In this assignment, you will compare the performance of ArrayList and LinkedList. More specifically, your program should measure the time to “get” and “insert” an element in an ArrayList and a LinkedList. You program should 1. Initialize i. create an ArrayList of Integers and populate it with 100,000 random numbers ii. create a LinkedList of Integers and populate it with 100,000 random numbers 2. Measure and print the total time it takes to i. get 100,000 numbers at random positions from the ArrayList 3. Measure and print the total time it takes to i. get 100,000 numbers at random positions from the LinkedList 4. Measure and print the total time it takes to i. insert 100,000 numbers in the beginning of the ArrayList 5. Measure and print the total time it takes to i. insert 100,000 numbers in the beginning of the LinkedList 6. You must print the time in milliseconds (1 millisecond is 1/1000000 second). A sample run will be like this: Time for get in ArrayList(ms): 1 Time for get in LinkedList(ms): 4115 Time for insertion in ArrayList(ms): 1376 Time for insertion in LinkedList(ms): 7 II. Implementation Requirements You may use any implementation mechanism for random numbers and timers. The followings are suggested. • You can use java.util.Random class to create random numbers. • You can use the System.nanoTime() method to get the system timer in nanoseconds (1 nanosecond is 1/1000000000 second). • To measure the time of execution of some code, you need to call the timer before and after the execution. long startTime = System.nanoTime(); //code whose execution is measured long endTime = System.nanoTime(); long executionTime = (endTime - startTime); //in nanoseconds
In this assignment, you will compare the performance of ArrayList and LinkedList. More specifically, your
program should measure the time to “get” and “insert” an element in an ArrayList and a LinkedList.
You program should
1. Initialize
i. create an ArrayList of Integers and populate it with 100,000 random numbers
ii. create a LinkedList of Integers and populate it with 100,000 random numbers
2. Measure and print the total time it takes to
i. get 100,000 numbers at random positions from the ArrayList
3. Measure and print the total time it takes to
i. get 100,000 numbers at random positions from the LinkedList
4. Measure and print the total time it takes to
i. insert 100,000 numbers in the beginning of the ArrayList
5. Measure and print the total time it takes to
i. insert 100,000 numbers in the beginning of the LinkedList
6. You must print the time in milliseconds (1 millisecond is 1/1000000 second).
A sample run will be like this:
Time for get in ArrayList(ms): 1
Time for get in LinkedList(ms): 4115
Time for insertion in ArrayList(ms): 1376
Time for insertion in LinkedList(ms): 7
II. Implementation Requirements
You may use any implementation
• You can use java.util.Random class to create random numbers.
• You can use the System.nanoTime() method to get the system timer in nanoseconds (1
nanosecond is 1/1000000000 second).
• To measure the time of execution of some code, you need to call the timer before and after the execution.
long startTime = System.nanoTime();
//code whose execution is measured
long endTime = System.nanoTime();
long executionTime = (endTime - startTime); //in nanoseconds

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

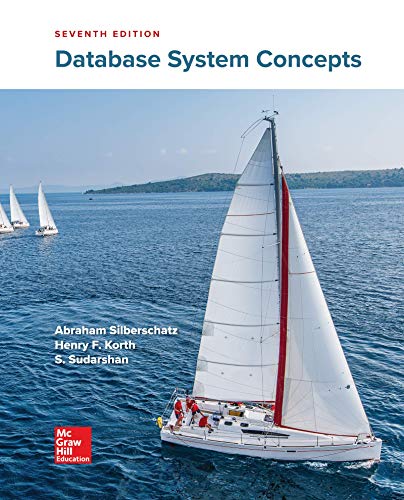
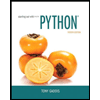
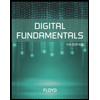
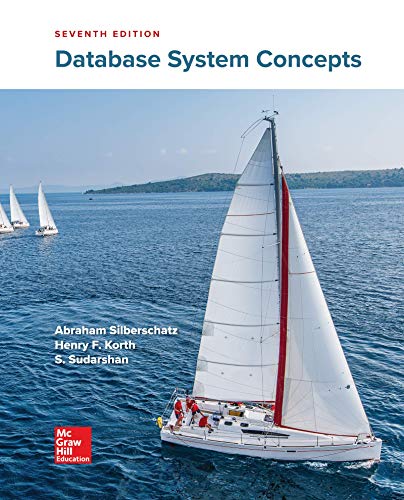
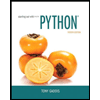
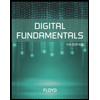
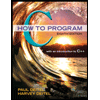
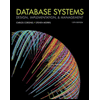
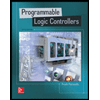