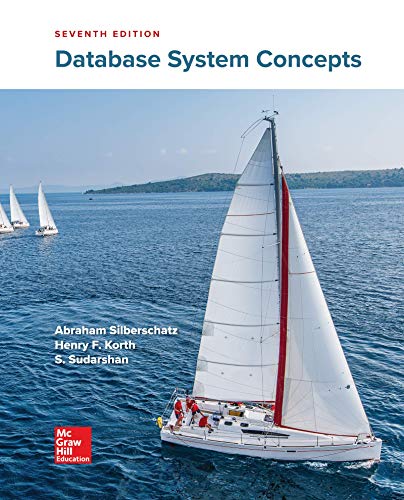
I am trying to compile a poker game:
#include <iostream>
#include <string>
#include <fstream>
#include <iomanip>
#include <sstream>
#include "card.h"
#include "deck.h"
#include "hand.h"
using namespace std;
/************************************************************
* FunctionName *
* Function description *
* *
* *
************************************************************/
int main()
{
string repeat = "Y";
Deck myDeck;
Hand myHand;
string exchangeCards;
while (repeat == "Y" || repeat == "y")
{
cout << endl;
myHand.newHand(myDeck);
myHand.print();
cout << endl;
cout << "Would you like to exchange any cards? [Y / N]: ";
getline(cin, exchangeCards);
while (exchangeCards != "Y" && exchangeCards != "y" && exchangeCards != "X" && exchangeCards != "n")
{
cout << "Please enter Y or N only: ";
getline(cin, exchangeCards);
}
if(exchangeCards = "Y" || exchangeCards = "y")
{
myHand.exchangeCards(myDeck);
}
cout << endl;
myHand.print();
cout << endl;
myDeck.reset(); // Resets the deck for a new game
cout << "Play again? [Y / N]: ";
getline(cin, repeat);
while (repeat != "Y" && repeat != "y" && repeat != "N" && repeat != "n")
{
cout << "Please enter Y or N only: ";
getline(cin, repeat);
}
}
return 0;
}
deck.h:
deck.h
#ifndef DECK_H
#define DECK_H
#include <
#include <cstdlib> // srand(), rand()
#include <ctime> // time()
#include "card.h" // Include card header file here
using namespace std;
class Deck
{
public:
// class Constructor
Deck();
// Reset deck to new state (completely undealt)
void resetDeck();
// Print all cards in the undealt deck
void printUndealtDeck();
// Print all cards in the dealt deck
void printDealtDeck();
// Get size of the undealt deck
const int getSizeUndealtDeck();
// Get size of the dealt deck
const int getSizeDealtDeck();
// Deal a single card
Card dealCard(); // Is the dealCard() here an accessor or mutator function???
private:
vector<Card> m_undealtDeck; // Undealt cards
vector<Card> m_dealtDeck; // Dealt cards
};
#endif
// card.h
#ifndef CARD_H
#define CARD_H
#include <iostream>
#include <string>
using namespace std;
// Sources: https://en.wikipedia.org/wiki/Standard_52-card_deck
// https://en.wikipedia.org/wiki/Pip_(counting)
const string pips[] = {"Ace", "Two", "Three", "Four", "Five",
"Six", "Seven", "Eight", "Nine", "Ten",
"Jack", "Queen", "King"};
const string suits[] = {"Hearts", "Spades", "Clubs", "Diamonds"};
class Card
{
public:
// Get card value
int get();
// Set card value
void set(int value);
string getPip();
string getSuit();
// Print card value
void print();
private:
int m_cardValue;
};
#endif
hand.h:
hand.h
#ifndef HAND_H
#define HAND_H
#include <iostream>
#include "card.h" // Include card header file here
#include "deck.h" // Include deck header file here
using namespace std;
const int NUM_CARDS_ON_HAND = 5;
class Hand
{
public:
// Put new card from deck into given location in a hand
void newCard(Deck& deck, int location);
// Get all new cards
void newHand(Deck& deck);
// Determine cards to exchange and exchange them in a hand
void exchangeCards(Deck& deck);
// Print the hand
void print();
private:
Card m_hand[NUM_CARDS_ON_HAND]; // A hand consists of 5 cards
};
#endif
How do I fix the error on line 62? I know C++ and know how to debug a program but this is a different book. I apollogize I am trying to understand it and this is the only way I'll how.
![A main.cpp - Code:Blocks 20.03
X
File Edit View Search Project Build Debug Fortran wxSmith
Tools Tools+ Plugins DoxyBlocks Settings Help
/** *<
S C
| <global>
vmain) : int
Management
Start here X main.cpp x deck.cpp X
Projects
FSymbols
Files
string repeat = "Y";
Deck myDeck;
29
Workspace
30
31
Hand myHand;
32
string exchangeCards;
33
34
while (repeat == "Y" || repeat == "y")
35
36
cout << endl;
37
38
myHand.newHand (myDeck) ;
myHand.print ();
cout << endl;
39
40
41
42
cout << "Would you like to exchange any cards? [Y / N]: ";
43
getline (cin, exchangeCards);
44
45
while (exchangeCards != "y" && exchangeCards != "y" && exchangeCards != "X" && exchangeCards != "n")
46
{
47
cout << "Please enter Y or N only: ";
48
getline (cin, exchangeCards);
49
50
51
52
if (exchangeCards == "y" || exchangeCards == "y")
53
{
54
myHand.exchangeCards (myDeck) ;
55
56
cout << endl;
57
58
myHand.print ();
cout << endl;
61
62 O
myDeck.reset () ;
// Resets the deck for a new game
63
cout << "Play again? [Y / N]: ";
getline (cin, repeat);
64
65
Logs & others
2 Code:Blocks x
Q Search results X
A Cccc X
Ở Build log X
P Build messages x CppCheck/Vera++ X 2 CppCheck/Vera++ messages X Cscope X
Z DoxyBlocks X
F Fortran info x
Closed files list X
Q Thread search x
File
Line
Message
=== Build file: "no target" in "no project" (compiler: unknown)
===
C:\Users\cccc...
