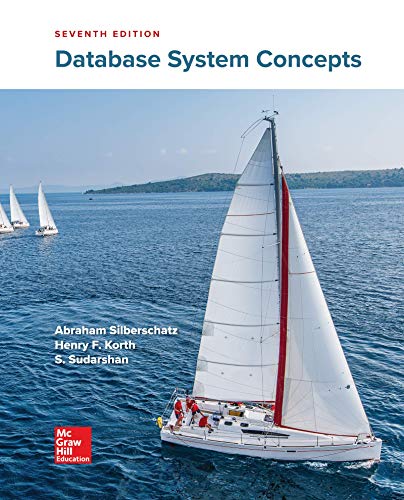
in C#
In Chapter 2, you created an interactive application named GreenvilleRevenue.
The program prompts a user for the number of contestants entered in this year’s and last year’s Greenville Idol competition, and then it displays the revenue expected for this year’s competition if each contestant pays a $25 entrance fee.
The programs also display a statement that compares the number of contestants each year. Using the program you wrote in Case Study 1 of Chapter 2, replace that statement with one of the following messages:
If the competition has more than twice as many contestants as last year, display The competition is more than twice as big this year!
If the competition is bigger than last year’s but not more than twice as big, display The competition is bigger than ever!
If the competition is smaller than last year’s, display, A tighter race this year! Come out and cast your vote!
In order to prepend the $ to currency values, the program will need to use the CultureInfo.GetCultureInfo method. In order to do this, include the statement using System.Globalization; at the top of your program and format the output statements as follows: WriteLine("This is an example: {0}", value.ToString("C", CultureInfo.GetCultureInfo("en-US")));

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- For this lab, you will be doing the following in C#: 1) Create a Home form with buttons that when clicked, will open the Account, Email, and eventually the Contact forms. The Account form should be opened in "View" mode. 2) Add code to the Login form so the Account form opens when the user clicks "Create New Account". The Account form should be opened in "Modify" mode. 3) Add code to the Login form that validates the user input before allowing the user the access the Home form. For now, just verify the user enters "user1" and "12345" for the username and password respectively.arrow_forwardObject-Oriented Programming ❤❤❤ Matchmaker with Java ❤❤❤ Summary: Create a Java application that will determine your true love. Prerequisites: Java, VS Code, and Terminal In this assignment you will develop and test a command-line application written in the Java language that asks questions to determine if a person is your true love. More specifically your application should ask five questions in the form of statements and allow the user to respond to each statement with the numbers 1 through 5 with 1 indicating strongly disagree and 5 indicating strongly agree. You will then compare the person’s answers with your desired “true love” answers. The closer the answers are to your desired “true love” answers the better match the two of you are for each other. For example, suppose you choose the statement “Broccoli is delicious.” and your desired answer was 1 (strongly disagree) because you really don’t like broccoli. If the application user entered 4 (agree), then the two of you would not…arrow_forwardDon't use AI.arrow_forward
- Attributes item_name (string) item_price (int) item_quantity (int) Default constructor Initializes item's name="none", item's price = 0, item's quantity=0 Method print_item_cost() Ex of print_item_cost() output: Bottled Water 10 @ $1 = $10 in the main section of your code, prompt the user for two items and create two objects of the ItemToPurchase class. Exc Item 1 Enter the item name: Chocolate Chips Enter the item price: 3 Enter the item quantity: 1 Item 2 Enter the item name: Bottled Water Enter the item price: 1 Enter the item quantity: 10 Add the costs of the two items together and output the total cost Ex TOTAL COST Chocolate Chips 1 @ $3-$3 Bottled Water 10 @ $1= $10 Total: $13arrow_forwardModule main() // Local variables Constant Integer SIZE = 7 Declare Integer employee[SIZE] = 56588, 45201, 78951, 87775, 84512, 13028, 75804 Declare Integer hoursWork[SIZE], index Declare Real payRate[SIZE], wages[SIZE], totalWages = 0 // Get name For index = 0 to SIZE - 1 Display “For employee number “, employee[index], “: “ Display “Enter hours: “ Input hoursWork[index] Display “Enter pay rate: “ Input payRate[index] Set wages[index] = hoursWork[index] * payRate[index] Set totalWages = totalWages + wages[index] End For // show gross wages For index = 0 to SIZE – 1 Display “For employee number “, employee[index], “, wages “,wages[index] End For Display “Total wages “,totalWages End Module Flowchart