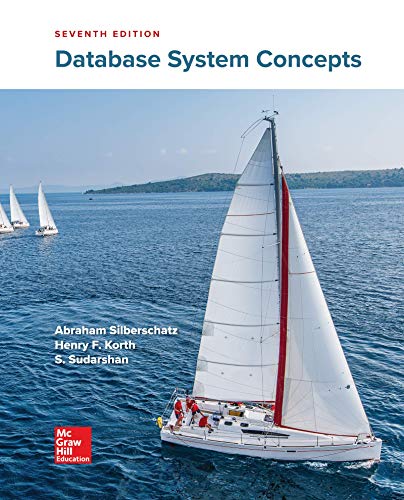
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
thumb_up100%
Complete the following code. The goal is to implement the producer-consumer problem. You are expected to extend the provided C code to synchronize the thread operations consumer() and producer() such that an underflow and overflow of the queue is prevented. You are not allowed to change the code for implementing the queue operations, that is the code between lines 25 and 126 as shown in the Figure below. You must complete the missing parts between lines 226-261 as shown in the screenshot.
![ܕ : ܘܢܬܬܢ ܪ ܪ ܪ ܪ . ܝ . . ܫ ܕ
13
#include <pthread.h>
14 #include <semaphore.h>
15 #include <errno.h>
16 #include <fcntl.h>
17 #define MAX_LENGTH_CAP 100 You can modify MAX_LENGTH_CAP
18
#define INIT -127
19 #define UNDERFLOW (0x80 + 0x02)
20 #define OVERFLOW 0x80+ 0x01
21 #define BADPTR (0x80 + 0x03)
22 #define CONSUMER_TERMINATION_PROBABILITY 40
23 #define PRODUCER_TERMINATION PROBABILITY 30
24
25 //
26
27
28 sem_t *FULLSPOTS;
29 sem_t *EMPTYSPOTS;
You can modify these two constants
for test and experimentation
//sem_t ACCESS; // use a mutex instead of a binary semaphore
pthread_mutex_t ACCESS = PTHREAD_MUTEX_INITIALIZER;
30 struct timespec remaining;
31 struct timespec requested_time;
32 > struct Queue{---
38
39
40> void enqueue (struct Queue *q, char x) -
65 > char dequeue(struct Queue *q) -
97 > void build(struct Queue **q, unsigned int length) ---
125
126 //
127
128 void *producer ( void *qptr ) ---
179
180 void *consumer( void *qptr) ...
225
226 void closeup (void);
227 int main(int argc, const char * argv[])--
308
309> void closeup (void) ...
LOCKED
UNLOCKED
Don't modify this code segment
TOTAL](https://content.bartleby.com/qna-images/question/f9be1d69-a222-4ca1-8ade-d83f4256e548/d7df4cf5-e557-4bfb-af1c-f6cefeeafaac/pptl0bf_thumbnail.png)
Transcribed Image Text:ܕ : ܘܢܬܬܢ ܪ ܪ ܪ ܪ . ܝ . . ܫ ܕ
13
#include <pthread.h>
14 #include <semaphore.h>
15 #include <errno.h>
16 #include <fcntl.h>
17 #define MAX_LENGTH_CAP 100 You can modify MAX_LENGTH_CAP
18
#define INIT -127
19 #define UNDERFLOW (0x80 + 0x02)
20 #define OVERFLOW 0x80+ 0x01
21 #define BADPTR (0x80 + 0x03)
22 #define CONSUMER_TERMINATION_PROBABILITY 40
23 #define PRODUCER_TERMINATION PROBABILITY 30
24
25 //
26
27
28 sem_t *FULLSPOTS;
29 sem_t *EMPTYSPOTS;
You can modify these two constants
for test and experimentation
//sem_t ACCESS; // use a mutex instead of a binary semaphore
pthread_mutex_t ACCESS = PTHREAD_MUTEX_INITIALIZER;
30 struct timespec remaining;
31 struct timespec requested_time;
32 > struct Queue{---
38
39
40> void enqueue (struct Queue *q, char x) -
65 > char dequeue(struct Queue *q) -
97 > void build(struct Queue **q, unsigned int length) ---
125
126 //
127
128 void *producer ( void *qptr ) ---
179
180 void *consumer( void *qptr) ...
