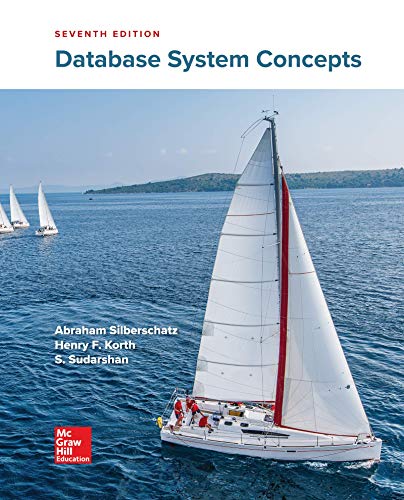
i need this practice problem in C++
part 1
28. This exercise uses a
(i) Use the size() operation in the vector class to implement the priority-queue functions size() and empty().
(ii) The push() operation inserts a new element at the back of the vector by using push_back(). The elements in the vector are not ordered in any way. Assign the boolean data member recomputeMaxIndex the value true, since the new value may be the largest in the priority queue. Part (iii) describes this variable.
pqVector
pqVector
before push(4)
3521
after push(4) 35214
(iii) The functions top() and pop() must compute the largest of the pqVector.size() el- ements. Do this computation with the private-member function findMaxIndex (). which assigns to the data member maxIndex the index of the maximum element.
The functions top() and pop() both need the maximum value in the vec- lare a boolean data member recomputeMaxIndex, and initialize it to false. The functions top() and pop() use recomputeMaxIndex to avoid unnecessary calls to findMaxIndex().
(iv) The function top() returns the maximum value in pqVector. The function top() first checks to see whether the priority queue is empty. In that case, throw the exception underflowError. A second check looks at recomputeMaxIndex. If it is true, call findMaxIndex(), assign its return value to maxIndex, and set re- computeMaxIndex to false. In either case, top() returns the element pqVec- tor[maxIndex].
next steps are in the pictures then the code below is the last step.
int findMaxIndex() const;
// find the index of the maximum value in pqVector int maxIndex;
// index of the maximum value
bool recomputeMaxIndex;
// do we need to compute the index of the maximum element?
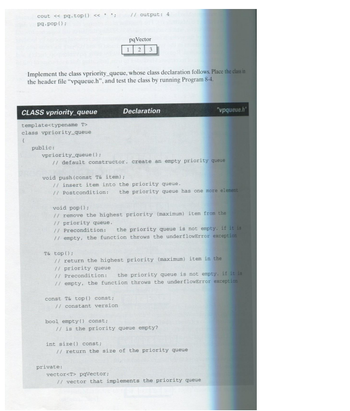
![(v) If recomputeMaxIndex is true, the function pop() finds the maximum element
by calling findMaxIndex(). The function must remove the highest priority item
from the vector, which vacates a position. Sliding the tail of the sequence toward
the front of the vector is inefficient. Implement the removal process by locating
the last element in the vector (pqVector.back()) and copying its value into the
vacated position. Remove the last element by using pqVector.pop_back(), and
set the value of recomputeMaxIndex to true, indicating that a subsequent call to
top() or pop() requires a new maximum.
The figures show the sequence of values in pqVector as push() and pop() operations ex-
ecute for the vpriority_queue object pq.
int arr [5] (6, 2, 3, 5, 3);
vpriority_queue<int> pq:
int i;
for (i=0;i< 5; i++)
pq.push(arr [i]);
cout << pq.top() << " ";
pq.pop();
6
cout << pq.top() << "
pq.pop();
pq.push (10);
pq.push (1);
4
cout << pq.top() << " ";
pq.pop();
4
pqVector
2 3 5 4
// output: 6
pq Vector
2 3 5
// output: 5
pqVector
4 2 3
pq Vector
4 2 3 10
pqVector
2 3 10 1
// output: 10.
