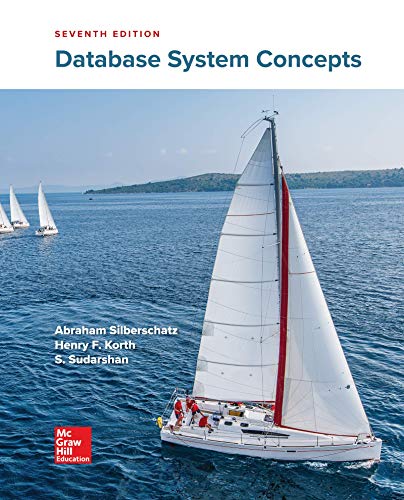
Concept explainers
I am trying to program a hangman game in C++ using Dev-C++, complete with an ASCII-based main menu. This is the code that I have so far:
//What is this code and what is it doing?
#include <cstdlib> // What do each of these do?
#include <ctime>
#include <iostream>
using namespace std;
//What is this code doing?
int NUM_TRY = 7;
int checkGuess (char, string, string);
void menu();
string message = "Play!";
//What is this code doing?
int main(int argc, char *argv[])
{
string name;
char letter;
string animal;
string animals[] =
{
"owl",
"bear",
"ocelot",
"whale",
"zebra",
"armadillo",
"chimpanzee",
"alligator",
"seahorse",
"feline",
"bovine",
"horse",
"canine"
};
//What is this code doing?
srand(time(NULL));
int n=rand()% 12;
animal=animals[n];
string hide_m(animal.length(),'X');
//What is this code and what is it doing?
while (NUM_TRY!=0)
{
menu();
cout << "\n\n\t\t\t\t" << hide_m;
cout << "\n\n\t\t\t\tGuess a letter: ";
cin >> letter;
if (checkGuess(letter, animal, hide_m)==0)
{
message = "Incorrect letter.";
NUM_TRY = NUM_TRY - 1;
}
else
{
message = "NICE! You guessed a letter";
}
if (animal==hide_m)
{
message = "Congratulations! You got it!";
menu();
cout << "\n\t\t\t\tThe animal is : " << animal << endl;
break;
}
}
if(NUM_TRY == 0)
{
message = "No!! You've been hanged.";
menu();
cout << "\n\t\t\t\tThe animal was : " << animal << endl;
}
cin.ignore();
cin.get();
return 0;
}
//What is this code and what is it doing?
int checkGuess (char guess, string secretanimal, string &guessanimal)
{
int i;
int matches=0;
int len=secretanimal.length();
for (i = 0; i < len; i++)
{
if (guess == guessanimal[i])
return 0;
if (guess == secretanimal[i])
{
guessanimal[i] = guess;
matches++;
}
}
return matches;
}
menu{(
//What is this code and what is it doing?
system("color 05"); //change the color!
system("cls");
cout << "\t\t\t\t*\t*";
cout<<"\t\t\t\t**\t**";
cout<<"\t\t\t\t***\t***";
cout<<"\t\t\t\t****\t****";
cout<<"\t\t\t\t*****\t*****";
cout<<"\t\t\t\t******\t******";
cout<<"\t\t\t\t*******\t*******";
cout<<"\t\t\t\t*******\t*******";
cout<<"\t\t\t\t******\t******";
cout<<"\t\t\t\t*****\t*****";
cout<<"\t\t\t\t****\t****";
cout<<"\t\t\t\t***\t***";
cout<<"\t\t\t\t**\t**";
cout<<"\t\t\t\t*\t*";
//What is this code and what is it doing?
cout<<"\t\t@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@";
cout << "\n\t\t\t\tHangman Game!"; //Feel free to change the wording
cout << "\n\t\tYou have " << NUM_TRY << " tries to try and guess the animal.";
cout << "\n\n\t\t\t\t" + message;
cout<<"\n\t\t@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@\n";
)}
I cannot get the code to compile because of ONE error. Some help would be greatly appreciated.

