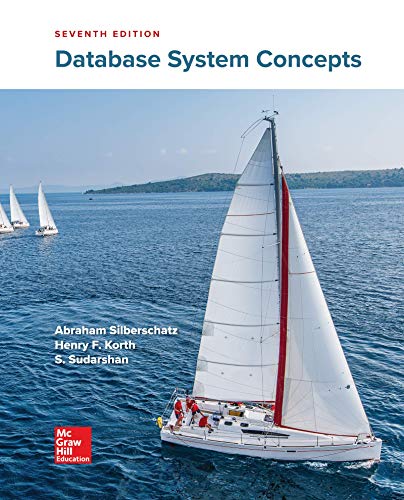
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
![For the sorting Choice, do the following:
Right after your header file and using namespace std;
int sortingChoice;
// Declare the functions
void SelectionSort(item Type A[], int N);
int Min(item Type A[], int i, int N);
void InsertionSort(item Type A[], int N);
void BubbleSort(item Type A[], int N);
In the main:
a)
Declare the array dynamically (this has been discussed in the class) using pointer
b) Allocate memory to N number of data
c)
Get user inputs (do not initialize the array) using cin statement to fill up the array for all N elements
d)
e)
Get the value for sortingChoice by properly prompting the user
Write a switch statement using sorting Choice as controlling parameter, and call SelectionSort for case 1,
InsertionSort for case 2, BubbleSort for case 3, and default case display an error message saying that the choice is
wrong.
Outside the switch statement, display the sorted array with proper title.
If you have forgotten how to use switch statement, use the following structure
switch(sorting Choice)
case 1:
SelectionSort(A, N);
{
break;
case 2:
InsertionSort(A, N);
break;
and so on
default:
cout << "Not a correct choice" << endl;
}
Then display the sorted array.](https://content.bartleby.com/qna-images/question/f8fa7aa4-dafc-4c07-b274-462aa6ff800c/89530d4d-2167-478d-9012-03c974eb266b/58ejdlt_thumbnail.png)
Transcribed Image Text:For the sorting Choice, do the following:
Right after your header file and using namespace std;
int sortingChoice;
// Declare the functions
void SelectionSort(item Type A[], int N);
int Min(item Type A[], int i, int N);
void InsertionSort(item Type A[], int N);
void BubbleSort(item Type A[], int N);
In the main:
a)
Declare the array dynamically (this has been discussed in the class) using pointer
b) Allocate memory to N number of data
c)
Get user inputs (do not initialize the array) using cin statement to fill up the array for all N elements
d)
e)
Get the value for sortingChoice by properly prompting the user
Write a switch statement using sorting Choice as controlling parameter, and call SelectionSort for case 1,
InsertionSort for case 2, BubbleSort for case 3, and default case display an error message saying that the choice is
wrong.
Outside the switch statement, display the sorted array with proper title.
If you have forgotten how to use switch statement, use the following structure
switch(sorting Choice)
case 1:
SelectionSort(A, N);
{
break;
case 2:
InsertionSort(A, N);
break;
and so on
default:
cout << "Not a correct choice" << endl;
}
Then display the sorted array.
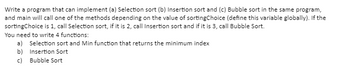
Transcribed Image Text:Write a program that can implement (a) Selection sort (b) Insertion sort and (c) Bubble sort in the same program,
and main will call one of the methods depending on the value of sortingChoice (define this variable globally). If the
sortingChoice is 1, call Selection sort, if it is 2, call Insertion sort and if it is 3, call Bubble Sort.
You need to write 4 functions:
a) Selection sort and Min function that returns the minimum index
b)
Insertion Sort
c) Bubble Sort
Expert Solution

arrow_forward
Step 1
Algorithm:
1. Begin.
2. Declare sortingChoice, N, and A (an array).
3. Prompt the user to enter the number of elements and store it in N.
4. Prompt the user to enter the elements of the array and store it in A.
5. Prompt the user to enter the sorting choice.
6. Compare the sorting choice
6a. If sortingChoice is 1, call SelectionSort().
