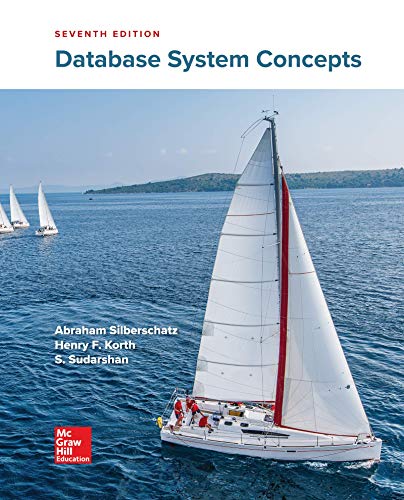
Concept explainers
Implement in C Programming
10.11.1: LAB: All permutations of names
Given a main program that reads the number of one word names followed by the list of names, complete the recursive function PrintAllPermutations() to list all ways people can line up for a photo (all permutations of an array of strings). Function PrintAllPermutations() takes 4 parameters: an array of a permutation of names, the number of names in the array of the permutation of names, an array of available names, and the number of names in the array of available names. Function PrintAllPermutations() then creates and outputs all possible orderings of those names separated by a comma, one ordering per line.
When the input is:
3 Julia Lucas Mia
then the output is (must match the below ordering):
Julia, Lucas, Mia
Julia, Mia, Lucas
Lucas, Julia, Mia
Lucas, Mia, Julia
Mia, Julia, Lucas
Mia, Lucas, Julia
(please add commas in code please)
#include <stdlib.h>
#include <stdio.h>
#include <string.h>
const int MAX_NAME_SIZE = 50;
// TODO: Write function to create and output all permutations of the list of names.
void PrintAllPermutations(char **permList, int permSize, char **nameList, int nameSize) {
}
int main(void) {
int size;
int i = 0;
char name[MAX_NAME_SIZE];
scanf("%d", &size);
char *nameList[size];
char *permList[size];
for (i = 0; i < size; ++i) {
nameList[i] = (char *)malloc(MAX_NAME_SIZE);
scanf("%s", name);
strcpy(nameList[i], name);
}
PrintAllPermutations(permList, 0, nameList, size);
// Free dynamically allocated memory
for (i = 0; i < size; ++i) {
free(nameList[i]);
}
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- Question: First write a function mulByDigit :: Int -> BigInt -> BigInt which takes an integer digit and a big integer, and returns the big integer list which is the result of multiplying the big integer with the digit. You should get the following behavior: ghci> mulByDigit 9 [9,9,9,9] [8,9,9,9,1] Your implementation should not be recursive. Now, using mulByDigit, fill in the implementation of bigMul :: BigInt -> BigInt -> BigInt Again, you have to fill in implementations for f , base , args only. Once you are done, you should get the following behavior at the prompt: ghci> bigMul [9,9,9,9] [9,9,9,9] [9,9,9,8,0,0,0,1] ghci> bigMul [9,9,9,9,9] [9,9,9,9,9] [9,9,9,9,8,0,0,0,0,1] ghci> bigMul [4,3,7,2] [1,6,3,2,9] [7,1,3,9,0,3,8,8] ghci> bigMul [9,9,9,9] [0] [] Your implementation should not be recursive. Code: import Prelude hiding (replicate, sum)import Data.List (foldl') foldLeft :: (a -> b -> a) -> a -> [b] -> afoldLeft =…arrow_forwardWrite a RECURSIVE function, without using any loops, that prints the contents of a matrix with 3 columns. The function should take the matrix and the number of rows as arguments. void print_matrix(int arr[][3], int num_rows);arrow_forward1. The sorted values array contains 16 integers 5, 7, 10, 13, 13, 20, 21, 25, 30,32, 40, 45, 50, 52, 57, 60. Indicate the sequence of recursive calls that are made tobinaraySearch, given an initial invocation of binarySearch(32, 0, 15).show only the recursive calls. For example, initial invocation is binarySearch(45,0,15)where the target is 45, first is 0 and last is 15.arrow_forward
- In C++ please and thank you!arrow_forwardImplement in C Programming 6.11.2: Modify an array parameter. Write a function SwapArrayEnds() that swaps the first and last elements of the function's array parameter. Ex: sortArray = {10, 20, 30, 40} becomes {40, 20, 30, 10}. #include <stdio.h> /* Your solution goes here */ int main(void) { const int SORT_ARR_SIZE = 4; int sortArray[SORT_ARR_SIZE]; int i; int userNum; for (i = 0; i < SORT_ARR_SIZE; ++i) { scanf("%d", &sortArray[i]); } SwapArrayEnds(sortArray, SORT_ARR_SIZE); for (i = 0; i < SORT_ARR_SIZE; ++i) { printf("%d ", sortArray[i]); } printf("\n"); return 0;}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
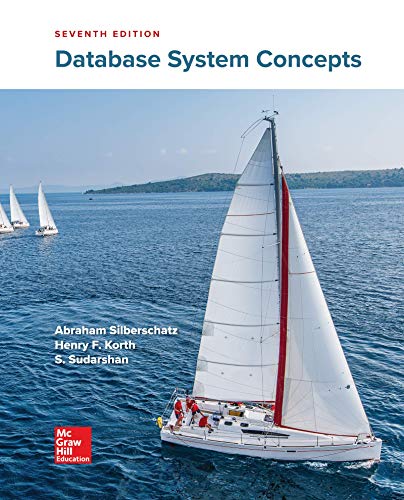
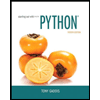
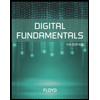
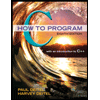
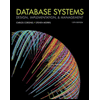
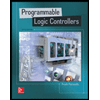