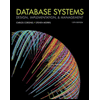
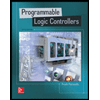