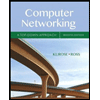
Given the following code, I need help correcting it to follow these instructions using python
(the csv file contains the following info...)
Jan | 14317 |
Feb | 3903 |
Mar | 1073 |
Apr | 3463 |
May | 2429 |
Jun | 4324 |
Jul | 9762 |
Aug | 15578 |
Sep | 2437 |
Oct | 6735 |
Nov | 88 |
Dec | 2497 |
#import the csv module for read-write
import csv
#file name for csv
FILE_NAME = "monthly_sales.csv"
#Show title of editor
def display_title():
print("FirstName LastName's Monthly Sales")
#space
print()
#show user menu display
def display_menu():
print("Command Menu\n\n Monthly - View monthly sales\n yearly - View yearly summary\n edit - Edit sales for a month\n exit - Exit program")
"""def read_sales():"""
#main function
def main():
sales = []
#write to the csv file
with open(FILE_NAME, "r", newline="") as file:
reader = csv.reader(file)
for row in reader:
sales.append(row)
#print out the display title and menu and then give user options to choose from
while True:
#show menu
display_title()
display_menu()
command = input("Command: ")
#begin of if loop
if command == "monthly":
view_monthly_sales(sales)
#show yearly
elif command == "yearly":
view_yearly_summary(sales)
#allow to edit
elif command == "edit":
edit(sales)
#exit program
elif command == "exit":
break
#give user error if invalid input is entered
else:
print("Not a valid command. Please try again.\n")
#allow user to know program is ended
print("Bye!")
view_monthly_sales(sales)
for row in sales:
print(f"{row[0]} - {row[1]}")
print()
view_yearly_summary(sales)
total = 0
for row in sales:
amount = int(row[1])
total += amount
# get count
count = len(sales)
# calculate average
average = total / count
average = round(average, 2)
# format and display the result
print("Yearly total: ", total)
print("Monthly average: ", average)
print()
#end of program
if __name__ == "__main__":
main()
![• Define a display_title() function and have it
• use the print() to show the text: [FirstName] [LastName]'s Monthly Sales
▪ Replace the placeholders with your own first and last name
• add a space using the print()
• Define a display_menu() function and have it
• Use the print() function several times to show the following text with a space
after:
COMMAND MENU
monthly - View monthly sales
View yearly summary
- Edit sales for a month
- Exit program
yearly
edit
exit
Step 2
• Define a main() function
• Call the display_title() function and the display_menu() function created in step 1.
• Test the program to see if the functions work as expected. You should see the
title and the command menu
• Create a new variable named sales and assign it the value of read_sales() function.
• Define a new read_sales() function
▪ Assign sales to an empty array
sales = []
▪ Open the csv file, read the file, and close the file.
with open(FILENAME, newline="") as file:
reader = csv.reader (file)
for row in reader:
sales.append(row)
Step 3
. Back in the main method
• Create a while loop that executes until the user types exit.
• Inside the loop, store the user's input in a variable called "command"
• Next, create an if, elif, elif,... for each of the command menu items
while True:
command=input("Command: ")
if command == "monthly":
view_monthly_sales (sales)
elif command == "yearly":
.
view_yearly_summary(sales)
elif command == "edit":
edit (sales)
elif command == "exit":
break
else:
print("Bye!")
print("Not a valid command. Please try again.\n")
• HINT: Notice the functions are not yet created. We will do this in the next few
steps.
• Add the following at the bottom of your code:
if __name__ == "_
"__main__": main()
Step 4
• Create a view_monthly_sales(sales) function that receives the sales as a parameter.
In the view_monthly_sales(sales) function, create a for loop that loops through all
items.
for row in sales:
print (f"{row[0]} - {row[1])")
• Add a space after using the print() function](https://content.bartleby.com/qna-images/question/3c348cba-a63b-4ada-8013-6981e5c617ad/da04fe01-84ee-4faf-ba45-02fe9a620e34/gte82uy_thumbnail.png)
![Step 5
• Create a view_yearly_summary(sales) function that receives the sales as a parameter
• Create a total variable and set it equal to 0.
• Create a for loop that loops through each row in the sales.
• Create an amount variable and assign it to the integer value in row 2 (row[1]).
• Add to the total the amount received in that row (total += amount)
• Get the count and store it in a variable named "count" (count = len(sales)).
• Create an average variable and assign it to the total / count (average = total /
count)
• Round the average to two decimal places.