225
226 void closeup (void);
227 int main(int argc, const char * argv[])--
308
309> void closeup (void) ...
LOCKED
UNLOCKED
Don't modify this code segment
TOTAL
![f
225
226 void closeup (void);
227 int main(int argc, const char * argv[])
228 {
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
pthread_t thread [6]; // declares the identifier for the thread we will create.
pthread_attr_t attr;
int iret [6];
int q_test_size = 5;
struct Queue *qptr = NULL;
build (&qptr, q_test_size);
(&attr);
closeup();
FULLSPOTS = (sem_t*) sem_open ("/FULLSPOTS", O_CREAT 10_EXCL, 0644,
if (
}
perror("sem_open");
exit (EXIT_FAILURE);
EMPTYSPOTS = sem_open("/EMPTYSPOTS", O_CREATIO_EXCL, 0644,
if
perror("sem_open");
exit (EXIT_FAILURE);
}
int x=0;
for(; x<5; x++) {
iret [x] = pthread_create(&thread [x], &attr, producer, qptr); // creat 5 producers
if (!iret [x]) // check for success
printf("PRODUCER_%d was created successfully.", x);
}
iret [x] = pthread_create(&thread [x], &attr, consumer, qptr); // ctreate one consumer
if (!iret [x])
printf("CONSUMER_%d was created successfully.", x);
for(int x=0; x<6; x++) // Wait for all threads; verify the for-loop
closeup ();
if (qptr->data) free(qptr->data);
if(qptr) free (qptr);
264
265
266
267
268
269> void closeup (void) --
printf("\n");
return 0;
& main
Ⓡ⁰ A⁰
Figure 2 You must complete the missing parts. between lines 226 - 261 as shown in this screenshot
> TOTAL](https://content.bartleby.com/qna-images/question/f9be1d69-a222-4ca1-8ade-d83f4256e548/d7df4cf5-e557-4bfb-af1c-f6cefeeafaac/7k3ogpn_thumbnail.png)
Transcribed Image Text:f
225
226 void closeup (void);
227 int main(int argc, const char * argv[])
228 {
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
pthread_t thread [6]; // declares the identifier for the thread we will create.
pthread_attr_t attr;
int iret [6];
int q_test_size = 5;
struct Queue *qptr = NULL;
build (&qptr, q_test_size);
(&attr);
closeup();
FULLSPOTS = (sem_t*) sem_open ("/FULLSPOTS", O_CREAT 10_EXCL, 0644,
if (
}
perror("sem_open");
exit (EXIT_FAILURE);
EMPTYSPOTS = sem_open("/EMPTYSPOTS", O_CREATIO_EXCL, 0644,
if
perror("sem_open");
exit (EXIT_FAILURE);
}
int x=0;
for(; x<5; x++) {
iret [x] = pthread_create(&thread [x], &attr, producer, qptr); // creat 5 producers
if (!iret [x]) // check for success
printf("PRODUCER_%d was created successfully.", x);
}
iret [x] = pthread_create(&thread [x], &attr, consumer, qptr); // ctreate one consumer
if (!iret [x])
printf("CONSUMER_%d was created successfully.", x);
for(int x=0; x<6; x++) // Wait for all threads; verify the for-loop
closeup ();
if (qptr->data) free(qptr->data);
if(qptr) free (qptr);
264
265
266
267
268
269> void closeup (void) --
printf("\n");
return 0;
& main
Ⓡ⁰ A⁰
Figure 2 You must complete the missing parts. between lines 226 - 261 as shown in this screenshot
> TOTAL
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Implement a simple linked list in Python (Write source code and show output) with basic linked list operations like: (a) create a sequence of nodes and construct a linear linked list. (b) insert a new node in the linked list. (b) delete a particular node in the linked list. (c) modify the linear linked list into a circular linked list. Use this template: class Node:def __init__(self, val=None):self.val = valself.next = Noneclass LinkedList:"""TODO: Remove the "pass" statements and implement each methodAdd any methods if necessaryDON'T use a builtin list to keep all your nodes."""def __init__(self):self.head = None # The head of your list, don't change its name. It shouldbe "None" when the list is empty.def append(self, num): # append num to the tail of the listpassdef insert(self, index, num): # insert num into the given indexpassdef delete(self, index): # remove the node at the given index and return the deleted value as an integerpassdef circularize(self): # Make your list circular.