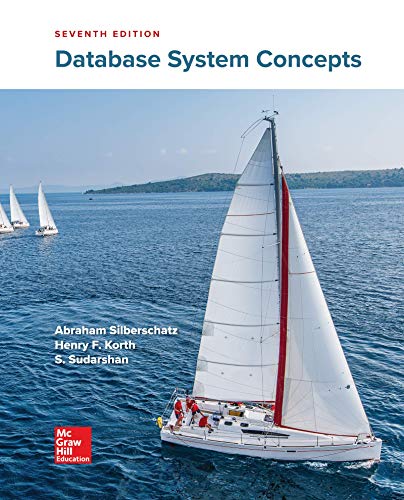
Concept explainers
Implement the following function, without using any data structure or #include <bits/stdc++.h>
/* Given two
e.g., V1=[‘a’,’b’,’a’] and V2=[‘b’,’a’,’a’] stores same multi-set of data points: i.e., both contains two ‘a’, and one ‘b’.
e.g., V3=[‘a’,’c’,’t’,’a’] and V4=[‘a’,’c’,’t’] are not same multi-set. V3 contains two ‘a’s, while V4 has only one ‘a’.
Note: when considering multiset, the number of occurrences matters. @param list1, list2: two vectors of chars
@pre: list1, list2 have been initialized
@post: return true if list1 and list2 stores same values (in same or different order); return false, if not. */
bool SameMultiSet (vector<char> list1, vector<char> list2)
THIS IS NOT THE CORRECT SOLUTION
/ include headers
#include <bits/stdc++.h>
// deinfe the namespace
using namespace std;
// function fo rchecking same
bool checkSame(vector<int> arr,vector<int> arr1)
{
// define a flag variables
int flag=0;
// loop from 0 to size of arr
for(int i=0;i<arr.size();i++)
{
// initialize flag=0
flag=0;
// loop from 0 to size of arr1
for(int j=0;j<arr1.size();j++)
{
// if arr[i] is equal to arr[j]
if(arr[i]==arr1[j])
{
// make flag as 1
flag=1;
// remove arr1[j] from arr1
vector<int>::iterator it;
it = arr1.begin()+j;
arr1.erase(it);
// break and move to next element
break;
}
}
// if flag is still 0
// return 0
if(flag==0)
{
return 0;
}
}
// return 1 after comparing
return 1;
}
// main function for testing
int main()
{
// call function and print answer accordinly
checkSame({1,2,3,4,5},{5,4,3,2,1})?cout<<The vectors are same:cout<<The vectors are not same;
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps

- What is the big O notation for this function?arrow_forwardPlease code in C.arrow_forwardCan you help me write a C++ program to do the following: Create a function append(v, a, n) that adds to the end of vector v a copy of all the elements of array a. For example, if v contains 1, 2, 3, and a contains 4, 5, 6, then v will end up containing 1, 2, 3, 4, 5,6. The argument n is the size of the array. The argument v is a vector ofintegers and a is an array of integers. Write a test driverarrow_forward
- Write a C++ Program that does the following: Implement a sort function using a vector.Ask the user to enter numbers to be sorted. Add them to the vector. The user will signal the end of input bygiving you a negative number.Then, sort the vector. Because this is a vector, you don’t need to pass the number of entriesin the vector.Then, print out the vector in order.Remember the following things about vectors.a) You declare a vector v of ints by saying vector⟨int⟩ v;b) You add elements to a vector by saying v.push back(thing to be added);c) You access elements of a vector with [ ] just like an array;d) One of the vector member functions is .length()arrow_forwardIn C++, Can you please look at the code below and revise/fix so it will work according to instructions and criteria. Instruction 1) Write a function that copies a 1D array to a 2D array. The function’s prototype is bool copy1DTo2D(int d1[], int size, int d2[][NCOLS], int nrows); where size > 0 NCOLS > 0 nrows > 0 NCOLS is a global constant size = NCOLS * nrows the function returns true if the parameters and constants satisfy these conditions and false otherwise. the relation between 1d array indices and 2d array indices is 2d row index = 1d array index / NCOLS 2d column index = 1d