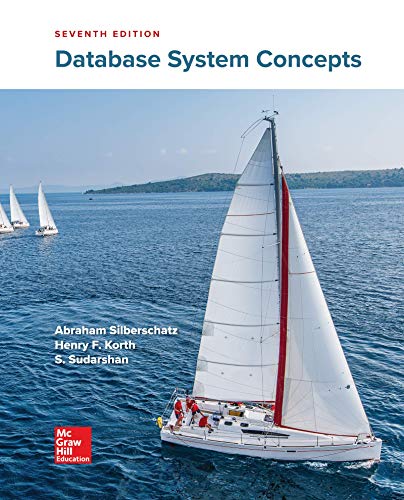
Concept explainers
CODE to Copy & Paste
#include <iostream>
#include <iomanip>
#include <fstream>
using namespace std;
void readStudData(ifstream &rss, int scores[], int id[], int &count, bool &tooMany);
float mean(int scores[], int count);
void printTable(int score[], int ID[], int count);
void printGrade(int oneScore, float average);
// Only accept MAX_SIZE entries, this will be the size of our arrays
const int MAX_SIZE = 10;
int main()
{
// variables. Need to create two int arrays: scores and ID.
// Need to create one int: count. Need to create one bool: tooMany
int scores[MAX_SIZE];
int ID[MAX_SIZE];
int count;
bool tooMany;
// Call readStudData to read data from the file that was opened above
// inFile is the name of the file opened
ifstream inFile;
inFile.open("scores.txt");
readStudData(inFile, scores, ID, count, tooMany);
// Don't forget to close the file
inFile.close();
// If there are more than MAX_SIZE records in the file, then tooMany should be
// set to true. We need to warn the user that not all the records were used
if( tooMany ) cout << "\nWarning! Some data is missing\n";
// Print the data
printTable(scores, ID, count);
cout << endl;
system("Pause");
return 0;
}
void readStudData(ifstream &rss, int scores[], int id[], int &count, bool &tooMany)
{
// Set tooMany to false and count to 0
tooMany = false;
count = 0;
while(!rss.eof())
{
if(count == MAX_SIZE) break;
rss >> id[count] >> scores[count];
count++;
}
// Determine if we have too many records in the file
if( !rss.eof() && count == MAX_SIZE ) tooMany = true;
}
float mean(int scores[], int count)
{
// Store the sum of the scores here
int sum = 0;
// Loop through and accumulate the scores from the array
for(int i = 0; i < count; i++)
{
sum += scores[i];
}
// Return the average
return (float)sum/count;
}
void printTable(int score[], int ID[], int count)
{
// Need the average
float average = mean(score, count);
// Display the average to the user
cout << "Average: " << average << endl;
// Display the header for the table
cout << "ID\t" << "Score\t" << "Grade" << endl;
for(int i = 0; i < count; i++)
{
cout << ID[i] << '\t' << score[i] << '\t';
printGrade(score[i], average);
}
}
void printGrade(int oneScore, float average)
{
if(oneScore < average + 10 && oneScore > average - 10) cout << "Satisfactory\n";
else if(oneScore > average + 10) cout << "Outstanding\n";
else cout << "Unsatisfactory\n";
}
TASK
Redo using a struct to store each student’s data and an array of structs to store the whole class. The struct should have data members for id, score, and grade.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Coorect the Following C++ code: #include <bits/stdc++.h>#include <iostream>#include <fstream>#include <string>#include <cctype> using namespace std; // Function to print available currencies for exchangevoid printCurrencies() { cout << "Available currencies for exchange: " << endl; cout << "SAR --> Saudi Arabia Riyal" << endl; cout << "KWD --> Kuwaiti Dinar" << endl; cout << "QAR --> Qatar Riyal" << endl; cout << "AED --> United Arab Emirates Dirham" << endl; cout << "BHD --> Bahraini Dinar" << endl; cout << "OMR --> Omani Rial" << endl;} // Function to convert currencydouble convertCurrency(string fromCurrency, string toCurrency, double amount) { ifstream exchangeRateFile("ExchangeRate.txt"); // Open file for reading string line; double desiredRate=0; while (getline(exchangeRateFile, line)) { // Read file…arrow_forwardC++ Code dynamicarray.h and dynamicarray.cpparrow_forward#include <bits/stdc++.h>using namespace std;int main() { double matrix[4][3]={{2.5,3.2,6.0},{5.5, 7.5, 12.6},{11.25, 16.85, 13.45},{8.75, 35.65, 19.45}}; cout<<"Input no in first row of matrix"<<endl; for(int i=0;i<3;i++){ double t; cin>>t; matrix[0][i]=t; } cout<<"Contents of the last column in matrix"<<endl; for(int i=0;i<4;i++){ cout<<matrix[i][2]<<" "; } cout<<"Content of first row and last column element in matrix is: "<<matrix[0][3]<<endl; matrix[3][2]=13.6; cout<<"Updated matrix is :"<<endl; for(int i=0;i<4;i++){ for(int j=0;j<3;j++){ cout<<matrix[i][j]<<" "; }cout<<endl; } return 0;} Please explain this codearrow_forward
- Code in C Code in the file IO: /************************************************************* This program prints a degree-to-radian table using a for- loop structure. The results are printed to a file and the the screen. *************************************************************/ #include <stdio.h> #define PI 3.141593 #define FILENAME "tableD2R.dat" int main(void) { /* Declare variables. */ double radians; FILE *fileout; /* Open file. */ fileout = fopen(FILENAME,"w"); if (fileout == NULL) printf("Error opening input file. \n"); else { /* Print radians and degrees in a loop. */ printf("Degrees to Radians \n"); for (int degrees=0; degrees<=360; degrees+=10) { radians = degrees*PI/180; printf("%6i %9.6f \n",degrees,radians); fprintf(fileout,"%6i %9.6f \n",degrees,radians); } /* Exit program. */ }arrow_forwardCode in c++ pleasearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
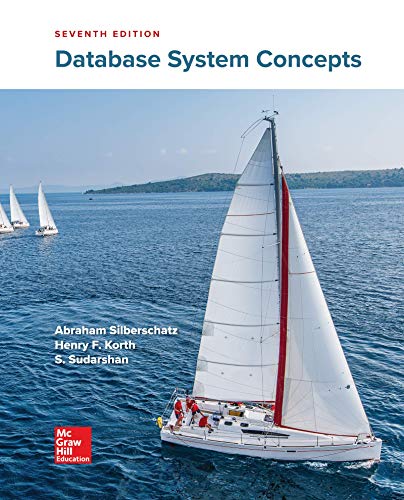
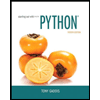
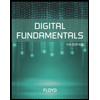
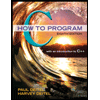
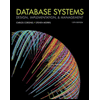
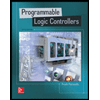