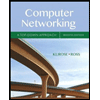
import 'dart:io';
import 'module.dart';
List<Module> modules = [];
void main() {
int? choice = 1;
while (choice != 5) {
choice = menu();
if (choice != null && choice >= 1 && choice <= 5) {
switch (choice) {
case 1:
createFromExisting();
break;
case 2:
printModuleData();
break;
case 3:
changeWeights();
break;
case 4:
printAverageOfClass();
break;
}
} else {
print('Invalid choice, please choose again');
choice = -1;
}
}
print('Good bye!');
}
void createFromExisting() {
//30 marks
//This method retrieves the data from the TPG316C.csv file to create a new Module
//
//Steps:
//1. Ask the user to enter a name for the new module
//2. Read the name
//3. Ask the user to enter the name of the file to read module data from (we will use TPG316C.csv)
//4. Read the name of the file
//5. Read the lines from the file into a List<String>
//6. Create a new Module instance with empty fields, except for the name of the Module
//7. Use the first element in the List<String> created in step 5 to extract the heading (use split method)
// The first 2 values is the FirstName and LastName headers. Everything after that will be the names of marks.
// The names for this file is Sem1Mark and Sem2Mark. Make provision for any number of marks. Save the mark name
// into the markNames (List<String>) field of the new Module instance.
//8. Remove the first element of the List created in step 5 to remove the header. You are then left with only
// student data.
//9. Run a loop through the data of the List created in step 5:
// - Add a Student object to the students field (List<Student>) in the new Module instance.
// - Create an empty Map<String, double> map to represent the specific student's marks
// - Add the student's marks in the map as follows:
// { Sem1Mark : 100, Sem2Mark : 100 }
// - Add the map to the marks field (List<Map<String, double>>) in the new Module instance.
//10. Run a loop through the markNames, created in step 7, and ask the user for the weight of every mark
// Add those weights to the weights field (List<double>) of the new Module instance.
//11. Add the new Module to the modules field (List<Module>) declared above the main method.
}
void printModuleData() {
//30 marks
//This method will print all info of the Module chosen
//
//Steps:
//1. List the available modules to choose from and ask the user to choose a module
//2. Print a column heading (Name, Surname ---> Sem1Mark--Sem2Mark--)
// Note that the heading could be different depending on the file you read from
//3. Run a loop through the students field of the Module chosen:
// - Print the student name and surname
// - Create a running total variable to sum the marks of the specific student after weights applied
// - e.g. mark = (sem1Mark * sem1Weight) / 100
// - Create a loop inside point 3's loop to run through the markNames field of the Module chosen:
// - get the marks from the map using the markName(s) as key
// - get the weight of the marks from the weights field of the Module chosen
// - work out the marks after the weights has been applied and add to the running total
// - print out the mark
// - Print the value of the running total (the average of the student's marks)
}
void changeWeights() {
//10 marks
//This method will change all weights of Marks to new weights
//Steps:
//1. Ask the user to choose a module to change the weights for
//2. Run a loop through the markNames field of the chosen Module
// - Ask the user to enter the weight of every mark
// - Change the weight in the weights field of the chosen Module
}
void printAverageOfClass() {
//10 marks
//This method will print the average mark for the class
//Steps:
//1. Ask the user for the Module to print the average
//2. Create a running total initialized to 0
//3. Run a loop through the students field of the Module chosen:
// - Create a running total variable to sum the marks of the specific student after weights applied
// - e.g. mark = (sem1Mark * sem1Weight) / 100
// - Run a loop through the markNames field of the Module chosen:
// - get the marks from the map using the markName(s)
// - get the weight of the marks
from the weights field of the Module chosen
// - work out the marks after the weights has been applied and add to the running total
// - Add the running total to the running total of step 2
}
// code reuse - 10 marks
// defensive programming techniques - 10 marks
int? menu() {
print('\n\nWelcome to our application!');
print('Menu: ');
print('1. Create new modules from existing data');
print('2. Print a module\'s marks');
