In Java Programming, 2 class needs a no-arg constructor and a constructor that initializes all fields with data (The ProductionWorker class constructor should receive data for the Employee class fields as well). I need help to create a method within the Employee and ProductionWorker classes called displayInfo. (displayInfo accepts no arguments and returns void). To display the data for each constructor, the demonstration class will call the subclass displayInfo method and it will call the superclass displayInfo method. User input is not required for the demonstration class. Have a class named "Employee" with the following fields: - Employee Name - Employee Number in format XXX-L, X = digit w/ range 0-9 & L = letter w/ range A-M. - Hire Date Then one or more constructors and the appropriate accessor and mutator methods for the class. Also, another class named "ProductionWorker" that extends "Employee" class. The "ProductionWorker" class should have the following fields to hold information, - Shift (an integer) -Hourly pay rate (a double) The workday is divided into 2 shifts: day and night. The shift field will be an integer value representing the shift that the employee works. The days shift is shift 1 and the night shift is shift 2. Like the first class, also create one or more constructors and the appropriate accessor and mutator methods for this class. Demonstrate the classes by writing a program that uses a ProductionWorker object.
In Java
Have a class named "Employee" with the following fields:
- Employee Name
- Employee Number in format XXX-L, X = digit w/ range 0-9 & L = letter w/ range A-M.
- Hire Date
Then one or more constructors and the appropriate accessor and mutator methods for the class.
Also, another class named "ProductionWorker" that extends "Employee" class. The "ProductionWorker" class should have the following fields to hold information,
- Shift (an integer)
-Hourly pay rate (a double)
The workday is divided into 2 shifts: day and night. The shift field will be an integer value representing the shift that the employee works. The days shift is shift 1 and the night shift is shift 2. Like the first class, also create one or more constructors and the appropriate accessor and mutator methods for this class.
Demonstrate the classes by writing a program that uses a ProductionWorker object.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

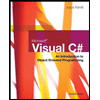
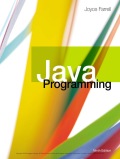
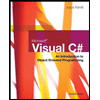
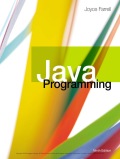