Step by stepSolved in 4 steps with 4 images

- I've been asking about this poker game that I have been trying to compile in c++ Main.cpp #include <iostream>#include <string>#include <fstream>#include <iomanip>#include <sstream>#include "card.h"#include "deck.h"#include "hand.h"using namespace std;int main(){string repeat = "Y";Deck myDeck;Hand myHand;string exchangeCards;while (repeat == "Y" || repeat == "y"){cout << endl;myHand.newHand(myDeck);myHand.print();cout << endl;cout << "Would you like to exchange any cards? [Y / N]: ";getline(cin, exchangeCards);while (exchangeCards != "Y" && exchangeCards != "y" && exchangeCards != "X" && exchangeCards != "n"){cout << "Please enter Y or N only: ";getline(cin, exchangeCards);}if(exchangeCards == "Y" || exchangeCards == "y"){myHand.exchangeCards(myDeck);}cout << endl;myHand.print();cout << endl;myDeck.reset(); // Resets the deck for a new gamecout << "Play again? [Y / N]: ";getline(cin,…arrow_forward// // main.c // Assignment1 // // Created by Hassan omer on 15/10/21. // #include <stdio.h> # include <stdlib.h> int input(); int multiples(); int cions(); void display_change(); int main() { int num; num = input(); multiples(num); cions(); display_change(); return 0; } int input(int num) { printf("enter 5-95 number\n"); scanf("%d",&num); return num; } int multiples(int num) { int sum5 =0; if (sum5 %5 != 0 ||sum5<5|| sum5 >95) { printf("invaild input %d",sum5); } cions(sum5); return sum5; } int cions(int sum05){ int cent50 = 0; int cent20 = 0; int cent10 = 0; int cent05 = 0; if (sum05 > 0) { if (sum05 >= 50){ sum05 -= 50; cent50++; } else if (sum05 >=20){ sum05 -= 20; cent20++; } else if (sum05 >= 10){ sum05 -=10;…arrow_forward>> IN C PROGRAMMING LANGUAGE ONLY << COPY OF DEFAULT CODE, ADD SOLUTION INTO CODE IN C #include <stdio.h>#include <stdlib.h>#include <string.h> #include "GVDie.h" int RollSpecificNumber(GVDie die, int num, int goal) {/* Type your code here. */} int main() {GVDie die = InitGVDie(); // Create a GVDie variabledie = SetSeed(15, die); // Set the GVDie variable with seed value 15int num;int goal;int rolls; scanf("%d", &num);scanf("%d", &goal);rolls = RollSpecificNumber(die, num, goal); // Should return the number of rolls to reach total.printf("It took %d rolls to get a \"%d\" %d times.\n", rolls, num, goal); return 0;}arrow_forward
- in c++ In the preceding exercises, you saw how one can use stepwise refinement to solve the problem of translating Wiki markup to HTML. Now turn the pseudocode into code. Complete the convert_to_HTML and next_symbol functions.............. #include <iostream>#include <string>using namespace std; // Functions defined belowstring convert_to_HTML(string message);int next_symbol(string message, string symbols, int start);string replace_at(string str, int position, string replacement); string tag_for_symbol(string symbol);string replace_escapes(string str); /**Converts a message with Wiki markup to HTML.@param message the message with markup@return the message with Wiki notation converted to HTML*/string convert_to_HTML(string message){ string result = message;/* Your code goes here */return replace_escapes(result);} /**Finds the next unescaped symbol.@param message a message with Wiki markup@param symbols the symbols to search for@param start the starting position for the…arrow_forwardin C++ , change the code to do the same purpose and same output : Source Code: #include<iostream>#include<fstream>#include<string> using namespace std; void displayPlain();void displayHex(); void executeCommand(int choice){ switch (choice) { case 1: displayPlain(); break; case 2: displayHex(); break; case 9: exit(0); default: cout<<"Invalid Choice Entered!!