In function 'int main ()':
C:\Users\ccee...
62
error: 'class Deck' has no member named 'reset'; did you mean 'resetDeck'?
=== Build failed: 1 error (s), 0 warning (s) (0 minute (s), 0 second (s)) ===
C:\Users\cccccc\Documents\Assignment 2\main.cpp
C/C++
Windows (CR+LF)
WINDOWS-1252 Line 59, Col 1, Pos 1497
Insert
Read/Write default](https://content.bartleby.com/qna-images/question/e6b9d87e-6e1d-4edc-b4b6-dbb55c2a248d/faf85042-b4f2-4d36-97ab-1014a75fe0c9/77rhsaa_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Build Brothers company in C A civil engineering company called Build Brothers has approached you to write a program to help some of their employees with getting their job quicker specially when it comes to calculating different equations in their construction projects. Currently, they use the following PDF to perform all their calculations: http://www.madison-lake.k12.oh.us/userfiles/680/Classes/16192/IED-Review%20Engineering%20Formula%20Sheet.pdf They are willing to develop a small software to calculate all the functions and calculations in the above cheat sheet. They have made this decision to reduce inherent human errors during calculation and improve efficiency. They think that these can be achieved by an “advanced calculator” that allows a user to choose an equation, provide the inputs, and calculate the outputs. Before the company invests on the final product, the have agreed on developing a proof-of-concept first. The idea is that your solution will ‘prove’ that a better…arrow_forwardA word Decrambling game using srand() for descrambling these words: "Screen, Programming, Command, Definition." The game should be timed for 1 minute or 30 seconds using the <ctime> library. Should allow for user input to be able to guess...arrow_forwardample code for the reader-writer: #include <pthread.h>#include <semaphore.h>#include <stdio.h> /*This program provides a possible solution for first readers writers problem using mutex and semaphore.I have used 10 readers and 5 producers to demonstrate the solution. You can always play with these values.*/ sem_t wrt;pthread_mutex_t mutex;int cnt = 1;int numreader = 0; void *writer(void *wno){ sem_wait(&wrt);cnt = cnt*2;printf("Writer %d modified cnt to %d\n",(*((int *)wno)),cnt);sem_post(&wrt); }void *reader(void *rno){ // Reader acquire the lock before modifying numreaderpthread_mutex_lock(&mutex);numreader++;if(numreader == 1) {sem_wait(&wrt); // If this id the first reader, then it will block the writer}pthread_mutex_unlock(&mutex);// Reading Sectionprintf("Reader %d: read cnt as %d\n",*((int *)rno),cnt); // Reader acquire the lock before modifying numreaderpthread_mutex_lock(&mutex);numreader--;if(numreader == 0) {sem_post(&wrt); //…arrow_forward
- Integer userValue is read from input. Assume userValue is greater than 1000 and less than 99999. Assign tensDigit with userValue's tens place value. Ex: If the input is 15876, then the output is: The value in the tens place is: 7 2 3 public class ValueFinder { 4 5 6 7 8 9 10 11 12 13 GHE 14 15 16} public static void main(String[] args) { new Scanner(System.in); } Scanner scnr int userValue; int tensDigit; int tempVal; userValue = scnr.nextInt(); Your code goes here */ 11 System.out.println("The value in the tens place is: + tensDigit);arrow_forwardAssign pizzasInStore's first element's caloriesInSlice with the value in pizzasInStore's second element's caloriesInSlice. Only the *your code goes here* can be affected, the rest of the program cannot be changed.Program below:--------------------------- #include <iostream>#include <vector>#include <string>using namespace std; struct PizzaInfo {string pizzaName;int caloriesInSlice;}; int main() {vector<PizzaInfo> pizzasInStore(2); cin >> pizzasInStore.at(0).pizzaName;cin >> pizzasInStore.at(0).caloriesInSlice; cin >> pizzasInStore.at(1).pizzaName;cin >> pizzasInStore.at(1).caloriesInSlice; /* Your code goes here */ cout << "A " << pizzasInStore.at(0).pizzaName << " slice contains " << pizzasInStore[0].caloriesInSlice << " calories." << endl;cout << "A " << pizzasInStore.at(1).pizzaName << " slice contains " << pizzasInStore[1].caloriesInSlice << " calories." <<…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
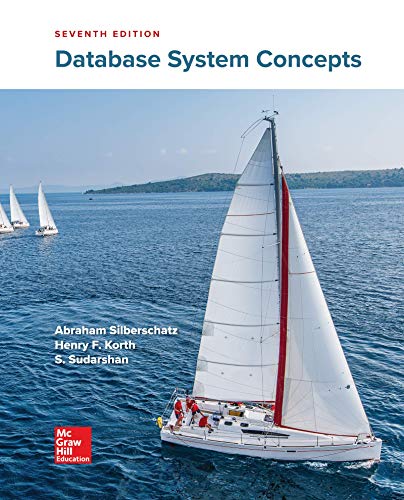
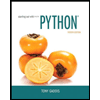
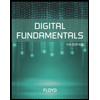
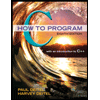
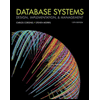
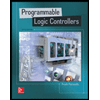