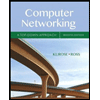
Implement in ARM7 assembly language a max-heap. In the main
function, open a file to read a sequence of unsorted integers. For the first input integer,
create a root node (12 bytes), holding the integer in the first 4 bytes and two empty
pointers, one for the left child node and the other for the right child node. For each of the
remaining input integers, build a node N to hold the number (X) and insert the node to the
tree such that
(1) any descending nodes of N contain integers smaller than X and ancestral nodes of
N contain integers equal or larger than X. Therefore, if X is larger than the
root.data, then node N will become the new root and the old root becomes N's
left child.
(2) when N is inserted below a node M (because N.data < M.data), follow the
following order:
a. if M has two empty children, N is inserted as the left child of M;
b. if M has only one empty child, for example the right child is empty, then
N becomes the right child;
c. if M has no empty children, N is inserted as M's the left child and M's
original left child becomes N's right child.
Implement the insert function with the following specification: Insert (heap, node),
where heap is a pointer to the root node, and node is the node to be inserted. The Insert
function should return the pointer to the root.
Implement deleteMax function with the following specification: deleteMax(heap), where
heap is a pointer to the root node of the heap. The deleteMax function should do the
following:
(1) return the maximum value stored in the heap, which is the root.data;
(2) maintain the heap structure by recursively (i.e., continuously) percolating the
vacancy down the binary tree to a leaf node and then removing the leaf node
whose data is elevated to the parent node. Note: your deleteMax function does
not have to be a recursive function.
data | Null or a Pointer to the left child node | Null or a Pointer to the right child node |

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- Recall the Stack ADT. It has the following operations: push(item): adds item to the top of the stack pop() : removes and returns the top item in the stack peek() : returns the top item in the stack (but does NOT remove it) size() : returns the number of items in the queue Write pseudocode for a function called remove(S, item) that takes a stack S and a value called item as input. The function removes all occurrences of item in S and returns the number of occurrences. The order of items in the modified stack must be the same as their original ordering (but with all occurrences of item absent). You can ONLY use basic control flow structures and Stack operations. You can use multiple stacks if you wish. Examples: S:= empty stack S.push('a'), S.push('t'), S.push('a'), S.push('u'), S.push('p') assert: the stack S now looks like ['a', 't', 'a', 'u', 'p'] (where 'p' is the top value) n = remove(S, 'a') assert : n is 2 assert : the stack S now looks like ['t', 'u', 'p'] (where 'p' is the top…arrow_forwardSolve the problem in the imagearrow_forwardInsert locks into the following two functions for stack operations assuming the two functions are executed in two threads. int Push(int stack[ ], int *top, int new_element){ stack[top]= new_element; top++; return 0; } int Pop(int stack[ ], int *top, int *top_element){ if (top>0) { top_element= stack[top]; top--; return 0; } else return -1; \\ empty stack }arrow_forward
- 1. In a Linked stack implemented using singly linked list with pointer top pointing to top of the stack, which of the following statements, correctly push a node into a non-empty stack? Assume temp is the node to be pushed. temp = top; top->next = temp; %3! top->next = temp; temp = top %3D temp->next = top; top = temp %3D %3D top = temp; temp->next = top 2. You are given pointers to first and last nodes of a singly linked list, which of the following operations are dependent on the length of the linked list? O Insert a new element as a first element Add a new element to the end of the list Delete the first element Delete the last element of the listarrow_forwardComplete the following code. The goal is to implement the producer-consumer problem. You are expected to extend the provided C code to synchronize the thread operations consumer() and producer() such that an underflow and overflow of the queue is prevented. You are not allowed to change the code for implementing the queue operations, that is the code between lines 25 and 126 as shown in the Figure below. You must complete the missing parts between lines 226-261 as shown in the screenshot.arrow_forwardCreate a computer programme to arrange objects in a stack so that the smallest ones are on top. Although you are allowed to utilise an extra temporary stack, you are not allowed to transfer the components into another data structure (such an array). The following operations are supported by the stack: push, pop, peek, and is Empty.arrow_forward
- This program will use an array implementation of a linked list to keep an ascending sorted list of numbers. The input data consists of two fields: a single character and an integer value. The single character gets interpreted as one of the following: A - represents a value that is to be added to the linked listD - represents a value to be deleted from the linked listP - indicates that all values are to be printed in ascending order The character is only used to determine which process is to be executed; it should not be printed, entered into the linked list, or processed in any way. The main program should be mainly function calls. Process input data until end of file. All output should be sent to an output file and be appropriately formatted and labeled. Keep a running log of everything that is going on in the processing. Input file: A 54A -17A 32 A 81 A -30 A 18 P A 41 A 93 A 65 A 80 A 104 A -20 D 81 D 54 D -30 A 79 A 63 A 77 A –33 D 104 D -20 D 80 D 32 Parrow_forwardFor this assignment you need to write a parallel program in C++ using OpenMP for vector addition. Assume A, B, C are three vectors of equal length. The program will add the corresponding elements of vectors A and B and will store the sum in the corresponding elements in vector C (in other words C[i] = A[i] + B[i]). Every thread should execute approximately equal number of loop iterations. The only OpenMP directive you are allowed to use is: #pragma omp parallel num_threads(no of threads) The program should take n and the number of threads to use as command line arguments: ./parallel_vector_addition Where n is the length of the vectors and threads is the number of threads to be created. Pseudocode for Assignment mystart = myid*n/p; // starting index for the individual thread myend = mystart+n/p; // ending index for the individual thread for (i = mystart; i < myend; i++) // each thread computes local sum do vector addition // and later all local sums combined. As an input vector A,…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
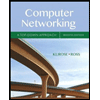
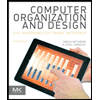
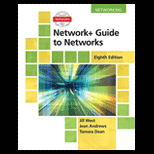
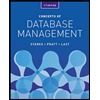
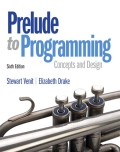
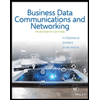