pq Vector
4 2 3 1](https://content.bartleby.com/qna-images/question/c4b4111f-0cb3-46a3-bff1-74a667fd5dd6/15aeb8bb-3d74-404c-9abb-bfd90ffb3f4a/1yerno9_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 4 images

- Assuming you have a c-string variable "location" with a size of 30, write a line of code that allows you to read in an entire line of input to fill the variable. When we say that vectors are an example of a template class, what do we mean? Provide two unique vector declarations to illustrate this. What is the difference between the find and find_first_of string member functions?arrow_forwardEvery data structure that we use in computer science has its weaknesses and strengthsHaving a full understanding of each will help make us better programmers!For this experiment, let's work with STL vectors and STL dequesFull requirements descriptions are found in the source code file Part 1Work with inserting elements at the front of a vector and a deque (30%) Part 2Work with inserting elements at the back of a vector and a deque (30%) Part 3Work with inserting elements in the middle, and removing elements from, a vector and a deque (40%) Please make sure to put your code specifically where it is asked for, and no where elseDo not modify any of the code you already see in the template file This C++ source code file is required to complete this problemarrow_forwardObjectives: The code for the different stack and queue operations in both implementations (array and linked list) are discussed in the lectures: and are written in the lectures power point. So the main object of this assignment is to give the student more practice to increase their understanding of the different implementation of these operations. - The students also are asked to write by themselves the main methods in the different exercises below; The Lab procedures: The following files must be distributed to the students in the Lab - arrayImpOfStack.java // it represents an array implementation of the stack. - pointerImOfStack.java // it represents a Linked List implementation of the stack. - pointerImOfQueue.java // it represents a pointer implementation of the queue. Then the students by themselves are required to write the code for the following questions Ex1) Given the file arrayImpOfStack.java then write a main method to read a sequence of numbers and using the stack…arrow_forward
- Solve using c++. You are supposed to construct a class named Matrix that shall contains private data member matrix: integer type array of size 3 by 3;Define a constructor that should have default parameters that can set all elements to 0. Moreover,define a function called setMatrixValues(int matrixArray[3][3]) that can assign values ofmatrixArray (provided to the function in argument) to the matrix array (data member of the classMatrix) of the class. Along with it, define a function called displayMatrix() that can display allvalues of the matrix. Moreover, you are instructed to construct a program that can perform operator overloading forthe following operators + (plus), - (minus), and == (equal).arrow_forwardDesign and implement a class representing a circular sorted linked list. The class must have the following requirements: The linked list and the nodes must be implemented as C++ templates First must point to the largest value in the list It must include a constructor, a destructor, a copy constructor and an operator= It must include functions to insert a given item, delete a given item, search for a given item, check if the list is empty, return the length of the list and print the list (from smallest to largest) Hint: Use the menu-driven program of the linked list to test all the functions in your class.arrow_forwardWrite a program that will sort a prmiitive array of data using the following guidelines - DO NOT USE VECTORS, COLLECTIONS, SETS or any other data structures from your programming language. The codes for both the money and currency should stay the same, the main focus should be on the main file code (Programming language Java) Create a helper function called 'RecurInsSort' such that: It is a standalone function not part of any class from the prior lab or any new class you feel like creating here, Takes in the same type of parameters as any standard Insertion Sort with recursion behavior, i.e. void RecurInsSort(Currency arr[], int size) Prints out how the array looks every time a recursive step returns back to its caller The objects in the array should be Money objects added or manipulated using Currency references/pointers. It is OK to print out the array partially when returning from a particular step as long as the process of sorting is clearly demonstrated in the output. In…arrow_forward
- Implement a class representing a circular sorted linked list. The class must have the following requirements: The linked list and the nodes must be implemented as C++ templates First must point to the largest value in the list It must include a constructor, a destructor, a copy constructor and an operator= It must include functions to insert a given item, delete a given item, search for a given item, check if the list is empty, return the length of the list and print the list (from smallest to largest) Hint: Use the menu-driven program of the linked list to test all the functions in your class. Screenshot the output and make comments on each area on the program.arrow_forwardImplement in C Programing 7.10.1: LAB: Product struct Given main(), build a struct called Product that will manage product inventory. Product struct has three data members: a product code (string), the product's price (double), and the number count of product in inventory (int). Assume product code has a maximum length of 20. Implement the Product struct and related function declarations in Product.h, and implement the related function definitions in Product.c as listed below: Product InitProduct(char *code, double price, int count) - set the data members using the three parameters Product SetCode(char *code, Product product) - set the product code (i.e. SKU234) to parameter code void GetCode(char *productCode, Product product) - return the product code in productCode Product SetPrice(double price, Product product) - set the price to parameter product double GetPrice(Product product) - return the price Product SetCount(int count, Product product) - set the number of items in inventory…arrow_forward<< Data Structures >> << JAVA >>arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
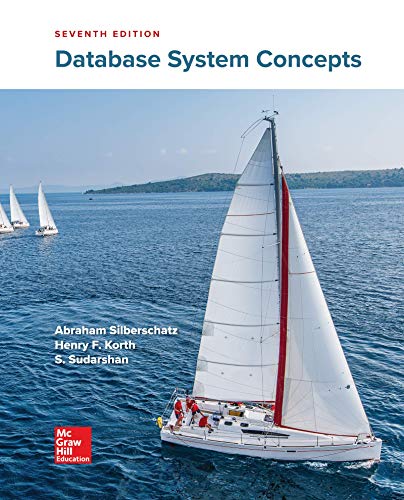
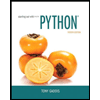
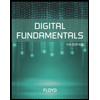
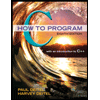
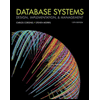
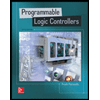