• Display the yearly total and the monthly average
• Add a space below the results.
for row in sales:
amount = int(row[1])
total
amount
# get count
count len(sales)
=
# calculate average
total / count
average
average = round(average, 2)
# format and display the result
print("Yearly total:
", total)
print("Monthly average: ", average)
print ()
Step 6
• Create an edit(sales) function that receives the sales as a parameter
.
• Create a new variable named "names" in the new edit function.
• Assign it a list of abbreviated months (names = ['Jan', 'Feb, 'Mar', ... ])
• Create another variable named "name" and assign it the user's input for the
month.
• Make sure it is not case sensitive (name = name.title())
• If the input DOES NOT exist in the names list, display "Invalid three-letter month."
• If it does exist, move to step 6.
Step 7
• Create a variable named "index" and assign it the value of index of the month
entered (index = names.index(name))
Create a variable in the if statement named "amount" and assign it the value of an
integer from the user's input (amount = int(input("Sales Amount: "))
Create a "month" variable and assign it an empty list (month = [])
• Append the name to the list as well as the amount
month.append(name)
month.append(str(amount))
• Add the month and amount to the sales list at the correct index (sales[index] =
month)
• Call the method write_sales(sales) that we will create in step 7.
• Show the text "Sales amount for [month [0]} was modified."
• Add a space using the print() function.
Step 8
• Create the write_sales(sales) function and have sales as a parameter.
• Similar to the read() function, we will need to open, write, and close the file
with open (FILENAME, "w", newline="") as file:
writer
csv.writer (file)
writer.writerows (sales)
Step 9
• Test the application thoroughly to ensure there are no syntax or logical errors.
• Add a line of pseudo-code for EACH line of source code you created.
• Save the file.](https://content.bartleby.com/qna-images/question/3c348cba-a63b-4ada-8013-6981e5c617ad/da04fe01-84ee-4faf-ba45-02fe9a620e34/86u1kl_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- write in c++Write a program that would allow the user to interact with a part of the IMDB movie database. Each movie has a unique ID, name, release date, and user rating. You're given a file containing this information (see movies.txt in "Additional files" section). The first 4 rows of this file correspond to the first movie, then after an empty line 4 rows contain information about the second movie and so forth. Format of these fields: ID is an integer Name is a string that can contain spaces Release date is a string in yyyy/mm/dd format Rating is a fractional number The number of movies is not provided and does not need to be computed. But the file can't contain more than 100 movies. Then, it should offer the user a menu with the following options: Display movies sorted by id Display movies sorted by release date, then rating Lookup a release date given a name Lookup a movie by id Quit the Program The program should perform the selected operation and then re-display the menu. For…arrow_forwardUsing JAVA OOP, needs to work on the eclipse IDE The international Olympics Committee has asked you to write a program to process their data and determine the medal winners for the pairs figure skating. You will be given a file named Pairs.txt.This file contains the data for each pair of skaters. The data consists of each skater’s name, their country and the score from each of eight judges on the technical aspects and on the performance aspects. A typical record in the file would be as follows: SmithJonesAustralia5.0 4.9 5.1 5.2 5.0 5.1 5.2 4.84.3 4.7 4.8 4.9 4.6 4.8 4.9 4.5 LennonMurrayEngland2.1 2.2 2.3 2.4 2.5 2.6 2.7 2.83.1 3.2 3.3 3.4 3.5 3.6 3.7 3.8GustoPetitotItalia4.1 4.2 4.3 4.4 4.5 4.6 4.7 4.85.1 5.2 5.3 5.4 5.5 5.6 5.7 5.8LahaiePetitFrance1.1 1.2 1.3 1.4 1.5 1.6 1.7 1.85.1 5.2 5.3 5.4 5.5 5.6 5.7 5.8BilodeauBernardCanada2.1 2.2 2.3 2.4 2.5 2.6 2.7 2.84.1 4.2 4.3 4.8 4.9 4.6 4.0 4.5LahorePedroMexico3.2 3.1 3.8 3.9 3.0 3.6 3.9 3.35.9 5.8 5.8 5.8 5.9 5.6 5.0…arrow_forwardPlease help with my C++Specifications • For the view and delete commands, display an error message if the user enters an invalid contact number. • Define a structure to store the data for each contact. • When you start the program, it should read the contacts from the tab-delimited text file and store them in a vector of contact objects. •When reading data from the text file, you can read all text up to the next tab by adding a tab character ('\t') as the third argument of the getline() function. •When you add or delete a contact, the change should be saved to the text file immediately. That way, no changes are lost, even if the program crashes laterarrow_forward
