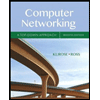
Exercise: Dir and Help
Learn about the methods Python provides for strings. To see what methods Python provides for a datatype, use the dir and help commands:
>>> s = 'abc'
>>> dir(s)
['__add__', '__class__', '__contains__', '__delattr__', '__doc__', '__eq__', '__ge__', '__getattribute__', '__getitem__', '__getnewargs__', '__getslice__', '__gt__', '__hash__', '__init__','__le__', '__len__', '__lt__', '__mod__', '__mul__', '__ne__', '__new__', '__reduce__',
'__reduce_ex__','__repr__', '__rmod__', '__rmul__', '__setattr__', '__str__', 'capitalize', 'center', 'count', 'decode', 'encode', 'endswith', 'expandtabs', 'find', 'index', 'isalnum', 'isalpha', 'isdigit', 'islower', 'isspace', 'istitle', 'isupper', 'join', 'ljust', 'lower', 'lstrip', 'replace', 'rfind','rindex', 'rjust', 'rsplit', 'rstrip', 'split', 'splitlines', 'startswith', 'strip', 'swapcase', 'title', 'translate', 'upper', 'zfill']
>>> help(s.find)
Help on built-in function find:
find(...) method of builtins.str instance
S.find(sub[, start[, end]]) -> int
Return the lowest index in S where substring sub is found, such that sub is contained within S[start:end]. Optional arguments start and end are interpreted as in slice notation.
Return -1 on failure.
>> s.find('b')
- Try out some of the string functions listed in dir (ignore those with underscores '_' around the method name).

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 4 images

- numpy.ipynb 1. addToArray(i) def addToArray(i): # TO DO %time addToArr(10) 2. a function def findDriver(distanceArr, driversArr, customerArr): result = '' ### put your code here return result print(findDriver(locations, drivers, cust)) # this should return Clara 3. The Amazing 5D Music example array([[ 1, 3, -2, -4, -1], [ 0, -1, 1, -1, -2], [ 2, 3, -2, -3, -1], [ 1, -1, 0, -1, -3], [-2, -1, 1, -1, -3], [ 1, 3, -1, -2, -3]]) ``` # TODO array([11, 5, 11, 6, 8, 10]) # TODO array([1, 3, 4, 5, 0, 2]) # TODO # TODO def findClosest(customers, customerNames, x): # TO DO return '' print(findClosest(customers, customerNames, mikaela)) # Should print Ben print(findClosest(customers, customerNames, brandon)) # Should print Ann 4. Numpy drones arr = np.array([-1, 2, -3, 4]) arr2 = np.square(arr) arr2 locations = np.array([[4, 5], [6, 6], [3, 1], [9,5]]) drones = np.array(["wing_1a", "wing_2a",…arrow_forwardIn Java, string method concat is used for __________ strings.arrow_forwardGiven the previous Car class, the following members have been added for you: Private: string * parts; //string array of part names int num_parts; //number of parts Public: void setParts(int numpart, string newparts[]) //set the numparts and parts int getNumParts() string * getParts() string getPart(int index) //return the string in parts at index num Please implement the following for the Car class: Add the copy constructor. (deep copy!) Add the copy assignment operator. (deep copy!) Add the destructorarrow_forward
- Q# Describe what a data structure is for a char**? (e.g. char** argv) Group of answer choices A. It is a pointer to a single c-string. B. It is an array of c-strings C. It is the syntax for dereferencing a c-string and returning the value.arrow_forwardclass Artist{StringsArtist(const std::string& name="",int age=0):name_(name),age_(age){}std::string name()const {return name_;}void set_name(const std::string& name){name_=name;}int age()const {return age_;}void set_age(int age){age_=age;}virtual std::string perform(){return std::string("");}private:int age_;std::string name_;};class Singer : public Artist{public:Singer():Artist(){};Singer(const std::string& name,int age,int hits);int hits() const{return hits_;}void set_hits(int hit);std::string perform();int operator+(const Singer& rhs);int changeme(std::string name,int age,int hits);Singer combine(const Singer& rhs);private:int hits_=0;};int FindOldestandMostSuccess(std::vector<Singer> list); Task: Implement the function int Singer::changeme(std::string name,int age,int hits) : This function should check if the values passed by name, age and hits are different than those stored. And if this is the case it should change the values. This should be done by…arrow_forwardclass Artist{StringsArtist(const std::string& name="",int age=0):name_(name),age_(age){}std::string name()const {return name_;}void set_name(const std::string& name){name_=name;}int age()const {return age_;}void set_age(int age){age_=age;}virtual std::string perform(){return std::string("");}private:int age_;std::string name_;};class Singer : public Artist{public:Singer():Artist(){};Singer(const std::string& name,int age,int hits);int hits() const{return hits_;}void set_hits(int hit);std::string perform();int operator+(const Singer& rhs);int changeme(std::string name,int age,int hits);Singer combine(const Singer& rhs);private:int hits_=0;};int FindOldestandMostSuccess(std::vector<Singer> list); Implement the function Singer Singer::combine(const Singer& rhs):It should create a new Singer object, by calling the constructor with the following values: For name it should pass a combination of be the name of the calling object followed by a '+' and then…arrow_forward
