In a doubly linked list the first class is Node which we can create a new node with a given element. Its constructor also includes previous node reference prev and next node reference next. make a node object for the new element. Check if the index >= 0. If index is 0, make new node as head; else, make a temp node and iterate to the node previous to the index. If the previous node is not null, adjust the prev and next references. Print a message when the previous node is null. implement these 3 methods: insert_at_index(), delete_at_end(), display(). use these three functions above use this to name the items
In a doubly linked list the first class is Node which we can create a new node with a given element. Its constructor also includes previous node reference prev and next node reference next.
make a node object for the new element. Check if the index >= 0.
If index is 0, make new node as head; else, make a temp node and iterate to the node previous to the index.
If the previous node is not null, adjust the prev and next references. Print a message when the previous node is null.
implement these 3 methods: insert_at_index(), delete_at_end(), display(). use these three functions above
use this to name the items
1. insert_to_empty_list()
2. insert_to_end()
3. insert_at_index()
4. delete_at_start()
5. delete_at_end() .
6. display()
let the output be
OUTPUT:
The list is empty
Element is: 3
Element is: 10
Element is: 20
Element is: 30
Element is: 35
Element is: 38
Element is: 40
Element is: 50
Element is: 60
Element is: 10
Element is: 20
Element is: 30
Element is: 35
Element is: 38
Element is: 40
Element is: 50

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

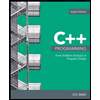
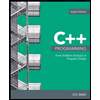