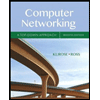
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
import numpy as np
import json
img_codes = np.load("data/image_codes.npy")
captions = json.load(open('data/captions_tokenized.json'))
for img_i in range(len(captions)):
for caption_i inrange(len(captions[img_i])):
sentence = captions[img_i][caption_i]
captions[img_i][caption_i] = ["#START#"] + sentence.split(' ') + ["#END#"]
class CaptionNet(nn.Module):
def__init__(self, n_tokens=n_tokens, emb_size=128, lstm_units=256, cnn_feature_size=2048):
""" A recurrent 'head' network for image captioning. See scheme above. """
super().__init__()
# a layer that converts conv features to initial_h (h_0) and initial_c (c_0)
self.cnn_to_h0 = nn.Linear(cnn_feature_size, lstm_units)
self.cnn_to_c0 = nn.Linear(cnn_feature_size, lstm_units)
# create embedding for input words. Use the parameters (e.g. emb_size).
# YOUR CODE HERE
# lstm: create a recurrent core of your network. Use either LSTMCell or just LSTM.
# In the latter case (nn.LSTM), make sure batch_first=True
# YOUR CODE HERE
# create logits: linear layer that takes lstm hidden state as input and computes one number per token
# YOUR CODE HERE
defforward(self, image_vectors, captions_ix):
"""
Apply the network in training mode.
:param image_vectors: torch tensor containing inception vectors . shape: [batch, cnn_feature_size]
:param captions_ix: torch tensor containing captions as matrix. shape: [batch, word_i].
padded with pad_ix
:returns: logits for next token at each tick, shape: [batch, word_i, n_tokens]
"""
self.lstm.flatten_parameters()
initial_cell = self.cnn_to_c0(image_vectors)
initial_hid = self.cnn_to_h0(image_vectors)
# compute embeddings for captions_ix
# YOUR CODE HERE
# apply recurrent layer to captions_emb.
# 1. initialize lstm state with initial_* from above
# 2. feed it with captions. Mind the dimension order in docstring
# 3. compute logits for next token probabilities
# Note: if you used nn.LSTM, you can just give it (initial_cell[None], initial_hid[None]) as second arg
# lstm_out should be lstm hidden state sequence of shape [batch, caption_length, lstm_units]
# YOUR CODE HERE
# compute logits from lstm_out
# YOUR CODE HERE
#for reference and more detail go to ---> https://colab.research.google.com/github/hse-aml/intro-to-dl-pytorch/blob/main/week06/week06_final_project_image_captioning.ipynb#scrollTo=x_wH1bYu-QK8
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Similar questions
- Clear Explanation on this question add some step to leading on how you answerd it neat handwriting and clear explanation table completed Question are below here:arrow_forwardHere is my entire code(average is not working yet). The response I got from the professor is that I have to create a DRY solution replacing the five display_functions with a single get_array function you call five times like common_array = get_array(plant_xml, "COMMON") etc. from urllib.request import urlopenfrom xml.etree.ElementTree import parse def read_data(data_file): plant_url = urlopen(data_file) plant_xml = parse(plant_url) return plant_xml def display_common_items(plant_xml): plant_root = plant_xml.getroot() common_array = [] for item in plant_root.findall("PLANT"): common_array.append(item.find("COMMON").text) return common_array def display_botanical_items(plant_xml): plant_root = plant_xml.getroot() botanical_array = [] for item in plant_root.findall("PLANT"): botanical_array.append(item.find("BOTANICAL").text) return botanical_array def display_zone_items(plant_xml): plant_root = plant_xml.getroot() zone_array = []…arrow_forwardDetermine if the following statement are true or false screen shot shows the text's SortedArrayCollection and ArrayCollection When comparing two objects using the == operator, what is actually compared is the references to the objects. When comparing two objects using the equals method inherited from the Object class, what is actually compared is the references to the objects. The text's sorted array-based collection implementation stores elements in the lowest possible indices of the array. The text's array-based collection implementation stores elements in the lowest possible indices of the array.arrow_forward