- // FILE: DPQueue.h// CLASS PROVIDED: p_queue (priority queue ADT)//// TYPEDEFS and MEMBER CONSTANTS for the p_queue class:// typedef _____ value_type// p_queue::value_type is the data type of the items in// the p_queue. It may be any of the C++ built-in types// (int, char, etc.), or a class with a default constructor, a// copy constructor, an assignment operator, and a less-than// operator forming a strict weak ordering.//// typedef _____ size_type// p_queue::size_type is the data type considered best-suited// for any variable meant for counting and sizing (as well as// array-indexing) purposes; e.g.: it is the data type for a// variable representing how many items are in the p_queue.// It is also the data type of the priority associated with// each item in the p_queue//// static const size_type DEFAULT_CAPACITY = _____// p_queue::DEFAULT_CAPACITY is the default initial capacity of a// p_queue that is created by the default…arrow_forward#include <stdio.h>#include <stdlib.h>#include <time.h> struct treeNode { struct treeNode* leftPtr; int data; struct treeNode* rightPtr;}; typedef struct treeNode TreeNode;typedef TreeNode* TreeNodePtr; void insertNode(TreeNodePtr* treePtr, int value);void inOrder(TreeNodePtr treePtr);void preOrder(TreeNodePtr treePtr);void postOrder(TreeNodePtr treePtr); int main(void) { TreeNodePtr rootPtr = NULL; srand(time(NULL)); puts("The numbers being placed in the tree are:"); for (unsigned int i = 1; i <= 10; ++i) { int item = rand() % 15; printf("%3d", item); insertNode(&rootPtr, item); } puts("\n\nThe preOrder traversal is: "); preOrder(rootPtr); puts("\n\nThe inOrder traversal is: "); inOrder(rootPtr); puts("\n\nThe postOrder traversal is: "); postOrder(rootPtr);} void insertNode(TreeNodePtr* treePtr, int value) { if (*treePtr == NULL) { *treePtr = malloc(sizeof(TreeNode)); if…arrow_forward#include <stdio.h>#include <stdlib.h>#include <time.h> struct treeNode { struct treeNode *leftPtr; int data; struct treeNode *rightPtr;}; typedef struct treeNode TreeNode;typedef TreeNode *TreeNodePtr; void insertNode(TreeNodePtr *treePtr, int value);void inOrder(TreeNodePtr treePtr);void preOrder(TreeNodePtr treePtr);void postOrder(TreeNodePtr treePtr); int main(void) { TreeNodePtr rootPtr = NULL; srand(time(NULL)); puts("The numbers being placed in the tree are:"); for (unsigned int i = 1; i <= 10; ++i) { int item = rand() % 15; printf("%3d", item); insertNode(&rootPtr, item); } puts("\n\nThe preOrder traversal is: "); preOrder(rootPtr); puts("\n\nThe inOrder traversal is: "); inOrder(rootPtr); puts("\n\nThe postOrder traversal is: "); postOrder(rootPtr);} void insertNode(TreeNodePtr *treePtr, int value) { if (*treePtr == NULL) { *treePtr = malloc(sizeof(TreeNode)); if (*treePtr != NULL) { (*treePtr)->data = value;…arrow_forward
- // FILE: IntSet.h - header file for IntSet class// CLASS PROVIDED: IntSet (a container class for a set of// int values)//// CONSTANT// static const int MAX_SIZE = ____// IntSet::MAX_SIZE is the highest # of elements an IntSet// can accommodate.//// CONSTRUCTOR// IntSet()// Pre: (none)// Post: The invoking IntSet is initialized to an empty// IntSet (i.e., one containing no relevant elements).//// CONSTANT MEMBER FUNCTIONS (ACCESSORS)// int size() const// Pre: (none)// Post: Number of elements in the invoking IntSet is returned.// bool isEmpty() const// Pre: (none)// Post: True is returned if the invoking IntSet has no relevant// relevant elements, otherwise false is returned.// bool contains(int anInt) const// Pre: (none)// Post: true is returned if the invoking IntSet has anInt as an// element, otherwise false is returned.// bool isSubsetOf(const IntSet& otherIntSet) const//…arrow_forwardIn C++ Assume that a 5 element array of type string named boroughs has been declared and initialized. Write the code necessary to switch (exchange) the values of the first and last elements of the array.arrow_forwardThe C++ Vertex class represents a vertex in a graph. The class's only data member is _____ Ⓒlabel 1000 O weight O framEdges O toEdges Question 36 The C++ Graph class's fromEdges member is a(n) _________ O string Ⓒdouble O vector O unordered_map Question 37 The C++ Graph class's AddVertex() function's return type is _____ void O Edge+ Overtex) O vectorarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
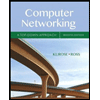
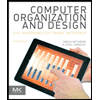
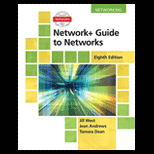
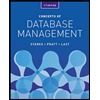
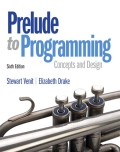
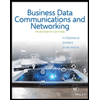