6b. If sortingChoice is 2, call InsertionSort().
6c. If sortingChoice is 3, call BubbleSort().
6d. Otherwise, display an error message.
7. After the sorting, display the sorted array.
8. Free the memory allocated for A.
9. End.
Step by stepSolved in 8 steps with 12 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- This program will display an array being passed to a function, show. The show function will display the elements of the given array. The main program controls the size and the inputs of an array. // function prototype void show(int [], int); int main(){ const int arraysize=5; int numbers[arraysize]={3,6,12,7,5}; show(numbers,arraysize); // (A) show(numbers,arraysize+1); return 0;} //function body void show(int nums[], int size){ for (int index=0;index<size; index++) cout<<nums[index]<<endl; } What is the output of this program? What is the output if modification (A) has been made?arrow_forward1- Write a user-defined function that accepts an array of integers. The function should generate the percentage each value in the array is of the total of all array values. Store the % value in another array. That array should also be declared as a formal parameter of the function. 2- In the main function, create a prompt that asks the user for inputs to the array. Ask the user to enter up to 20 values, and type -1 when done. (-1 is the sentinel value). It marks the end of the array. A user can put in any number of variables up to 20 (20 is the size of the array, it could be partially filled). 3- Display a table like the following example, showing each data value and what percentage each value is of the total of all array values. Do this by invoking the function in part 1.arrow_forward# dates and times with lubridateinstall.packages("nycflights13") library(tidyverse)library(lubridate)library(nycflights13) Qustion: Create a function called date_quarter that accepts any vector of dates as its input and then returns the corresponding quarter for each date Examples: “2019-01-01” should return “Q1” “2011-05-23” should return “Q2” “1978-09-30” should return “Q3” Etc. Use the flight's data set from the nycflights13 package to test your function by creating a new column called quarter using mutate()arrow_forward
- Please complete the following guidelines and hints. Using C language. Please use this template: #include <stdio.h>#define MAX 100struct cg { // structure to hold x and y coordinates and massfloat x, y, mass;}masses[MAX];int readin(void){/* Write this function to read in the datainto the array massesnote that this function should return the number ofmasses read in from the file */}void computecg(int n_masses){/* Write this function to compute the C of Gand print the result */}int main(void){int number;if((number = readin()) > 0)computecg(number);return 0;}Testing your workTypical Input from keyboard:40 0 10 1 11 0 11 1 1Typical Output to screen:CoG coordinates are: x = 0.50 y = 0.50arrow_forwardgetting error please helparrow_forwardUsing C++ Language Write a function call with arguments tensPlace, onesPlace, and userInt. Be sure to pass the first two arguments as pointers. Sample output for the given program: tensPlace = 4, onesPlace = 1 Code: #include <stdio.h> void SplitIntoTensOnes(int* tensDigit, int* onesDigit, int DecVal){ *tensDigit = (DecVal / 10) % 10; *onesDigit = DecVal % 10;} int main(void) { int tensPlace; int onesPlace; int userInt; scanf("%d", &userInt); /* Your solution goes here */ printf("tensPlace = %d, onesPlace = %d\n", tensPlace, onesPlace); return 0;}arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
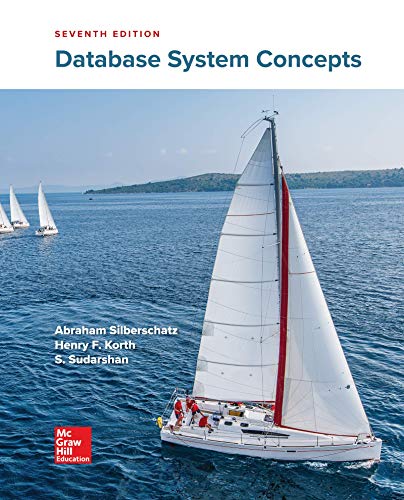
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
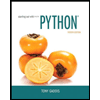
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
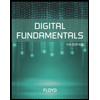
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
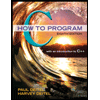
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
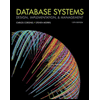
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
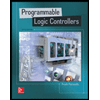
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education