- I need this done in C++ please! This program implements the top-level menu option 'R' of the Airport Application and constitutes Part 2 of the Airport Application Project requirement. When the user selects 'R' in the airport application, she will be taken to the "page" that is displayed by this program. This program is meant to replace the flight status report program (Programming Assignment 3) that did not use arrays to store the flight status information. It also reads the information from the file token wise.arrow_forwardI am looking to write two python programs for golf data. The Westminster Amateur Golf Club has a tournament every weekend. The club president has askedyou to design and write two programs. The first program will read each player's name and golf score as keyboard input, and then save(append) these records in a file named golf.dat (Each record will have a field for the player'sname and a field for the player's score, separated by commas). The second program will read the records from the golf.dat file and displays them. n addition, italso displays the name of the player with the best (lowest) score. The output should look like this: Program 1Enter a player's name: Larry FineEnter the player's score: 20Do you want to enter another record?Enter y for yes or anything else for no: y Enter a player's name: Moe HowardEnter the player's score: 21Do you want to enter another record?Enter y for yes or anything else for no: y Enter a player's name: Curly HowardEnter the player's score: 22Do you…arrow_forwardcreateDatabaseOfProfiles(String filename) This method creates and populates the database array with the profiles from the input file (profile.txt) filename parameter. Each profile includes a persons' name and two DNA sequences. 1. Reads the number of profiles from the input file AND create the database array to hold that number profiles. 2. Reads the profiles from the input file. 3. For each person in the file 1. creates a Profile object with the information from file (see input file format below). 2. insert the newly created profile into the next position in the database array (instance variable).arrow_forward
- Filename: runlength_decoder.py Starter code for data: hw4data.py Download hw4data.py Download hw4data.pyYou must provide a function named decode() that takes a list of RLE-encoded values as its single parameter, and returns a list containing the decoded values with the “runs” expanded. If we were to use Python annotations, the function signature would look similar to this:def decode(data : list) -> list: Run-length encoding (RLE) is a simple, “lossless” compression scheme in which “runs” of data. The same value in consecutive data elements are stored as a single occurrence of the data value, and a count of occurrences for the run. For example, using Python lists, the initial list: [‘P’,‘P’,‘P’,‘P’,‘P’,‘Y’,‘Y’,‘Y’,‘P’,‘G’,‘G’] would be encoded as: ['P', 5, 'Y', 3, ‘P’, 1, ‘G’, 2] So, instead of using 11 “units” of space (if we consider the space for a character/int 1 unit), we only use 8 “units”. In this small example, we don’t achieve much of a savings (and indeed the…arrow_forwardWrite a program in C++ that creates a file. Write this data into that file column wise as a header. Then keep adding data in colum wise format. The program should check if there is data in the file and then write the data in the next row. First add: TIME DAY MONTH YEAR Then check the file and add: 01:25 07 JUNE 2008 Then check again the file and add this in next row: 14:00 30 MARCH 2019arrow_forwardplease help fix the following Scenario: Your team is working with data where dates are caputred as a string, "9/15/2019". You are tasked to create a python function named 'mdy_date' that will accept a string input, convert it to a python datetime object, and return the datetime object. Create the function in the cell below.arrow_forward
- Write a code in c++ to open a file called romania.txt for appending, only for a relevant code. You may assign any file objectarrow_forwardYou have more flexibility to implement your own function names and logic in these programs. The data files you need for this assignment can obtained from:Right-click and "Save Link As..." HUGO_genes.txt (Links to an external site.) chr21_genes.txt (Links to an external site.) Create an output directory inside your assignment4 directory called "OUTPUT" for result files, so that they will not mix with your programs. Output from your programs will be here! Your program must implement command line options for the infiles it must open, but for testing purposes it should run by default, so if no command line option is passed at the command line, the program will still run. This will help in the grading of your program. Create a Python Module called called my_io.py - You can use the same code from assignment 3's solution. Put this my_io.py Module in a subdirectory named assignment4 inside your assignment4 top-level directory (see the tree below, and see Lecture 7 on how to implement a…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
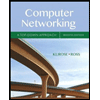
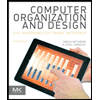
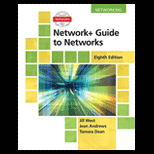
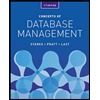
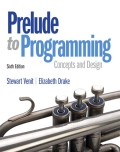
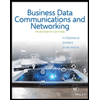