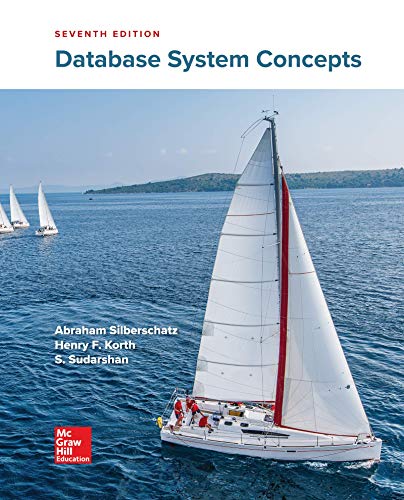
Concept explainers
In Computer Science a Graph is represented using an adjacency matrix. Is
matrix is a square matrix whose dimension is the total number of vertices.
The following example shows the graphical representation of a graph with 5 vertices, its matrix
of adjacency, degree of entry and exit of each vertex, that is, the total number of
arrows that enter or leave each vertex (verify in the image) and the loops of the graph, that is
say the vertices that connect with themselves
To program it, use Object Oriented
-Declare a constant V with value 5
-Declare a variable called Graph that is a VxV matrix of integers
-Define a MENU procedure with the following text
GRAPHS
1. Create Graph
2.Show Graph
3. Adjacency between pairs
4.Input degree
5.Output degree
6.Loops
0.exit
-Validate MENU so that it receives only valid options (from 0 to 6), otherwise send an error message and repeat the reading
-Make the MENU call in the main program
-Define a CREATE procedure/function to fill an array with random values between 0 and 1. Where 0 represents no
adjacency and 1 indicates that there is.
-Make the CREATE call in the main program, receive as
parameter the Graph matrix created in point 2
-Define a SHOW procedure/function to print an adjacency matrix, in matrix form
-Make the SHOW call in the main program, receive as
parameter the Graph matrix created in point 2
- Define an ADJACENCY procedure/function that receives, apart from the array, two integer values and returns a value of true if there is a 1 in those positions of the array and False otherwise.
-Make the ADYACENCY call in the main program. To do this, ask and validate (between 1 and V) two vertices of the graph. Indicate if these two vertices are adjacent in the graph. That is, if ADYACENCY returns 1, write “Adjacency”, if they are not, say “Not adjacency”. Remember that the user is going to give values between 1 and V and that the matrix has values from 0 to V-1
-For the Output degree option, use the function/procedure that
add rows and do it with each of the rows. Remember that the user will see vertices between 1 and V and that the matrix has values from 0 to V-1
- The MENU keeps repeating until the user presses 0 (exit)
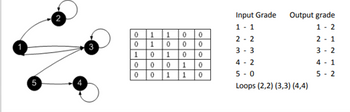

Step by stepSolved in 5 steps with 2 images

- P R O B L E M D E S C R I P T I O N :1. As described in the reading and lecture, an adjacency matrix for a graph with n verticesnumber 0, 1, …, n – 1 is an n by n array matrix such that matrix[i][j] is 1 (or true) ifthere is an edge from vertex i to vertex j, and 0 (or false) otherwise.2. In this assignment, you will implement the methods identified in the stub code below insupport of the Graph ADT that uses an adjacency matrix to represent an undirected,unweighted graph with no self-loops.import java.util.*; // for all needed JCF classespublic class Graph { private int[][] matrix;// the adjacency matrix of the graph. // Creates an n x n array with all values initialized to 0. public Graph(int n) {// your code here } // end constructor // This method returns the number of nodes in the graph. public int getNumVertices() {// your code here } // end getNumVertices // This method returns the number of edges in the graph. public int getNumEdges() {// your code here } // end getNumEdges //…arrow_forwardThe following image shows a graph with 7 vertices (nodes) labelled from 1 to 7 and edges connecting some of the vertices. 3 6 1 7 The adjacency matrix of a graph is the matrix A = (Aij) with Aij = 1 if there is an edge connecting the vertices i and j and Aij = 0 otherwise. Set up the adjacency matrix of the above graph and assign it to the variable adjacency_matrix. Represent the matrix as a list of lists such that adjacency_matrix[i-1][j-1] corresponds to Aij. Thereafter, define a function vdeg that takes an adjacency matrix (in the same format) and returns a list of the degrees of the vertices. The degree of a vertex is the number of other vertices that it is connected to. Use the function vdeg to determine the degrees of all vertices of the above graph and store the result in the variable example_degrees.arrow_forwardPick the correct statements for the attached directed graph for a toy world wide web. 1. There are exactly six columns having a 1 as an entry. 2. If a new edge from node I to F is added, the resulting graph is strongly connected. 3. The smallest non-zero element in the link matrix is 1/4. 4. The link matrix Q has a column of all zeros. 5. The link matrix is a reducible matrix.arrow_forward
- Please solve with the computer Question 2: Draw a simple undirected graph G that has 11 vertices, 7 edges.arrow_forwardGiven the following graph, show the adjacency matrix and adjacency list representations in the space below. 8 6 6 6 2. 00 9, 9, 3.arrow_forwardData Structures and Algorithmsarrow_forward
- In the following G, each adjacency list is sorted in increasing alphabetical order. B H Draw the component graph GSCC of G.arrow_forwardComputer science question helparrow_forwardGive an example of a graph that has all 3 of the following properties. (Note that you need to give a single graph as the answer.) (i) It is connected (ii) It has one articulation point. (iii) The graph needs at least 4 colors for a valid vertex coloring (iv) The graph does not have a 4-clique (that is, a clique of 4 vertices) as a subgraph.arrow_forward
- Assume you are to write a program to analyze the social connection between students in MTSU. Each student is a node in a undirected graph. An edge is added between two nodes if these two students have close social connections, i.e., in the same club, or in the same department. Which would be a better representation for this graph? Question 45 options: adjacency matrix representation adjacency list representationarrow_forwardWrite the adjacency matrix for the following graphs. b a C d farrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
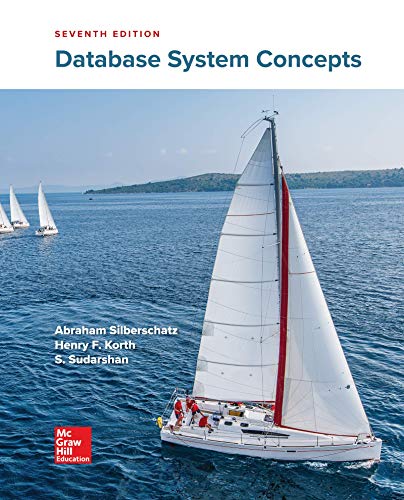
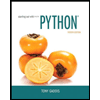
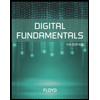
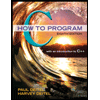
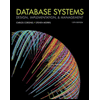
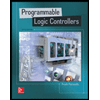