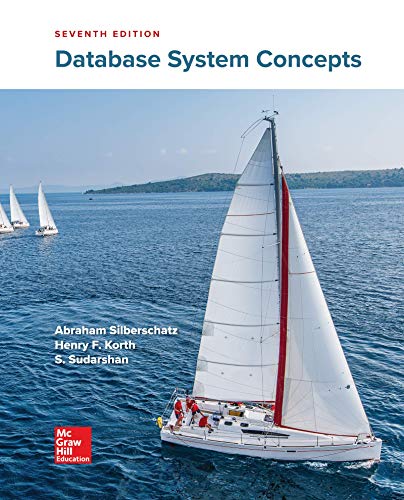
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
I need to write a java program called Fibonacci.java that prints the first n numbers of the Fibonacci sequence (starting with 0), where n is an integer entered by the user. Using a “for loop”. Some of the program has been provided below. Output should look like attached picture
import java.util.Scanner;
public class Fibonacci {
public static void main(String[] args) {
Scanner in = new Scanner(System.in); // Setting up scanner
System.out.print("Enter an integer: ");
int num = in.nextInt();
in.close(); // Close scanner when done using it
long current = 1;
long previous = 0;
// Add for loop logic here
}
}
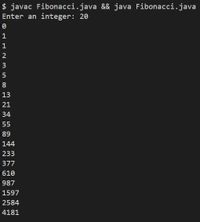
Transcribed Image Text:The image shows a terminal session demonstrating the compilation and execution of a Java program, presumably named `Fibonacci.java`, which calculates and displays the Fibonacci sequence up to a specified number. Here is a transcription and detailed explanation:
```
$ javac Fibonacci.java && java Fibonacci.java
Enter an integer: 20
0
1
1
2
3
5
8
13
21
34
55
89
144
233
377
610
987
1597
2584
4181
```
### Explanation:
1. **Command Execution**:
- The command `$ javac Fibonacci.java && java Fibonacci.java` is executed in a command-line interface. This command compiles the Java source file `Fibonacci.java` using the Java Compiler (`javac`). If the compilation is successful, the `&&` operator ensures that the Java Virtual Machine (`java`) runs the program.
2. **Program Input**:
- The program prompts the user to "Enter an integer:”. The user inputs the integer `20`.
3. **Fibonacci Sequence Output**:
- The program prints the Fibonacci sequence, starting from `0`, up to the 20th number in the sequence.
- The Fibonacci sequence is a series of numbers where the next number is found by adding up the two numbers before it. Starting with 0 and 1, the sequence for 20 numbers is:
- 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987, 1597, 2584, 4181.
This program is useful for educational purposes to demonstrate how loops or recursion can be employed to calculate mathematical sequences in programming.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Similar questions
- Java, the user selects an item from the text files ranging 1-15, repeats the loop until 'q'. the program provides a list of what they ordered including discounts, then calculates the total bill and how much they saved. The bottom is my the code I'm working on class BillProcessor {public static void prepareBill(LinkedHashMap<String, Integer> purchases, LinkedHashMap<String,Double>items,LinkedHashMap<String,String> sales, ArrayList<String> itemNames){double totalCost, totalDiscount, actualCost, discount; //note actualCost is the price of a single itemtotalCost = 0.00;totalDiscount = 0.00;actualCost = 0.00;discount = 0.00;for(Map.Entry<String,Integer>set: purchases.entrySet()) {if (sales.get(set.getKey()) != null) {if (sales.get(set.getKey()).equals("bogo") == true) {actualCost = set.getValue() * items.get(set.getKey());totalCost += actualCost;System.out.println("Item Name : " + set.getKey() + "\tItem Quantity(bogo) : " + (set.getValue() * 2 + "\tItem Cost :…arrow_forwardI could really use some help with a problem I got for coding. A screenshot showing an example would help.arrow_forwardPlease help me comment these lines of code. Please not these are just some lines of code that have been taken from the java program I am creating which is a coin toss program. I do not need you to create one. Just comment what the lines of code below private static String sideUp; } public void toss() { Random in = new Random(); int x = in.nextInt(); if (x % 2 == 0) sideUp = "Heads"; else sideUp = "Tails"; } public static void main(String[] args) { int tail = 0, head = 0; System.out.println("\nTossing coin 20 times:-"); for (int i = 1; i <= 20; i++) { coin.toss(); if (coin.getFace().equals("tails")) tail++; else head++;arrow_forward
- How do I code: public static void rotateElements(int[] arr, int rotationCount) public static void reverseArray(int[] arr) In Java?arrow_forwardneed help finishing/fixing this java code so it prints out all the movie names that start with the first 2 characters the user inputs the code is as follows: Movie.java: import java.io.File;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Scanner;public class Movie{public String name;public int year;public String genre;public static ArrayList<Movie> loadDatabase() throws FileNotFoundException {ArrayList<Movie> result=new ArrayList<>();File f=new File("db.txt");Scanner inputFile=new Scanner(f);while(inputFile.hasNext()){String name= inputFile.nextLine();int year=inputFile.nextInt();inputFile.nextLine();String genre= inputFile.nextLine();Movie m=new Movie(name, year, genre);//System.out.println(m);result.add(m);}return result;}public Movie(String name, int year, String genre){this.name=name;this.year=year;this.genre=genre;}public boolean equals(int year, String genre){return this.year==year&&this.genre.equals(genre);}public String…arrow_forwardJAVA Language: Transpose Rotate. Question: Modify Transpose.encode() so that it uses a rotation instead of a reversal. That is, a word like “hello” should be encoded as “ohell” with a rotation of one character. (Hint: use a loop to append the letters into a new string) import java.util.*; public class TestTranspose { public static void main(String[] args) { String plain = "this is the secret message"; // Here's the message Transpose transpose = new Transpose(); String secret = transpose.encrypt(plain); System.out.println("\n ********* Transpose Cipher Encryption *********"); System.out.println("PlainText: " + plain); // Display the results System.out.println("Encrypted: " + secret); System.out.println("Decrypted: " + transpose.decrypt(secret));// Decrypt } } abstract class Cipher { public String encrypt(String s) { StringBuffer result = new…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
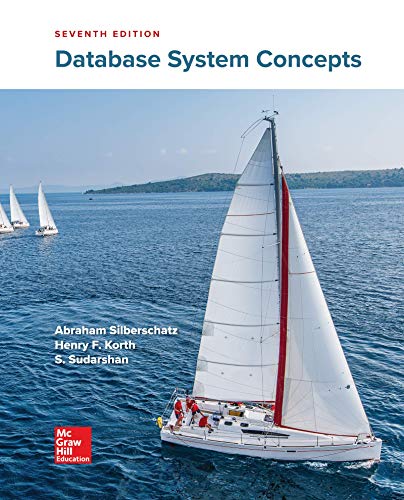
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
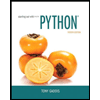
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
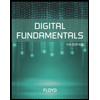
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
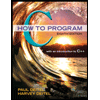
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
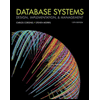
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
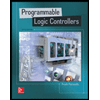
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education