print('3. Change weights');
print('4. Work out the average of the class');
print('5. Exit');
print('Please enter your choice: ');
return int.tryParse(stdin.readLineSync()!);
}
//place this class in its own file called module.dart
import 'student.dart';
class Module {
List<Student> students;
String moduleName;
List<String> markNames;
List<double> weights;
List<Map<String, double>> marks;
Module({
required this.students,
required this.moduleName,
required this.markNames,
required this.marks,
required this.weights,
});
}
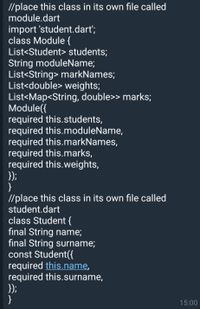

Step by stepSolved in 3 steps

- #include <iostream>using namespace std; class TurtleNode { public: TurtleNode(int babiesInit = 0, TurtleNode* nextLoc = nullptr); void InsertAfter(TurtleNode* nodeLoc); TurtleNode* GetNext(); void PrintNodeData(); private: int babiesVal; TurtleNode* nextNodePtr;}; TurtleNode::TurtleNode(int babiesInit, TurtleNode* nextLoc) { this->babiesVal = babiesInit; this->nextNodePtr = nextLoc;} void TurtleNode::InsertAfter(TurtleNode* nodeLoc) { TurtleNode* tmpNext = nullptr; tmpNext = this->nextNodePtr; this->nextNodePtr = nodeLoc; nodeLoc->nextNodePtr = tmpNext;} TurtleNode* TurtleNode::GetNext() { return this->nextNodePtr;} void TurtleNode::PrintNodeData() { cout << this->babiesVal << endl;} int main() { TurtleNode* headObj = nullptr; TurtleNode* firstTurtle = nullptr; TurtleNode* secondTurtle = nullptr; TurtleNode* currTurtle = nullptr; int babies1; int babies2; cin >> babies1; cin…arrow_forwardvoid getInput(){for(int i =0; i < studentName.length; i++){System.out.print("Student name: ");studentName[i] = keyboard.nextLine();System.out.print("Studnet ID: ");midTerm1[i] = keyboard.nextInt();}keyboard.close();} Can't put full student name becuase nextLine();arrow_forwardC++ complete and create magical square #include <iostream> using namespace std; class Vec {public:Vec(){ }int size(){return this->sz;} int capacity(){return this->cap;} void reserve( int n ){// TODO:// (0) check the n should be > size, otherwise// ignore this action.if ( n > sz ){// (1) create a new int array which size is n// and get its addressint *newarr = new int[n];// (2) use for loop to copy the old array to the// new array // (3) update the variable to the new address // (4) delete old arraydelete[] oldarr; } } void push_back( int v ){// TODO: if ( sz == cap ){cap *= 2; reserve(cap);} // complete others } int at( int idx ){ } private:int *arr;int sz = 0;int cap = 0; }; int main(){Vec v;v.reserve(10);v.push_back(3);v.push_back(2);cout << v.size() << endl; // 2cout << v.capacity() << endl; // 10v.push_back(3);v.push_back(2);v.push_back(3);v.push_back(4);v.push_back(3);v.push_back(7);v.push_back(3);v.push_back(8);v.push_back(2);cout…arrow_forward
- Check 1 ALLLEURE #include #include using namespace std; void PrintSize(vector numsList) { cout intList (2); PrintSize(intList); cin >> currval; while (currVal >= 0) { } Type the program's output intList.push_back(currval); cin >> currval; PrintSize(intList); intList.clear(); PrintSize(intList); return 0; CS Scanned with Calin canner Janviantars Input 12345-1 Output Feedback? 口口。arrow_forwardpublic class arrayOutput { public static void main (String [] args) { final int NUM_ELEMENTS = 3; int[] userVals = new int [NUM_ELEMENTS]; int i; int sumVal; userVals [0] = 3; userVals [1] = 5; userVals [2] = 8; Type the program's output sumVal = 0; for (i = 0; i < userVals.length; ++i) { sumVal= sumVal + userVals [i]; System.out.println (sumVal); } ? ? ??arrow_forwardclass temporary { public: void set(string, double, double); void print(); double manipulate(); void get(string&, double&, double&); void setDescription(string); void setFirst(double); void setSecond(double); string getDescription() const; double getFirst()const; double getSecond()const; temporary(string = "", double = 0.0, double = 0.0); private: string description; double first; double second; }; I need help writing the definition of the member function set so the instance varialbes are set according to the parameters. I also need help in writing the definition of the member function manipulation that returns a decimal with: the value of the description as "rectangle", returns first * second; if the value of description is "circle", it returns the area of the circle with radius first; if the value of the description is "cylinder", it returns the volume of the cylinder with radius first and height second; otherwise, it returns with the value -1.arrow_forward
