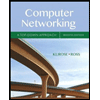
Help debugging code written in python to get the output from the attached image,
def main():
# set the initial month and declaration
month = 1
old_adults = 0
old_babies = 0
adults = 1 # initial adult pair
babies = 0 # initial babies pair
total = 0 # storing the total number of rabbit pairs
max = 500 # maximum number of rabbit pairs that can be accomodated
# output file
file = open("rabbit_sim.txt",'w')
# header of output file
file.write("# Table of rabbit pairs\n")
file.write("Month, Adults, Babies, Total\n")
# calculate the total number of rabbit pairs for the month
total = adults+babies
# loop that continues till total rabbit pairs < max
while total < max:
# output the counts to file
file.write(str(month)+", "+str(adults)+", "+str(babies)+", "+str(total)+"\n")
month += 1 # increment the month
# set the old_adults and old_babies to current adults and babies
old_adults = adults
old_babies = babies
# calculate the adults and babies for next month
babies = adults
adults = old_adults + old_babies
total = adults+babies # calculate the total number of rabbit pairs for the month
# output the counts when the total rabbit pairs > 500
file.write(str(month)+", "+str(adults)+", "+str(babies)+", "+str(total)+"\n")
# output the month number when it runs out of cages
file.write("# Cages will run out in month "+str(month))
# close the file
file.close()
if __name__ == '__main__':
main() #excucte main function
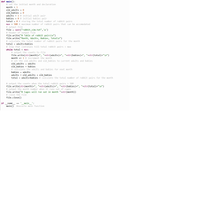
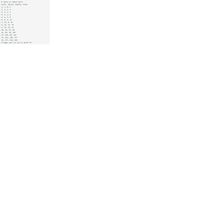

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- Python: Data = [ { "creatt": "2022-02-28T13:05:24" }, { "creat": "2022-02-21T23:49:33" }, { "creat": "2022-02-21T11:08:23" }, { "creat": "2022-02-19T10:06:46", "xxx": [ "23", "1", "9" ] }, { "creat": "2022-01-25T13:12:49", "xxx": [ "2" ] } ] How to count "xxx": ["23", "1", "9"] and "xxx": ["2"], then the total should be 4. I would like to return the 4. my desired code: { "total": 4 }arrow_forwardComputer Science JAVA #7 - program that reads the file named randomPeople.txt sort all the names alphabetically by last name write all the unique names to a file named namesList.txt , there should be no repeatsarrow_forwardWrite a code segment that searches a Grid object for a negative integer. The loop should terminate at the first instance of a negative integer in the grid, and the variables row and column should be set to the position of that integer. Otherwise, the variables row and column should equal the number of rows and columns in the grid. *Pythonarrow_forward
- Question 2. package sortsearchassigncodeex1; import java.util.Scanner; import java.io.*; // // public class Sortsearchassigncodeex1 { // public static void fillArray(Scanner inputFile, int[] arrIn){ int indx = 0; //Complete code { arrIn[indx] = inputFile.nextInt(); indx++; } }arrow_forwardIn Java please,arrow_forward1) What is one main disadvantage of an ArrayList? 2) Write a Java statement to create an ArrayList called list to hold 25 integers. 3) What is the type of the ArrayList defined in question number 2? 4) Write a for loop to initialize the objects in the ArrayList created in question number 2 above to -1.arrow_forward
- Non dynamic.arrays are allocated at runtime where dynamic arrays are allocated at compile time. True False Question 4 There are other ways to end a loop other than just the loop condition evaluating to false. True Falsearrow_forwardin java #6 - program that reads the name data from the files named firstNames.txt and lastNames.txtand produces a list of 1000 random names randomPeople.txtone complete name (firstname lastname) per linearrow_forwardEnhanced selection sort algorithm SelectionSortDemo.java package chapter7; /** This program demonstrates the selectionSort method in the ArrayTools class. */ public class SelectionSortDemo { public static void main(String[] arg) { int[] values = {5, 7, 2, 8, 9, 1}; // Display the unsorted array. System.out.println("The unsorted values are:"); for (int i = 0; i < values.length; i++) System.out.print(values[i] + " "); System.out.println(); // Sort the array. selectionSort(values); // Display the sorted array. System.out.println("The sorted values are:"); for (int i = 0; i < values.length; i++) System.out.print(values[i] + " "); System.out.println(); } /** The selectionSort method performs a selection sort on an int array. The array is sorted in ascending order. @param array The array to sort. */ public static void selectionSort(int[] array) { int startScan, index, minIndex, minValue; for (startScan = 0; startScan < (array.length-1); startScan++) { minIndex = startScan; minValue…arrow_forward
- Computer Science Part C: Interactive Driver Program Write an interactive driver program that creates a Course object (you can decide the name and roster/waitlist sizes). Then, use a loop to interactively allow the user to add students, drop students, or view the course. Display the result (success/failure) of each add/drop.arrow_forwarddef ppv(tp, fp): # TODO 1 return def TEST_ppv(): ppv_score = ppv(tp=100, fp=3) todo_check([ (np.isclose(ppv_score,0.9708, rtol=.01),"ppv_score is incorrect") ]) TEST_ppv() garbage_collect(['TEST_ppv'])arrow_forwardBAGEL FILES import javax.swing.JFrame; public class Bagel{//-----------------------------------------------------------------// Creates and displays the controls for a bagel shop.//-----------------------------------------------------------------public static void main (String[] args){JFrame frame = new JFrame ("Bagel Shop");frame.setDefaultCloseOperation (JFrame.EXIT_ON_CLOSE); frame.getContentPane().add(new BagelControls()); frame.pack();frame.setVisible(true);}} import java.awt.*;import java.awt.event.*; import javax.swing.*; public class BagelControls extends JPanel{private JComboBox bagelCombo;private JButton calcButton;private JLabel cost;private double bagelCost;public BagelControls(){String[] types = {"Make A Selection...", "Plain","Asiago Cheese", "Cranberry"};bagelCombo = new JComboBox(types);calcButton = new JButton("Calc");cost = new JLabel("Cost = " + bagelCost);setPreferredSize (new Dimension (400,…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
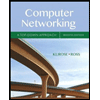
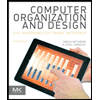
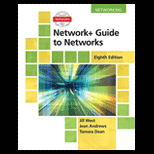
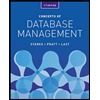
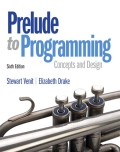
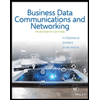