array index modulus operator NCOLS 2) Write a function that copies a 2D array to a 1D array. The function’s prototype is bool copy2DTo1D(int d2[][NCOLS, int nrows, int d1[], int size); where size > 0 NCOLS > 0 nrows > 0 NCOLS is a global constant the function return true if the parameters and constants satisfy these conditions and false otherwise. the relation between 1d array indices and…arrow_forwardUse pointers to write a function that finds the larg- est element in an array of integers. Use {6, 7, 9, 10, 15, 3, 99, -21} to test the function.arrow_forward
- Please use C++ and make sure it's for a sorted array Write a function, removeAll, that takes three parameters: an array of integers,the number of elements in the array, and an integer (say, removeItem). Thefunction should find and delete all of the occurrences of removeItem in thearray. If the value does not exist or the array is empty, output an appropriatemessage. (Note that after deleting the element, the number of elements in thearray is reduced.) Assume that the array is sorted.arrow_forwardCreate a function append(v, a, n) that adds to the end of vector v a copy of all the elements of array a. For example, if v contains 1,2, 3, and a contains 4, 5, 6, then v will end up containing 1, 2, 3, 4, 5,6. The argument n is the size of the array. The argument v is a vector ofintegers and a is an array of integers. Write a test driver.arrow_forwarda. Problem 1. Create an array of 30 random numbers that range between 1 and 100. Then, write a function that will receive a number from the user and determine if that number exists in the array or not. For instance, assume the array is: [2, 93, 14, 89, 12, 3, 81, 15, 14, 89, 52, 96, 71, 82, 5, 2, 41, 23, 52, 59, 44, 44, 88, 39, 49, 50, 97, 45, 48, 36] Now, assume the user enters 89, the program should output true. But, if the user enters 77, the program should output false. Approach: We will be implementing this method in two different ways. Both will be recursive. First, implement a method called findA (x,A), where x is the number we are looking for and A is an array. In the body of the function, compare x with the FIRST item that is in the array. If this first item is equal to X, return true. If not, remove the first item from A and call findA (x,A) on the revised list. If you call find on an empty list, you will want to return false. Writing any explicit loop in your code results a…arrow_forward
- Write a function that verifies if a given number exists in an array of floats. The function is supposed to return the first position in where the number is encountered. If the given number does not exist, the function returns –1. Then write a complete program that asks the user to enter an array of 8 floats and calls the function. The prototype of the function should be like: int Search (floats a[ ], int n, float number); Example: Consider the following array of floats int x[8]={2.1, 5.0, 9.8, 4.4, 6.8, 12.88, 9.87, 4.56}; If the number to be searched is 5.4, the function returns –1 If the number to be searched is 9.8,the function returns 2 C++ language only.arrow_forwardProduce the following program.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
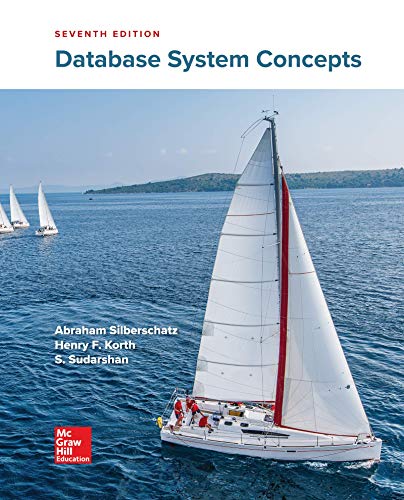
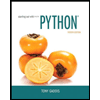
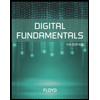
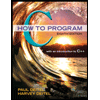
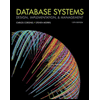
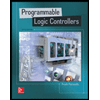