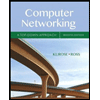
C++ language
Alter the code found in main.cpp
Using the file, add/change the code in the file, but only where indicating you can add or change code.
main.cpp
#include <iostream>
using namespace std;
/*
The function binarySearch accepts a sorted array data with no duplicates,
and the range within that array to search, defined by first and last.
Finally, goal is the value that is searched for within the array.
If the goal can be found within the array, the function returns the
index position of the goal value in the array. If the goal value does
not exist in the array, the function returns -1.
*/
int binarySearch(int data[], int first, int last, int goal)
{
cout << "first: " << first << ", last: " << last << endl;
// YOU CAN ONLY ADD OR CHANGE CODE BELOW THIS COMMENT
return -1;
// YOU CAN ONLY ADD OR CHANGE CODE ABOVE THIS COMMENT
}
int main()
{
const int ARRAY_SIZE = 20;
int searchValue;
/* generates an array data that contains:
0, 10, 20, 30, .... 170, 180, 190
*/
int data[ARRAY_SIZE];
for(int i = 0; i < ARRAY_SIZE; i++)
data[i] = i * 10;
cout << "Enter Search Value: ";
cin >> searchValue;
int answer = binarySearch(data, 0, ARRAY_SIZE-1, searchValue);
cout << "Answer: " << answer << endl;
return 0;
Create a function binarySearch that accepts 4 inputs:
- data[] - A sorted (low to high) array of integers with no duplicates.
- first - the smallest index position within the array that should be searched.
- last - the largest index position within the array that should be searched.
- goal - the value we are searching for within the array.
The function will return the index position of the value goal in the array. If the value goal does not exist in the array, the function returns -1. You must use recursion to solve this problem efficiently.
Sample Output:
Enter Search Value:
165
first: 0, last: 19
first: 10, last: 19
first: 15, last: 19
first: 15, last: 16
first: 16, last: 16
first: 17, last: 16
Answer: -1
[cathy]$ ./a.out
Enter Search Value: 40
first: 0, last: 19
first: 0, last: 8
Answer: 4
[cathy]$ ./a.out
Enter Search Value: 10
first: 0, last: 19
first: 0, last: 8
first: 0, last: 3
Answer: 1

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Code in Perl Create an array which holds a list of Video Games, consisting of title, the year it was released (guess if you don’t know) , platform (NES, XBOX One, etc) and publisher (in that order). Add at least ten albums to the list. Then, use the split function and a for or foreach loop to display the publisher followed by the title and platform for each list element.arrow_forward/)9. Given an array of integers, write a PHP function to find the maximum element in the array. PHP Function Signature: phpCopy code function findMaxElement($arr) { // Your code here } Example: Input: [10, 4, 56, 32, 7] Output: 56 You can now implement the findMaxElement function to solve this problem. Keep it and.arrow_forward// MichiganCities.cpp - This program prints a message for invalid cities in Michigan. // Input: Interactive // Output: Error message or nothing #include <iostream> #include <string> using namespace std; int main() { // Declare variables string inCity; // name of city to look up in array const int NUM_CITIES = 10; // Initialized array of cities string citiesInMichigan[] = {"Acme", "Albion", "Detroit", "Watervliet", "Coloma", "Saginaw", "Richland", "Glenn", "Midland", "Brooklyn"}; bool foundIt = false; // Flag variable int x; // Loop control variable // Get user input cout << "Enter name of city: "; cin >> inCity; // Write your loop here // Write your test statement here to see if there is // a match. Set the flag to true if city is found. // Test to see if city was not found to determine if // "Not a city in Michigan" message should be printed.…arrow_forward
- C++ Lexicographical Sorting Given a file of unsorted words with mixed case: read the entries in the file and sort those words lexicographically. The program should then prompt the user for an index, and display the word at that index. Since you must store the entire list in an array, you will need to know the length. The "List of 1000 Mixed Case Words" contains 1000 words. You are guaranteed that the words in the array are unique, so you don't have to worry about the order of, say, "bat" and "Bat." For example, if the array contains ten words and the contents are cat Rat bat Mat SAT Vat Hat pat TAT eat after sorting, the word at index 6 is Rat You are encouraged to use this data to test your program.arrow_forwardRead input integer n from the user and then write a recursive program in C language to print natural numbers from 1 to n in the output.arrow_forwardHelp now please Pythonarrow_forward
- Arrays write a C++ program that will declare two static arrays where each will hold the contents of a file (random.txt) of 200 integer values. Sorting Using the two arrays, call a private member function that uses the bubble sort algorithm to sort one of the arrays in ascending order. The function should count the number of exchanges it makes. Display this value. The program should then call a private member function that uses the selection sort algorithm to sort the other array. It should also count the number of exchanges it makes. Display this value. Searching Next, call a private member function that uses the linear search program to locate the value 869. The function should keep a count of the number of comparisons it makes until it finds the value. Display this value The program then should call a private member function that uses the binary search algorithm to locate the same value. It should also keep count of the numbers of comparisons it makes. Display this…arrow_forwardC++ Programming: How would you write a function that takes a filename and an array of struct time, opens a file, reads each line in the file as the number of days, converts this to a struct time and stores this is in an array? Here is my code so far (p.s. the function i need help with is called void readData): #include<iostream>#include<string>#include<fstream>#include<cstdlib>#include<vector> using namespace std; struct time{// private:int years, months, days;//public: time(){}time(int days){}}; time getYearsMonthsDays(int days){time x;x.years = days/450;days = days%450;x.months = days/30;x.days = days%30;return x;} int getTotalDays(time x){int totalDays;totalDays = x.years*450 + x.months*30 + x.days;return totalDays;} void openofile(string ofilename,ofstream &fout){fout.open(ofilename.c_str());if(!fout){cout << "Error opening output file..." << endl;exit(1);}} void openifile(string ifilename,ifstream…arrow_forwardAssume the following main module is in a program that includes the binarysearch function that was shown in a chapter. Why doesn't the pseudocode in the main module work?arrow_forward
- See attached images. Please help - C++arrow_forward1. Display Function Implement a function to display the contents of the patient_list array. Add code to call this function. Note that there are multiple places where this function needs to be called. Look for the // TODO comments to find the correct locations. 2. Sorting the array by Age Implement the code to sort the contents of the patient_list array based on the value stored in the age field. To do this you will need to implement code that relies on the qsort function from the C Standard library A function that compares two patient elements, based on the value stored in the age field. A call to the qsort function, which includes the array to be sorted, the number of elements in the array, the size of each array element and the function used to compare the array elements. Add code to call the qsort function, using the age comparison function that you implemented. This code should be placed just under the appropriate // TODO comment in main(). Sorting the array by…arrow_forwarduse c++ Programming language Write a program that creates a two dimensional array initialized with test data. Use any data type you wish . The program should have following functions: .getAverage: This function should accept a two dimensional array as its argument and return the average of each row (each student have their average) and each column (class test average) all the values in the array. .getRowTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The function should return the total of the values in the specified row. .getColumnTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The function should return the total of the values in the specified column. .getHighestInRow: This function should accept a two…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
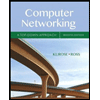
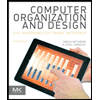
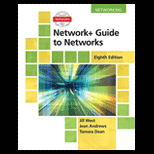
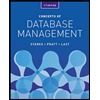
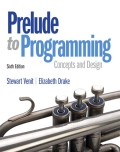
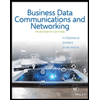