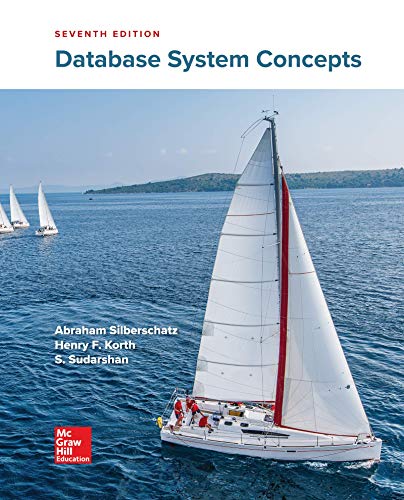
Concept explainers
How can add interface java file to these three java files that runs together?
Java file 1:
package property2;
import java.util.Scanner;
public class Property2 {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
// Read property name and rent as input values from the keyboard
System.out.println("Enter property name: ");
String propertyName=in.nextLine();
System.out.println("Enter property rent amount: ");
int rent=Integer.parseInt(in.nextLine());
Property property = new Apartment(propertyName, rent);
System.out.println("Property Name: "+property.getPropertyName());
System.out.println("Property Rent$: "+property.getRentAmount());
}
}
Java file 2:
package property2;
import javax.lang.model.SourceVersion;
abstract class Property
{
protected String propertyName;
protected int rentAmount;
public Property(String propertyName, int rentAmount)
{
this.propertyName = propertyName;
this.rentAmount = rentAmount;
}
public abstract String getPropertyName();
public abstract int getRentAmount();
}
Java file 3:
package property2;
class Apartment extends Property
{
/*Apartment class constructor that takes two input
* arguments and calls the constructor of the abstract class*/
public Apartment(String PropertyName, int RentAmount)
{
super(PropertyName, RentAmount);
}
@Override
/*
* Return propertyName value
* */
public String getPropertyName() {
return propertyName;
}
@Override
/*
* Return rentAmount value
* */
public int getRentAmount() {
return rentAmount;
}
}

Step by stepSolved in 6 steps with 3 images

- program that takes a first name as the input, and outputs a welcome message to that name. Ex: If the input is: Chrystal the output is: Hey Chrystal! Welcome to zyBooks!arrow_forwardusing System; class Program { publicstaticvoid Main(string[] args) { int number,max=-9999999; while (true) { Console.WriteLine("Enter a number: "); number=Convert.ToInt32(Console.ReadLine()); if(number==0) { break; } else { if(number>max) { max=number; } } } Console.WriteLine("Largest number is "+max); } } Hello! Hope you are well! This C# program gets numbers from the user and prints the largest one. How can it be fixed to only ask for 6 numbers from the user and then choose the largest one? Thank you!arrow_forwardjava programming I have a Java program with a restaurant/store menu. Can you please edit my program when displaying the physical receipt? I would like the physical receipt to export a PDF file, not printing the order receipt in the console. import java.util.*; public class Restaurant2 { publicstaticvoidmain(String[] args) { // Define menu items and pricesString[] menuItems= {"Apple", "Orange", "Pear", "Banana", "Kiwi", "Strawberry", "Grape", "Watermelon", "Cantaloupe", "Mango"};double[] menuPrices= {1.99, 2.99, 3.99, 4.99, 5.99, 6.99, 7.99, 8.99, 9.99, 10.99};StringusersName; // The user's name, as entered by the user.ArrayList<String> arr = new ArrayList<>(); // Define scanner objectScanner input=new Scanner(System.in); // Welcome messageSystem.out.println("Welcome to AppleStoreRecreation, what is your name:");usersName = input.nextLine(); System.out.println("Hello, "+ usersName +", my name is Patrick nice to meet you!, May I Take Your Order?"); // Display menu items…arrow_forward
- The program introduces goto and Label concepts. Add your own label B_label, add goto in any place of the program to jump to it (to your label) using System; using static System.Console; namespace HandlingExceptions { class Program { static void Main(string[] args) { WriteLine("Before parsing"); Write("What is your age? "); string input = ReadLine(); try { int age = int.Parse(input); WriteLine($"You are {age} years old."); } catch (OverflowException) { WriteLine("Your age is a valid number format but it is either too big or small."); } catch (FormatException) { WriteLine("The age you entered is not a valid number format."); } catch (Exception ex) { WriteLine($"{ex.GetType()} says {ex.Message}"); } WriteLine("After parsing"); } } }arrow_forwardimport java.util.Scanner;/** This program computes the time required to double an investment with an annual contribution.