In C language, Write a function that gets a string containing a positive integer. The function subtracts 1 from that integer and puts the obtained value in the string. void str_subtract_one(char* num); For example: - if before we call str_subtract_one(str) we have str==“1997”, then after return str will be “1996”. - if before we call str_subtract_one(str) we have str==“12345678987650”, then after return str will be “12345678987649”. - if before we call str_subtract_one(str) we have str==“100”, then after return str will be “99”. 1. You may assume that the input is always legal, i.e., the string is a positive integer correctly formatted. 2. Note that the numbers may be larger than the maximum of int or long. That is, you should not try to convert string to int
In C language, Write a function that gets a string containing a positive integer. The function subtracts 1 from
that integer and puts the obtained value in the string.
void str_subtract_one(char* num);
For example:
- if before we call str_subtract_one(str) we have str==“1997”, then after return str will be “1996”.
- if before we call str_subtract_one(str) we have str==“12345678987650”, then after return str will be “12345678987649”.
- if before we call str_subtract_one(str) we have str==“100”, then after return str will be “99”.
1. You may assume that the input is always legal, i.e., the string is a positive integer correctly formatted.
2. Note that the numbers may be larger than the maximum of int or long. That is, you should not try to convert string to int

Step by step
Solved in 4 steps with 4 images

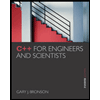
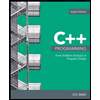
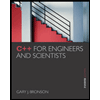
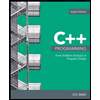