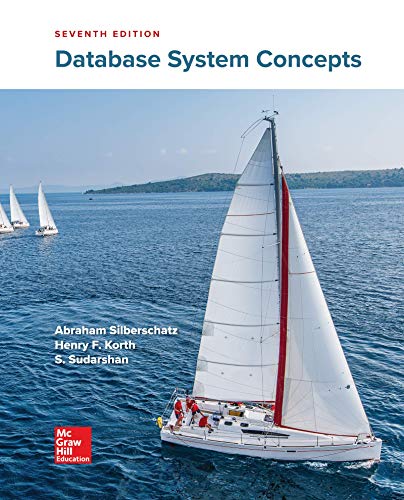
explain the functionality used in the below program. explain all the functions and algorithem used in this program.
#include<iostream>
#include<string.h>
using namespace std;
#define ROW 5
#define COL 5
// A function to check if a given
// cell (row, col) can be included in DFS
int isSafe(int M[][COL], int row, int col,
bool visited[][COL])
{
// row number is in range, column
// number is in range and value is 1
// and not yet visited
return (row >= 0) && (row < ROW) && (col >= 0) && (col < COL) && (M[row][col] &&
!visited[row][col]);
}
// A utility function to do DFS for a
// 2D boolean matrix. It only considers
// the 8 neighbours as adjacent vertices
void DFS(int M[][COL], int row, int col,
bool visited[][COL])
{
// These arrays are used to get
// row and column numbers of 8
// neighbours of a given cell
static int rowNbr[] = { -1, -1, -1, 0, 0, 1, 1, 1 };
static int colNbr[] = { -1, 0, 1, -1, 1, -1, 0, 1 };
// Mark this cell as visited
visited[row][col] = true;
// Recur for all connected neighbours
for (int k = 0; k < 8; ++k)
if (isSafe(M, row + rowNbr[k], col + colNbr[k], visited))
DFS(M, row + rowNbr[k], col + colNbr[k], visited);
}
// The main function that returns
// count of islands in a given boolean
// 2D matrix
int countIslands(int M[][COL])
{
// Make a bool array to mark visited cells.
// Initially all cells are unvisited
bool visited[ROW][COL];
memset(visited, 0, sizeof(visited));
// Initialize count as 0 and
// travese through the all cells of
// given matrix
int count = 0;
for (int i = 0; i < ROW; ++i)
for (int j = 0; j < COL; ++j)
// If a cell with value 1 is not
if (M[i][j] && !visited[i][j]) {
// visited yet, then new island found
// Visit all cells in this island.
DFS(M, i, j, visited);
// and increment island count
++count;
}
return count;
}
// Driver code
int main()
{
int M[][COL] = { { 1, 1, 0, 0, 0 },
{ 0, 1, 0, 0, 1 },
{ 1, 0, 0, 1, 1 },
{ 0, 0, 0, 0, 0 },
{ 1, 0, 1, 1, 0 } };
cout << "Number of islands is: " << countIslands(M);
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- In C++ Assume that a 5 element array of type string named boroughs has been declared and initialized. Write the code necessary to switch (exchange) the values of the first and last elements of the array.arrow_forwardProbelm part 1: Define a function called common_divisors that takes two positive integers m and n as its arguments and returns the list of all common divisors (including 1) of the two integers, unless 1 is the only common divisor. If 1 is the only common divisor, the function should print a statement indicating the two numbers are relatively prime. Otherwise, the function prints the number of common divisors and the list of common divisors. Complete each to do with comments to expalin your process. Problem part 2: complete the following todo's and make comments to explain each step # TODO: Define the function 'common_divisors' with two arguments 'm' and 'n'. # TODO: Start with an empty list and append common divisors to the list. # TODO: Create a conditional statement to print the appropriate statement as indicated above. Problem part 3: Call the function common_divisors by passing 5 and 38. Your output should look like this: 5 and 38 are relatively prime. # TODO: Call…arrow_forwardii)Detail the four abstraction layers that make up TCP/IP. ii) Provide a scenario in which two computers share a video file via an unencrypted ftp connection using a weak TCP three-way handshake.arrow_forward
- ## Given the stubs for the following function ## and the main program, complete the implementation.## Finish the 3 assert statements to properly## assert the result shown below (be careful ## with the types of the variables).def get_list_avg(________________): """ Given a list of numerical values in STRINGS, iterate over these elements until a negative value is found. Return the average of the positive numbers in these strings that are in the list that came **before** the first negative element. If the list is empty, return -1. For example, if the list is this: ['10', '1', '6', '3', '-1', '5', '9'] then the function returns (10 + 1 + 6 + 3) / 4, which is 5.0 """ passif __name__ == "__main__": ### Write 3 assert statements ### to test the function assert ...arrow_forwardstruct employee{int ID;char name[30];int age;float salary;}; (A) Using the given structure, Help me with a C program that asks for ten employees’ name, ID, age and salary from the user. Then, it writes the data in a file named out.txt (B) For the same structure, read the contents of the file out.txt and print the name of the highest salaried employee and youngest employee names name in the outputscreen.arrow_forwardC++ This a question to create a C string function. Create a function strfind(cs, c) that returns the index of the first occurrence of character c in C string cs. In case c is not found, −1 is returned. Implement the function as efficiently as possible.arrow_forward
- C++ Programing D.S.Malik 16-13\ This chapter defined and identified various operations on a circular linked list. Use the definitions of the class circularLinkedList and its member functions. (You may assume that the elements of the circular linked list are in ascending order.) In the main.cpp file, write a program to test various operations of the class defined in the step above. Your program should accept a sorted list as input and output the following: The length of the list. The list after deleting a number. A message indicating if a number is contained in the list.arrow_forwardFunction 3: Positive Unique Numbers _missingSpace(string) Create a JavaScript Arrow function that meets the following requirements: • Authored using arrow expression syntax (constant name _missingSpace) • The function is passed a string argument • The function inserts a white space between every instance of a lowercase character followed immediately by an uppercase character, and returns the modified string, with whitespaces, back to the caller. • Console log output is NOT permitted.arrow_forwardgetting error please helparrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
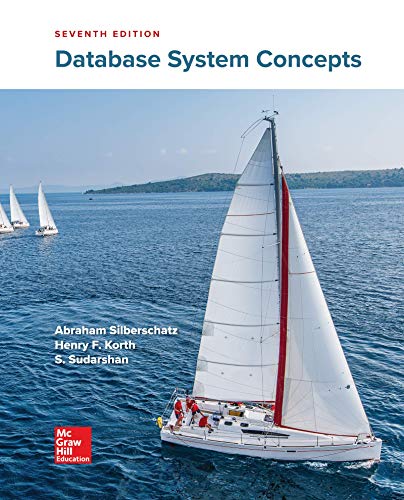
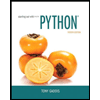
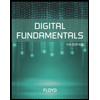
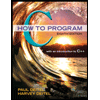
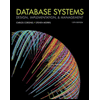
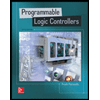