\n"; }} void displayMenu(){ cout<<"\n===============Menu==========================\n\n"; cout<<"1. Select 1 to see file data in plain text\n"; cout<<"2. Select 2 to see file data in hexadecimal\n"; cout<<"3. Select 9 to quit\n\n";} int main(){ int choice; do{ displayMenu(); cout<<"choice: "; cin>>choice; executeCommand(choice); }while(choice != 9); return 0;} void displayPlain(){ fstream file; file.open("q4File.txt", ios::in); string line;…arrow_forwardQuestion: In C#, how can I recreate this into a Windows form Application instead of a console application? Please show a photo of the display and show the code that you used, thank you! Problem: Write a function Seperate( number ) that separates an integer number (ranging from 0 to 99999) into its digits. For example, if the number is 42329, the function finds 4, 2, 3, 2, 9. If the number is 323, the function finds 0, 0, 3, 2, 3. (Hint: use modulus and integer division operations.) Code: using System;public class RecExercise4{static void Main(){Console.Write("Input any number (ranging from 0 to 99999) : ");int num = Convert.ToInt32(Console.ReadLine());Console.Write(" The digits in the number are : ");separateDigits(num);} static void separateDigits(int n){int i = 4;int[] arr;arr = new int[5];while (i>-1){arr[i] = n%10;n = n/10;i--;}foreach(int j in arr)Console.Write(" " + j);}}arrow_forward
- I've been asking about this poker game that I have been trying to compile in c++. Main.cpp #include <iostream>#include <string>#include <fstream>#include <iomanip>#include <sstream>#include "card.h"#include "deck.h"#include "hand.h"using namespace std;int main(){string repeat = "Y";Deck myDeck;Hand myHand;string exchangeCards;while (repeat == "Y" || repeat == "y"){cout << endl;myHand.newHand(myDeck);myHand.print();cout << endl;cout << "Would you like to exchange any cards? [Y / N]: ";getline(cin, exchangeCards);while (exchangeCards != "Y" && exchangeCards != "y" && exchangeCards != "X" && exchangeCards != "n"){cout << "Please enter Y or N only: ";getline(cin, exchangeCards);}if(exchangeCards == "Y" || exchangeCards == "y"){myHand.exchangeCards(myDeck);}cout << endl;myHand.print();cout << endl;myDeck.reset(); // Resets the deck for a new gamecout << "Play again? [Y / N]: ";getline(cin,…arrow_forwardCreate a flowchart for this program in c++, #include <iostream>#include <vector> // for vectors#include <algorithm>#include <cmath> // math for function like pow ,sin, log#include <numeric>using std::vector;using namespace std;int main(){ vector <float> x, y;//vector x for x and y for y float x_tmp = -2.5; // initial value of x float my_function(float x); while (x_tmp <= 2.5) // the last value of x { x.push_back(x_tmp); y.push_back(my_function(x_tmp)); // calculate function's value for given x x_tmp += 1;// add step } cout << "my name's khaled , my variant is 21 ," << " my function is y = 0.05 * x^3 + 6sin(3x) + 4 " << endl; cout << "x\t"; for (auto x_tmp1 : x) cout << '\t' << x_tmp1;//printing x values with tops cout << endl; cout << "y\t"; for (auto y_tmp1 : y) cout << '\t' << y_tmp1;//printing y values with tops…arrow_forwardThis assignment will give you practice on basic C programming. You will implement a few Cprogramsarrow_forward
- This assignment will give you practice on basic C programming. You will implement a few Cprogramsarrow_forwardList two features we used from the C++ standard library, including a short explanation of why you would use the feature and which header file must be included. Example: The std::cin object is found in the iostream header file and is used to read characters from the keyboard.arrow_forwardIn C++ please follow the instructions Write two code blocks -- one code block to declare a bag and its companion type-tracking array (both using the STL vector), and another code block to create and declare a Cat object and put it into the bag. Name the arrays and variables as you wish. Use any data type tracking and any designation for cats -- your choices. Assume that struct Cat is already defined and that all required libraries are properly included -- just write the two separate code blocks, separated by one or more blank lines.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
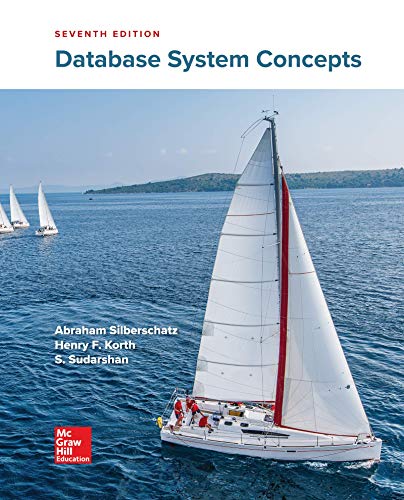
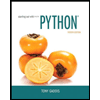
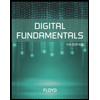
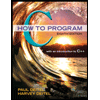
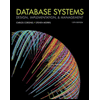
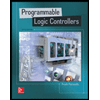