…arrow_forwardCreate an implementation of each LinkedList, Queue Stack interface provided For each implementation create a tester to verify the implementation of thatdata structure performs as expected Your task is to: Implement the LinkedList interface ( fill out the implementation shell). Put your implementation through its paces by exercising each of the methods in the test harness Create a client ( a class with a main ) ‘StagBusClient’ which builds a bus route by performing the following operations on your linked list: Create (insert) 4 stations List the stations Check if a station is in the list (print result) Check for a station that exists, and one that doesn’t Remove a station List the stations Add a station before another station. List the stations Add a station after another station. Print the stations StagBusClient.java package app; import linkedList.LinkedList; import linkedList.LinkedListImpl; public class StagBusClient { public static void main(String[] args) { // create…arrow_forwardDraw a memory map for the code you see on the next page, until the execution reaches the point indicated by the comment /* HERE */.In your diagram:• You must have a stack, heap, and static memory sections • Identify each frame as illustrated by the previous examples.• Draw your variables as they are encountered during program execution. Code: public class Passenger {private String name;private int ticketCost;private StringBuffer luggage;private static final int LUGGAGE_COST = 20;public Passenger(String name, int ticketCost) {this.name = name;this.ticketCost = ticketCost;luggage = new StringBuffer();}public Passenger addLuggage(String desc) {ticketCost += LUGGAGE_COST;luggage.append(desc);return this;}public int getTicketCost() {return ticketCost;}public Passenger reduceCost(int by) {ticketCost -= by;/* HERE */return this;}public String toString() {return "Passenger [name=" + name + ", ticketCost=" + ticketCost + ", luggage=" + luggage + "]";}}public class Driver {public static void…arrow_forward
- In a programming language (Pascal), the declaration of a node in a singly linked list is shown in Figure P6.7(a). The list referred to for the problem is shown in Figure P6.7(b). Given P to be a pointer to a node, the instructions DATA(P) and LINK(P) referring to the DATA and LINK fields, respectively, of node P are equivalently represented by P↑. DATA and P↑. LINK in the programming language. What do the following commands do to the logical representation of the list T? i) ii) TYPE POINTER=NODE; NODE RECORD DATA: integer; LINK: POINTER END; VAR P, Q R: T POINTER (a) Declaration of a node in a singly linked list T 31 TE 57 12 (b) A singly linked list T iii) R₁.LINK: = Q iv) R₁.DATA: = P₁.DATA: Q₁.DATA + R₁.DATA Q: = P |R Figure P6.7. (a and b) Declaration of a node in a programming language and the logical representation of a singly linked list T Q.LINK.DATA + 10 91 Linked Lists 189arrow_forwardWhat is the implementation of each instruction located in the main function of the following code? This is a sample program, i am trying to teach myself linked list and am having trouble ------------------------------------------------------------------------------- #include <iostream>#include <string> /*** node* * A node class used to make a linked list structure*/ template<typename type>struct node {node() : data(), next(nullptr) {}node(const type & value, node * link = nullptr) : data(value), next(link) {}type data;node * next;}; /*** print_list* @param list - the linked-list to print out* * Free function that prints out a linked-list space separated.* Templated so works with any node.* Use this for testing your code.*/ template<typename type>void print_list(node<type> * list) { for(node<type> * current = list; current != nullptr; current = current->next) {std::cout << current->data << ' ';}std::cout << '\n';} /***…arrow_forwardCreate a computer programme to arrange objects in a stack so that the smallest ones are on top. Although you are allowed to utilise an extra temporary stack, you are not allowed to transfer the components into another data structure (such an array). The following operations are supported by the stack: push, pop, peek, and is Empty.arrow_forward
- The program inserts a number of values into a priority queue and then removes them. The priority queue treats a larger unsigned integer as a higher priority than a smaller value. The priority queue object keeps a count of the basic operations performed during its lifetime to date, and that count is available via an accessor. In one or two places I have put statements such as op_count++;, but these are incomplete. We only count operations involved in inserting and removing elements, not auxiliary operations such as is_empty() or size() The class implements a priority queue with an unsorted vector. Your assignment is to re-implement the priority queue implementation using a heap built on a vector, exactly as we discussed in class. Leave