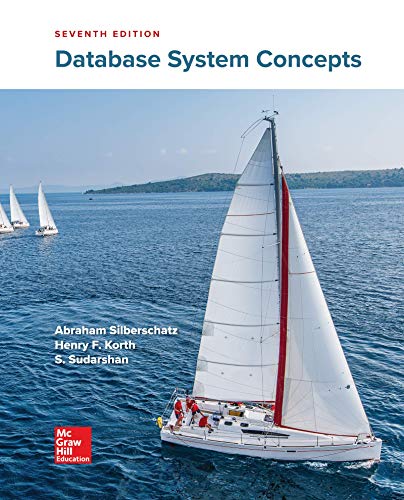
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Here is the iterative implementation of binary search:
For each call to binary_search below, indicate how many times the code inside the while loop will execute.
animals = ["aardvark", "cat", "dog", "elephant", "panda"]1. binary_search("elephant", animals)
2. binary_search("dog", animals)
3. binary_search("anteater", animals)
![9.
def binary_search(item, lst):
10
11
Function
binary_search
--
12
Perform a binary search for a given item. Return True when a
matching item is found. Return False if no match is found.
13
14
Parameters:
15
item
the item to search for.
--
16
lst
the list to search in.
--
17
18
left_index
= 0
right_index
found = False
19
len (lst) - 1
%3D
20
21
right_index and not found:
(left_index + right_index) // 2
22
while left_index <=
23
middle
if lst[middle]
found = True
24
item:
25
26
elif item < lst[middle]:
27
# Search left
28
right_index
middle - 1
29
else:
30
# Search right
31
left_index
= middle + 1
32
return found](https://content.bartleby.com/qna-images/question/39628135-d8f9-472b-a48d-a4792a06b79c/db082a22-3f17-4413-a9c9-c1a3aa6edbb1/nrv306_thumbnail.png)
Transcribed Image Text:9.
def binary_search(item, lst):
10
11
Function
binary_search
--
12
Perform a binary search for a given item. Return True when a
matching item is found. Return False if no match is found.
13
14
Parameters:
15
item
the item to search for.
--
16
lst
the list to search in.
--
17
18
left_index
= 0
right_index
found = False
19
len (lst) - 1
%3D
20
21
right_index and not found:
(left_index + right_index) // 2
22
while left_index <=
23
middle
if lst[middle]
found = True
24
item:
25
26
elif item < lst[middle]:
27
# Search left
28
right_index
middle - 1
29
else:
30
# Search right
31
left_index
= middle + 1
32
return found
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- + enuity.com/Player/ emester A 4 5 Mark this and return M BO DELL A user is going to process an unspecified quantity of numbers. The desired result is to have the numbers ordered from smallest to largest. Which statement is true? Select three options. You could use a loop to add the numbers to a list, and then use an insertion sort to order the list. You could use a loop to add the numbers to a dictionary, and then use a built-in sort method to sort the dictionary. You could use a loop to ask the user for the numbers, adding them to a list. Then use the built-in sort method to order the list. You could use a loop to add numbers to a list. Then use a binary search to sort the list. You could use a spreadsheet to enter the numbers, and then use the spreadsheet sort method from the menu to sort the numbers. Save and Exit Next < English Sign out Kinley TIME REMAINING 57:01 Submit D Mar 27 12:0arrow_forwardModify the quick sort implementation in the textbook to sort the array using pivot as the median of the first, last, and middle elements of the array. Add the modified quick sort implementation to the arrayListType class provided (arrayListType.h). Ask the user to enter a list of positive integers ending with -999, sort the integers, and display the pivots for each iteration and the sorted array. Main Function #include <iostream>#include "arrayListType.h"using namespace std; int main(){ arrayListType<int> list;int num;cout << "Line 8: Enter numbers ending with -999" << endl;cin >> num; while (num != -999){list.insert(num);cin >> num;} cout << "Line 15: The list before sorting:" << endl;list.print();cout << endl; list.selectionSort();cout << "Line 19: The list after sorting:" << endl;list.print();cout << endl; return 0;} Header File (arrayList.h) Including images #include <iostream>#include <cassert>…arrow_forward1. The codewords will be scrambled words. You will read the words used for the codes from a file and store it into an array. There are 60 words in the file that range in size from 3 characters to 7 characters. The file is called wordlist.txt and can be found attached to the assignment in IvyLearn.2. Start with input for an integer seed for the random number generator. There is no need to put a prompt before the cin operation.3. Ask the Player if they are ready to play and only proceed if they type a Y or y. If the user types an N or n then the program should finish. a. INPUT VALIDATION: Make sure the user has typed either y, Y, n, or N4. Create a variable to keep track of the number of guesses the user has made.5. Use the random number generator to pick a word from the list of words.6. Once you have chosen a word as the codeword you will need to scramble the letters to make it into a code. You should use the random number generator to help you mix up the letters.7. Display to the user…arrow_forward