pleasearrow_forwardIn Java code: A company pays its employees on a weekly basis. The company has three types of employees: Salaried employees, who are paid a fixed weekly salary regardless of the number of hours worked; Hourly employees, who are paid by the hour and receive overtime pay; Commissioned employees whose pays are 20% of the week's sales Create a console-based object-oriented Java application that:Calculates weekly pay for an employee. The application should display text that requests the user input the name of the employee, type of employee, and the monthly salary, or hourly rate, if it’s an hourly employee, and hours worked for the week. For hourly employees, the rate will be doubled if it’s beyond 40 hours/week.The application should then print out the name of the employee and the weekly pay amount. In the printout, display the dollar symbol ($) to the left of the weekly pay amount and format the weekly pay amount to display currency. Implements a feature that allows the company to…arrow_forward
- Computer Science Part C: Interactive Driver Program Write an interactive driver program that creates a Course object (you can decide the name and roster/waitlist sizes). Then, use a loop to interactively allow the user to add students, drop students, or view the course. Display the result (success/failure) of each add/drop.arrow_forwardC++ program Write a method that displays information about a movie. The method accepts the movie title, running time in minutes and the release year as arguments. Provide a default value for the running time so that if you call the method without minutes, it defaults to 90. Write a main() method that demonstrates you can call the method with two or three arguments. Save the file as Movie.cpparrow_forwardIt is not sufficient to declare a variable by using "type" alone. A variable may be identified by the data type it uses as well as other properties. The next obvious step is to figure out how to utilize this concept to describe any particular variable?arrow_forward
- object oriented programming need the full code as soon as possible.arrow_forwardWrite a program that records and displays league standings for a baseball league. The program will ask the user to enter five team names, and five win amounts. It will store the data in memory, and print it back out sorted by wins from highest to lowest. The sample output from your program should look something like this (user input in bold orange): Enter team #1: PadresEnter the wins for team #1: 75Enter team #2: DodgersEnter the wins for team #2: 91Enter team #3: GiantsEnter the wins for team #3: 92Enter team #4: Rockies Enter the wins for team #4: 65Enter team #5: DiamondbacksEnter the wins for team #5: 70League Standings:Giants: 92Dodgers: 91Padres: 75Diamondbacks: 70Rockies: 65 Requirements The data must be stored in two parallel arrays: an array of strings named teams, and an array of ints named wins. These arrays must be declared in the main() function. You can assume that the league has five teams, so each of the arrays should have five elements. As usual, you may not use any…arrow_forwardMadLib Game bigGame.py Write a program that plays a MadLib game with the user. The program should ask the user to enter the following words: A plural noun (PNOUN1) A person’s first name (FNAME) A noun (NOUN1) A person’s last name (LNAME) A second plural noun (PNOUN2) A place (PLACE1) A third plural noun (PNOUN3) Another place (PLACE2) A fourth plural noun (PNOUN4) A second noun (NOUN2) An adjective (ADJECTIVE1) A second adjective (ADJECTIVE2) A verb (VERB) A third adjective (ADJECTIVE3) After the user has entered these items, the program should display the following story, inserting the user’s input into the appropriate locations: Hello there, sports PNOUN1!This is FNAME, talking to you from the press NOUN1in LNAME Stadium, where 57,000 cheering PNOUN2Have gathered to watch (the) PLACE1 PNOUN3take on (the) PLACE2 PNOUN4.Even though the NOUN2 is shining, it’s a/an ADJECTIVE1cold day with the temperature in the ADJECTIVE2 20s.We’ll be back for the opening VERB -off after a few…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
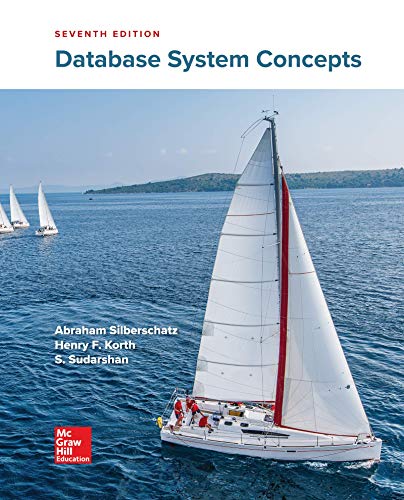
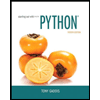
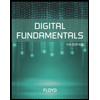
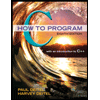
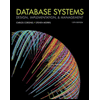
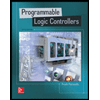