the framework and public method interfaces completely unchanged. My original test program must work with your new class. You will have to write new private methods bubble_up and percolate_down. You should not implement heapify or heapsort. #include…arrow_forwardWrite a program for Stack (Array-based or linked list-based) in Python. Test the scenario below with the implementation and with the reasoning of the answer. Make comments with a short description of what is implemented. Include source codes and screen-captured outputs. Stack: A letter means doing a push operation and an asterisk means doing a pop operation in the below sequence. Give the sequence of letters that are returned by the pop operations when this sequence of operations is performed on an initially empty stack. A*BCE**F*GH***I*arrow_forwardYou will create two programs. The first one will use the data structure Stack and the other program will use the data structure Queue. Keep in mind that you should already know from your video and free textbook that Java uses a LinkedList integration for Queue. Stack Program Create a deck of cards using an array (Array size 15). Each card is an object. So you will have to create a Card class that has a value (1 - 10, Jack, Queen, King, Ace) and suit (clubs, diamonds, heart, spade). You will create a stack and randomly pick a card from the deck to put be pushed onto the stack. You will repeat this 5 times. Then you will take cards off the top of the stack (pop) and reveal the values of the cards in the output. As a challenge, you may have the user guess the value and suit of the card at the bottom of the stack. Queue Program There is a new concert coming to town. This concert is popular and has a long line. The line uses the data structure Queue. The people in the line are objects…arrow_forward
- This assignment will require you to implement a generic interface (Queue<T>) with an array based queue implementation class QueueArray<T>. floating front design. Once you have implemented the class, the test program will run a client test program on the enqueue, dequeue, display and full methods. The test program will create queues of Integers as well as String objects. Enjoying the power of your generic implementation both will work with no additional changes. There are TODO based comments in the implementation file. Create a project and demonstrate that you can successfully create the required output file, shown below. Thr file includes: Queue.java package queueHw; public interface Queue <T> { public boolean empty(); public boolean full(); public boolean enqueue(T data); public T dequeue(); public void display(); } ... TestQueue.java package queueHw; public class TestQueue { public static void main(String[] args) { //TEST QueueArray with…arrow_forwardYour crazy boss has assigned you to write a linear array-based implementation of the IQueue interface for use in all the company's software. You've tried explaining why that's a bad idea with logic and math, but to no avail. So - suppress your inner turmoil and complete the add() and remove() methods of the AQueue class and identify the runtime order of each of your methods. /** A minimal queue interface */ public interface IQueue { /** Add element to the end of the queue */ public void add(E element); /** Remove and return element from the front of the queue * @returns fırst element * @throws NoSuchElementException if queue is empty */ public E remove(); /** Not an ideal Queue */ public class AQueue implements IQueue{ private El) array: private int rear; public AQueue(){ array = (E[)(new Object[10]): rear = 0: private void expandCapacity) { if (rear == array.length) { array = Arrays.copyOf(array, array.length 2): @Override public void add(E element) { / TODO @Override public E…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
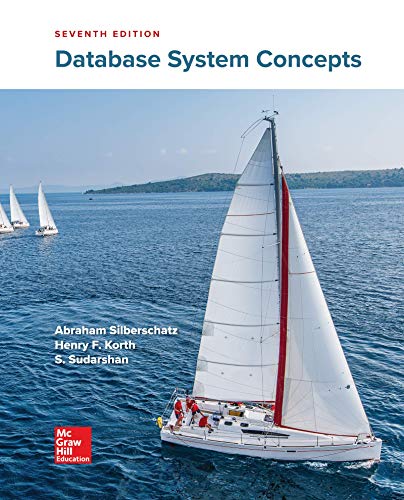
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
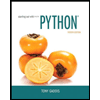
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
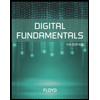
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
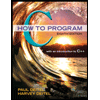
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
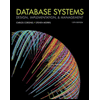
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
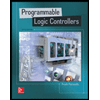
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education