- Q1. The last post didnt include the items in the database. Need a different function for the python program display_ticket below: def display_tickets():t = Texttable()tickets=cur.execute('''SELECT * FROM tickets''')for i in tickets:t.add_rows([['tid', 'actual_speed','posted_speed','age','violator_sex'],list(i)])print()print(t.draw())print() Q2. Also need a different function for the python program add_ticket below: def add_tickets():actual_speed=int(input("enter actual speed:"))posted_speed=int(input("enter posted speed:"))age=int(input("enter age:"))sex=input("Male or Female:")cur.execute('''INSERT INTO tickets(actual_speed,posted_speed,age,violator_sex) VALUES(?,?,?,?)''',(actual_speed,posted_speed,age,sex))con.commit() If you'd like to see what thousands of traffic tickets look like, check out this link: https://www.twincities.com/2017/08/11/we-analyzed-224915-minnesota-speeding-tickets-see-what-we-learned/arrow_forwardHomework 7: Merging two sorted arrays Due date: Saturday March 18. Objectives: Learn to implement algorithms that work with multiple arrays. Understand the Merging algorithm. IMPORTANT. Carefully study Lecture 1 from Week 8 on D2L before you start on this. Sometimes we need to combine the values in two sorted sequences to produce a larger sorted sequence. This process is called Merging. As an example, if the first sequence (S1)contains the numbers 2 3 4 4 5 9 and the second sequence (S2) contains the numbers 1 4 5 8 9 11 12 13 17 the output sequence will contain 1 2 3 4 4 4 5 5 8 9 9 11 12 13 17 Each sequence can be stored in an array. The strategy is as follows: Start by comparing the first items in the two input arrays. Write the smaller number to the output array, and move to the the next item in that array. This is repeated until we reach the end of any one of the input arrays; thereafter, we simply copy all the numbers from the other input array to the output array. (In the…arrow_forwardIn python make a function with the parameter of a dict[str, str] the function will simply invert the dictionary do not use .get, .item, .values, or import builtins If you encounter more than one of the same key when trying to invert the key and value, raise a keyerror for example ['john': 'white', 'garcia': 'white'], should trigger the raise KeyError callarrow_forward
- def makeRandomList(size): lyst = [] for count in range(size): while True: number = random.randint(1, size) if not number in lyst: lyst.append(number) break return lyst give me proper analysis of this code and Big Oarrow_forwardCode in R Write a while loop what prints all numbers up to 20, but it skips a collection of numbers: 3,9,13,19. A next statement is useful when we want to skip the current iteration of a loop without terminatingit. On encountering next, the R parser skips further evaluation and starts next iteration of the loop. x <- 1:5for (val in x) {if (val == 3){next}print(val)}arrow_forwardIn Python IDLE: How would I write a function for the problem in the attached image?arrow_forward
- import numpy as np%matplotlib inlinefrom matplotlib import pyplot as pltimport mathfrom math import exp np.random.seed(9999)def is_good_peak(mu, min_dist=0.8): if mu is None: return False smu = np.sort(mu) if smu[0] < 0.5: return False if smu[-1] > 2.5: return False for p, n in zip(smu, smu[1:]): #print(abs(p-n)) if abs(p-n) < min_dist: return False return True maxx = 3ndata = 500nset = 10l = []answers = []for iset in range(1, nset): npeak = np.random.randint(2,4) xs = np.linspace(0,maxx,ndata) ys = np.zeros(ndata) mu = None while not is_good_peak(mu): mu = np.random.random(npeak)*maxx for ipeak in range(npeak): m = mu[ipeak] sigma = np.random.random()*0.3 + 0.2 height = np.random.random()*0.5 + 1 ys += height*np.exp(-(xs-m)**2/sigma**2) ys += np.random.randn(ndata)*0.07 l.append(ys) answers.append(mu) p6_ys = lp6_xs =…arrow_forwardWrite a version of the binary search algorithm that can be used to search a list of strings. (Use the selection sort that you designed to sort the list.)arrow_forwardIn Java, Apply replacement selection sort to the following list assuming array size M = 3; 27 47 35 7 67 21 32 18 24 20 12 8 Show the content of each sorted file.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
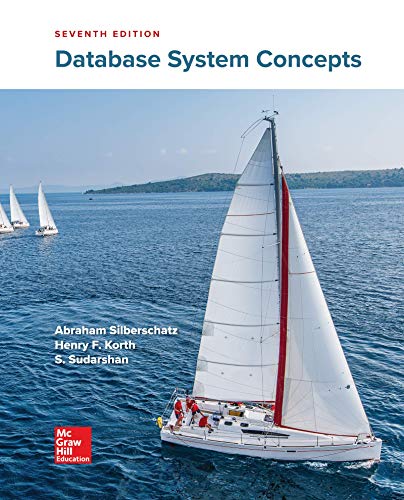
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
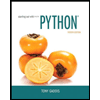
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
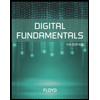
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
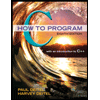
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
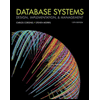
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
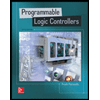
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education