*/public class DoubleInvestment{ public static void main(String[] args) { final double RATE = 5; final double INITIAL_BALANCE = 10000; final double TARGET = 2 * INITIAL_BALANCE; Scanner in = new Scanner(System.in); System.out.print("Annual contribution: "); double contribution = in.nextDouble(); double balance = INITIAL_BALANCE; int year = 0; // TODO: Add annual contribution, but not in year 0 do { year++; double interest = balance*(RATE/100); balance = (balance+contribution+interest); } while(balance< TARGET); System.out.println("Year: " + year); System.out.printf("Balance: %.2f%n", balance); }}arrow_forwardpublic static void printIt(int value) { if(value 0) { } System.out.println("Play a paladin"); System.out.println("Play a white mage"); }else { } }else if (value < 15) { System.out.println("Play a black mage"); System.out.println("Play a monk"); } else { public static void main(String[] args) { printit (5); printit (10); printit (15); Play a black mage Play a white mage (nothing/they are all printed) Play a paladin Play a monkarrow_forward
- Complete the isExact Reverse() method in Reverse.java as follows: The method takes two Strings x and y as parameters and returns a boolean. The method determines if the String x is the exact reverse of the String y. If x is the exact reverse of y, the method returns true, otherwise, the method returns false. Other than uncommenting the code, do not modify the main method in Reverse.java. Sample runs provided below. Argument String x "ba" "desserts" "apple" "regal" "war" "pal" Argument String y "stressed" "apple" "lager" "raw" "slap" Return Value false true false true true falsearrow_forwardWrite a statement that increases numPeople by 5. Ex: If numPeople is initially 10, the output is: There are 15 people. 367012.2549490.qx3zqy7 1 import java.util.Scanner; 2 3 public class AssigningNumberToVariable { public static void main(String[] args) { new Scanner(System.in); 4 5 Scanner scnr int numPeople; 7 8 numPeople scnr.nextInt(); 9 10 /* Your solution goes here * 11 System.out.print("There are "); System.out.print(numPeople); System.out.println(" people."); } 12 13 14 15 16 }arrow_forwardCorrect Code for Java: Enter an integer >> 11 Enter another integer >> 23 The sum is 34 The difference is -12 The product is 253 import java.util.Scanner; public class DebugTwo2 { public static void main(String args[]) { int a, b; Scanner input = new Scanner(System.in); System.out.print("Enter an integer >> "); a = nextInt (); System.out.print("Enter b = nextInt (); System.out.println("The System.out.println("The System.out.println("The } } another integer >> "); sum is " + (a + b)); difference is " + (a - b)); product is + (a*b));arrow_forward
- pRogramming Java Matcherarrow_forwardEdit the code below to print the volume of the roomarrow_forwardSimple try-catch Program This lab is a simple program that demonstrates how try-catch works. You will notice the output when you enter incorrect input (for example, enter a string or double instead of an integer). Type up the code, execute and submit the results ONLY. Do at least 2 valid inputs and 1 invalid. NOTE: The program stops executing after it encounters an error! CODE: import java.util.Scanner; public class TryCatchExampleSimple { public static void main(String[] args) { Scanner input = new Scanner(System.in); int num = 0; System.out.println("Even number tester.\n"); System.out.println("Enter your name: "); String name input.nextLine(); } } while (true) { try { System.out.println("Enter an integer : "); num= input.nextInt (); } int mod = num%2; if (mod == 0) " System.out.println(name + else " System.out.println(name + num = 0; } catch (Exception e) { break; > System.out.println("ERROR: The number you entered is illegal!"); System.out.println("Exception error: "+e.toString());…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
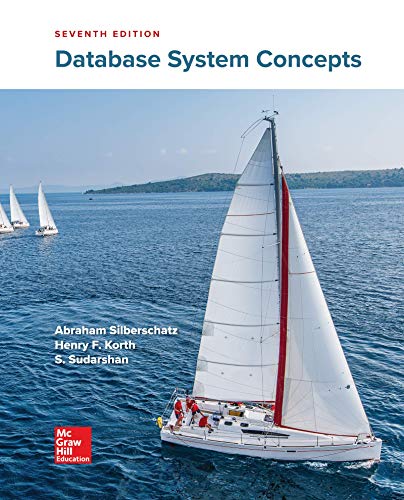
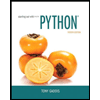
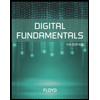
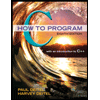
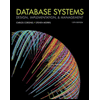
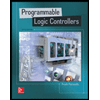