- Q1 #include <stdio.h> int arrC[10] = {0}; int bSearch(int arr[], int l, int h, int key); int *joinArray(int arrA[], int arrB[]) { int j = 0; if ((arrB[0] + arrB[4]) % 5 == 0) { arrB[0] = 0; arrB[4] = 0; } for (int i = 0; i < 5; i++) { arrC[j++] = arrA[i]; if (arrB[i] == 0 || (bSearch(arrA, 0, 5, arrB[i]) != -1)) { continue; } else arrC[j++] = arrB[i]; } for (int i = 0; i < j; i++) { int temp; for (int k = i + 1; k < j; k++) { if (arrC[i] > arrC[k]) { temp = arrC[i]; arrC[i] = arrC[k]; arrC[k] = temp; } } } for (int i = 0; i < j; i++) { printf("%d ", arrC[i]); } return arrC; } int bSearch(int arr[], int l, int h, int key) { if (h >= l) { int mid = l + (h - l) / 2; if…arrow_forwardHi i need an implementation on the LinkListDriver and the LinkListOrdered for this output : Enter your choice: 1 Enter element: Mary List Menu Selections 1. add element 2_remove element 3.head element 4. display 5.Exit Enter your choice: 3 Element @ head: Mary List Menu Selections 1-add element 2-remove element 3_head element 4.display 5-Exit Enter your choice: 4 1. Mary List Menu Selections 1. add element 2. remove element 3.head element 4. display 5. Exit Enter your choice: LinkedListDriver package jsjf; import java.util.LinkedList; import java.util.Scanner; public class LinkedListDriver { public static void main(String [] args) { Scanner input = new Scanner(System.in); LinkedList list = new LinkedList(); int menu = 0; do { System.out.println("\nList menu selection\n1.Add element\n2.Remove element\n3.Head\n4.Display\n5.Exit"); System.out.println();…arrow_forwardQ1 function myFunc() { let a = 10; if (true) { Q3 } } let a = 5; console.log(a); Q4 console.log(a); Q2 const square = num => num * num; console.log(square(3) + 5); myFunc(); let x = 30; let y "200"; console.log(x+y); const nums = [10, 20, 8, 17]; nums.filter(e=> e > 10); console.log(nums); Q5 const nums = [30, 35, 42, 20, 15]; console.log(nums.every (num => num > 20)); January 15arrow_forward
- main.cc file #include <iostream>#include <memory> #include "customer.h" int main() { // Creates a line of customers with Adele at the front. // LinkedList diagram: // Adele -> Kehlani -> Giveon -> Drake -> Ruel std::shared_ptr<Customer> ruel = std::make_shared<Customer>("Ruel", 5, nullptr); std::shared_ptr<Customer> drake = std::make_shared<Customer>("Drake", 8, ruel); std::shared_ptr<Customer> giveon = std::make_shared<Customer>("Giveon", 2, drake); std::shared_ptr<Customer> kehlani = std::make_shared<Customer>("Kehlani", 15, giveon); std::shared_ptr<Customer> adele = std::make_shared<Customer>("Adele", 4, kehlani); std::cout << "Total customers waiting: "; // =================== YOUR CODE HERE =================== // 1. Print out the total number of customers waiting // in line by invoking TotalCustomersInLine. //…arrow_forward#include <iostream>using namespace std;class st{private:int arr[100];int top;public:st(){top=-1;}void push(int ItEM) {top++;arr[top]=ItEM; }bool ise() {return top<0;} int pop(){int Pop;if (ise()) cout<<"Stack is emptye ";else{Pop=arr[top];top--;return Pop;}}int Top(){int TOP;if (ise()) cout<<" empty ";else {TOP=arr[top];return TOP;}}void screen(){ for (int i = 0; i <top+1 ; ++i) {cout<<arr[i];} }};int main() {st c;c.push(1);c.push(2);c.push(3);c.push(4);c.pop();c.push(5);c.screen();return 0;} alternative to this code??arrow_forwardclass Artist{StringsArtist(const std::string& name="",int age=0):name_(name),age_(age){}std::string name()const {return name_;}void set_name(const std::string& name){name_=name;}int age()const {return age_;}void set_age(int age){age_=age;}virtual std::string perform(){return std::string("");}private:int age_;std::string name_;};class Singer : public Artist{public:Singer():Artist(){};Singer(const std::string& name,int age,int hits);int hits() const{return hits_;}void set_hits(int hit);std::string perform();int operator+(const Singer& rhs);int changeme(std::string name,int age,int hits);Singer combine(const Singer& rhs);private:int hits_=0;};int FindOldestandMostSuccess(std::vector<Singer> list); Task: Implement the function int Singer::changeme(std::string name,int age,int hits) : This function should check if the values passed by name, age and hits are different than those stored. And if this is the case it should change the values. This should be done by…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
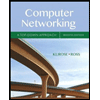
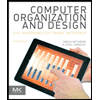
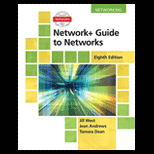
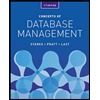
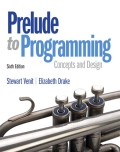
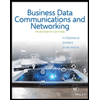