- import numpy as np%matplotlib inlinefrom matplotlib import pyplot as pltimport mathfrom math import exp np.random.seed(9999)def is_good_peak(mu, min_dist=0.8): if mu is None: return False smu = np.sort(mu) if smu[0] < 0.5: return False if smu[-1] > 2.5: return False for p, n in zip(smu, smu[1:]): #print(abs(p-n)) if abs(p-n) < min_dist: return False return True maxx = 3ndata = 500nset = 10l = []answers = []for iset in range(1, nset): npeak = np.random.randint(2,4) xs = np.linspace(0,maxx,ndata) ys = np.zeros(ndata) mu = None while not is_good_peak(mu): mu = np.random.random(npeak)*maxx for ipeak in range(npeak): m = mu[ipeak] sigma = np.random.random()*0.3 + 0.2 height = np.random.random()*0.5 + 1 ys += height*np.exp(-(xs-m)**2/sigma**2) ys += np.random.randn(ndata)*0.07 l.append(ys) answers.append(mu) p6_ys = lp6_xs =…arrow_forwardCode to ::::implement a SnapshotArray that supports the following interface: SnapshotArray(int length) initializes an array-like data structure with the given length. Initially, each element equals 0. void set(index, val) sets the element at the given index to be equal to val. int snap() takes a snapshot of the array and returns the snap_id: the total number of times we called snap() minus 1. int get(index, snap_id) returns the value at the given index, at the time we took the snapshot with the given snap_id Example 1: Input: ["SnapshotArray","set","snap","set","get"] [[3],[0,5],[],[0,6],[0,0]] Output: [null,null,0,null,5] Explanation: SnapshotArray snapshotArr = new SnapshotArray(3); // set the length to be 3 snapshotArr.set(0,5); // Set array[0] = 5 snapshotArr.snap(); // Take a snapshot, return snap_id = 0 snapshotArr.set(0,6); snapshotArr.get(0,0); // Get the value of array[0] with snap_id = 0, return 5...arrow_forwardDesign a class that acquires the JSON string from question #1 and converts it to a class data member dictionary. Your class produces data sorted by key or value but not both. Provide searching by key capabilities to your class. Provide string functionality to convert the dictionary back into a JSON string. question #1: import requestsfrom bs4 import BeautifulSoupimport json class WebScraping: def __init__(self,url): self.url = url self.response = requests.get(self.url) self.soup = BeautifulSoup(self.response.text, 'html.parser') def extract_data(self): data = [] lines = self.response.text.splitlines()[57:] # Skip the first 57 lines for line in lines: if line.startswith('#'): # Skip comment lines continue values = line.split() row = { 'year': int(values[0]), 'month': int(values[1]), 'decimal_date': float(values[2]),…arrow_forward
- Determine if the following statements is true or false. Screen shot shows the text's arraycollection and sortedarraycollection With the text's array-based collection implementation, the set of stored elements is scattered about the array. The text's sorted array-based collection implementation stores elements in the lowest possible indices of the array. When comparing two objects using the equals method inherited from the Object class, what is actually compared is the contents of the objects.arrow_forwardPython Code Create a code that can plot a distance versus time graph by importing matplotlib and appending data from a text file to a list. Follow the algorithm: Import matplotlib. Create two empty lists: Time = [ ] and Distance = [ ] Open text file named Motion.txt (content attached). Append data from Motion.txt such that the first column is placed in Time list and the second column is placed in Distance list. Plot the lists (Distance vs Time Graph). You may use this following link as a source for matplotlib functions: https://datatofish.com/line-chart-python-matplotlib/ Show Plot.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
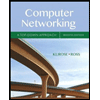
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
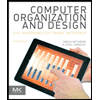
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
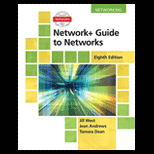
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
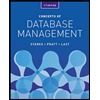
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
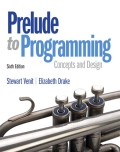
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
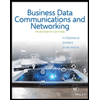
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY