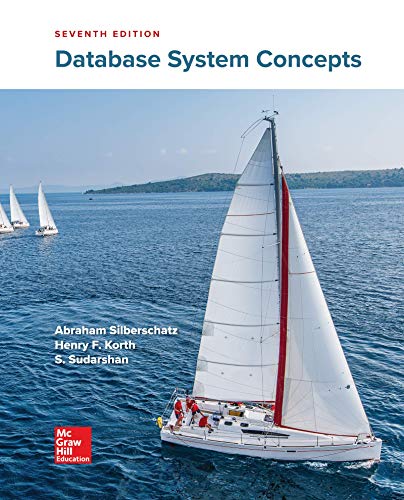
Write a simple airline ticket reservation
You should implement and use linked list class (the option of using a list class from STL is acceptable, though discouraged)
You will turn in
- a short written report containing:
- A description of the significant choices/issues in the design of your code.
- Listing of your experiments, showcasing the capabilities of the program.
- The source-code of your program.
You will turn in
- a short written report containing:
- A description of the significant choices/issues in the design of your code.
- Listing of your experiments, showcasing the capabilities of the program.
- The source-code of your program.

Trending nowThis is a popular solution!
Step by stepSolved in 10 steps

- This task is to develop a software for a bookshop that handles the purchases through online. When the customer places order to buy a book, the shop will search for the specific item uponarrow_forwardWe need a linked list to hold information about penguins in a zoo. You will need the following integers for your IntNode (see below). I have included sample values for one of the penguins: penguin ID: 45821 penguin weight (kg): 11 penguin height (cm): 90 We now need to track the number of penguins in the zoo. I would like to propose a better way to track the size of the list. Rather than traversing the list every time you need to know its size, why not keep a variable called listSize that increments every time you add a node to the list and decrements anytime you remove an item from the list? public class penguinList { //nested class IntNode goes here private IntNode first; private int listSize; //...the methods of penguinList class go here } Now, you just need to increment the listSize instance variable in every method that adds a node to the list. If you have any methods that removes a node from the list, decrement the listSize instance variable in those methods instead.…arrow_forwardJAVA please Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Code provided in the assignment ItemNode.java:arrow_forward
- We need a linked list to hold information about penguins in a zoo. You will need the following integers for your IntNode (see below). I have included sample values for one of the penguins: penguin ID: 45821 penguin weight (kg): 11 penguin height (cm): 90 We now need to track the number of penguins in the zoo. I would like to propose a better way to track the size of the list. Rather than traversing the list every time you need to know its size, why not keep a variable called listSize that increments every time you add a node to the list and decrements anytime you remove an item from the list? public class penguinList { //nested class IntNode goes here private IntNode first; private int listSize; //...the methods of penguinList class go here } Now, you just need to increment the listSize instance variable in every method that adds a node to the list. If you have any methods that removes a node from the list, decrement the listSize instance variable in those methods instead.…arrow_forwardInstruction: To test the Linked List class, create a new Java class with the main method, generate Linked List using Integer and check whether all methods do what they’re supposed to do. A sample Java class with main method is provided below including output generated. If you encounter errors, note them and try to correct the codes. Post the changes in your code, if any. Additional Instruction: Linked List is a part of the Collection framework present in java.util package, however, to be able to check the complexity of Linked List operations, we can recode the data structure based on Java Documentation https://docs.oracle.com/javase/8/docs/api/java/util/LinkedList.html package com.linkedlist; public class linkedListTester { public static void main(String[] args) { ListI<Integer> list = new LinkedList<Integer>(); int n=10; for(int i=0;i<n;i++) { list.addFirst(i); } for(int…arrow_forwardGiven the interface of the Linked-List struct Node{ int data; Node* next = nullptr; }; class LinkedList{ private: Node* head; Node* tail; public: display_at(int pos) const; ... }; Write a definition for a method display_at. The method takes as a parameter integer that indicates the node's position which data you need to display. Example: list: 5 -> 8 -> 3 -> 10 display_at(1); // will display 5 display_at(4); // will display 10 void LinkedList::display_at(int pos) const{ // your code will go here }arrow_forward
- Given the source code of linked List, answer the below questions(image): A. Fill out the method printList that print all the values of the linkedList: Draw the linked list. public void printList() { } // End of print method B. Write the lines to insert 10 at the end of the linked list. You must draw the final linked List. Notice that you can’t use second or third nodes. Feel free to define a new node. Assume you have only a head node C. Write the lines to delete node 2. You must draw the final linked list. Notice that you can’t use second or third node. Feel free to define a new node. Assume you have only a head nodearrow_forwardThis is using Data Structures in Javaarrow_forwardsee the image and answer the 2 questions. Thanksarrow_forward
- Given the following program segment. Assume the node is in the usual info-link form with the info of the type int. (list and ptr are reference variable of the LinkedListNode type. What is the output of this program? list = new LinkedListNode (); list.info = 20; ptr = new LinkedListNode (); ptr.info = 28; ptr.link= null; list.link ptr; ptr = list; list = new LinkedListNode (); list.info = 55; list.link = ptr; ptr = new LinkedListNode (); ptr.info = 30; ptr.link= list; list = ptr; ptr = new LinkedListNode (); ptr.info = 42; ptr.link= list.link; list.link= ptr; ptr = list; while (ptr!= null) { } System.out.println (ptr.info); ptr = ptr.link;arrow_forwardTrue or False For each statement below, indicate whether you think it is True or False. If you like, you can provide a description of your answer for partial credit in case you are incorrect. Use the standard linked list below to answer True/False statements 9-12: 8 7 null 4 10 The “head” pointer of this list is pointing to Node 4 If we called “insert(5)”, the new node’s “next” pointer will point to Node 8 If we called “delete(10)”, Node 7’s “next” pointer will point to Node 8 If we called “search(20)”, the “head” pointer will be at Node 4 after the search function endsarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
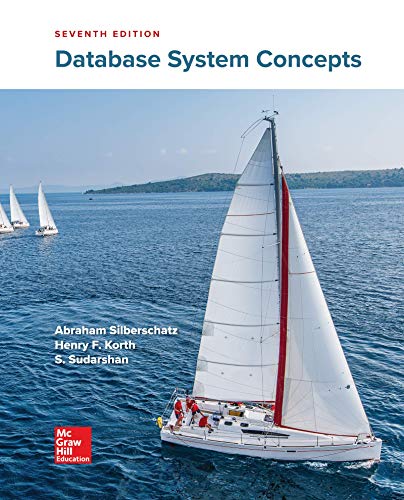
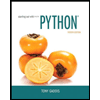
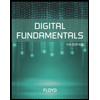
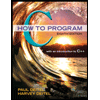
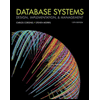
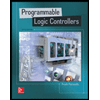