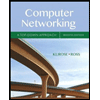
I have a quick question about an assignment with python, I am using data validation to validate that the user input does not go above a set amount, but I am not sure how to pass the variable by reference. Here is the code
# Theater Seating Lab
# Abisai Saavedra
# the main function
def main():
# constants
COST_A = 20
COST_B = 15
COST_C = 10
A = 300
B = 500
C = 200
programIntro(COST_A,COST_B,COST_C,A,B,C)
keepGoing = False
while (keepGoing == False):
totalA,totalB,totalC = get_section ()
display_output(totalA,totalB,totalC)
keepGoing = get_yes_or_no("Are you finished inputting ticket sales? Type yes or no:")
print("Thank you for using the
# brief explanation of program
def programIntro(COST_A,COST_B,COST_C,A,B,C):
print("Hello, welcome to Seatings Calculator")
print("Please follow the system prompts to place number of tickets sold per section")
print("After giving the program the proper information, you will get a total of revenue earned.")
print("----------------------------")
print("Prices:")
print(" o Section A Seatings = $", COST_A,"-" ,A, "MAX seats")
print(" o Section B Seatings = $", COST_B,"-" ,B, "MAX seats")
print(" o Section C Seatings = $", COST_C,"-" ,C, "MAX seats")
print("----------------------------")
# gathers information submitted by user
def get_section ():
soldA = get_valid_nbr("How many seats were sold in section A")
soldB = get_valid_nbr("How many seats were sold in section B")
soldC = get_valid_nbr("How many seats were sold in section C")
return soldA,soldB,soldC
def display_output(totalA,totalB,totalC):
if totalA > 0:
print("you have sold", totalA * 20, "worth of seats in section A by selling", totalA)
if totalB > 0:
print("you have sold", totalB * 15, "worth of seats in section B by selling", totalB)
if totalC > 0:
print("you have sold", totalC * 10, "worth of seats in section C by selling", totalC)
# function that validates user input
def get_yes_or_no(message):
while True:
new_value = get_string(message).lower()
if new_value == "y" or new_value == "yes":
return True
if new_value == "n" or new_value == "no":
return False
print('Error: invalid value entered')
# function that validates user int input
def get_valid_nbr(message):
new_value = get_integer(message)
while is_invalid(new_value):
new_value = get_integer("Please enter a number greater than 0")
return new_value
# function that returns True or False
def is_invalid(new_value):
if new_value > 300:
print("Theater seatings sold cannot be {0}, it must be lesser than or equal to 300".format(new_value))
return True
if new_value > 500:
print("Theater seatings sold cannot be {0}, it must be lesser than or equal to 500".format(new_value))
return True
if new_value > 200:
print("Theater seatings sold cannot be {0}, it must be lesser than or equal to 200".format(new_value))
return True
if new_value > 0:
return False
# validation for input
def get_integer(message):
while True:
try:
new_value = int(input(message))
return new_value
except ValueError:
print(' Error: non-integer value entered')
def get_string(message):
while True:
new_value = input(message)
if new_value and new_value.strip():
return new_value
else:
print(' Error: no data entered')
# calls main
if __name__ == '__main__':
main()

Step by stepSolved in 3 steps

- Could you help me create a python code for this question? Thanks.arrow_forwardI need help solving this in PYTHONarrow_forwardI can't seem to figure this out.. for python You have a business that cleans floors in commercial space and offices. You charge customers $0.45 per square yard, and some customers require multiple cleanings every month. You need two custom functions for your business. The first function takes a floor's length and width in feet and returns the area in square yards. The second void function takes the area, charge per square yard, and number of monthly cleanings as arguments and prints the cleaning cost. This latter function uses selection logic to print different output for single and multiple monthly cleanings (see Sample Outputs). Use a properly named constant for the charge per square yard, too.arrow_forward
- I need some help with project 5 in program logicarrow_forwardI am creating a c++ snakes and ladders game (no visuals). I want the game to have up to 5 or 6 players. For the snakes/ladders I am going to create a function which needs the dice roll and adds it to the score which will then decide if the player has landed on a snake or a ladder, is there any way I can get all players scores to be stored in the “score” variable in the switch statements without using multiple switch statements? So I basically want a switch statement which can accommodate all 1-6 players . E.g p1 initially at 1 rolls a 4. So their new score is 1+4=5, then the switch function checks for a ladder or snake. Then player 2 who's initially a 2 rolls a 5, so their new score is 2+5=7 then THE SAME switch function checks for a snake or ladder... And so on for all other players.arrow_forwardProblem taken from LeetCode // Problem Statement : // You are given a string. // Write a function that takes a string as input and reverse only the vowels of a string. // Example : // Sample Input - 1 : // "hello" // Sample Output - 1 : // "holle" // Sample Input - 2 : // "leetcode" // Sample Output - 2 : // "leotcede" class Solution {public: string reverseVowels(string s) { int i = 0 , j = s.size() - 1; while(i < j) { while(i < j && (s[i] != 'a' && s[i] != 'e' && s[i] != 'i' && s[i] != 'o' && s[i] != 'u' && s[i] != 'A' && s[i] != 'E' && s[i] != 'I' && s[i] != 'O' && s[i] != 'U' )) { i++;…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
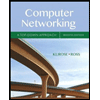
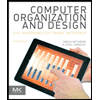
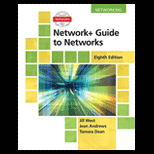
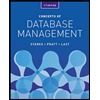
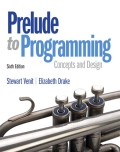
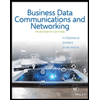