#include using namespace std; double average(int sum_of_grades,int num_grades) { return sum_of_grades/(float)num_grades; } int main() { int num_grades,grade,sum=0; char grade_value; cout<<"Enter the number of grades"<>num_grades; for(int i=0;i>grade; sum+=grade; } double avg=average(sum,num_grades); if(avg>=90 && avg<=100) grade_value='A'; else if(avg>=80 && avg<=89) grade_value='B'; else if(avg>=70 && avg<=79) grade_value='C'; else if(avg>=60 && avg<=69) grade_value='D'; else if(avg>=0 && avg<=59) grade_value='F'; cout<<"The grade is "<
#include <iostream>
using namespace std;
double average(int sum_of_grades,int num_grades)
{
return sum_of_grades/(float)num_grades;
}
int main() {
int num_grades,grade,sum=0;
char grade_value;
cout<<"Enter the number of grades"<<endl;
cin>>num_grades;
for(int i=0;i<num_grades;i++)
{
cout<<"Enter a numeric grade between 0-100"<<endl;
cin>>grade;
sum+=grade;
}
double avg=average(sum,num_grades);
if(avg>=90 && avg<=100)
grade_value='A';
else if(avg>=80 && avg<=89)
grade_value='B';
else if(avg>=70 && avg<=79)
grade_value='C';
else if(avg>=60 && avg<=69)
grade_value='D';
else if(avg>=0 && avg<=59)
grade_value='F';
cout<<"The grade is "<<grade_value;
}
review if the written c++ code is correct then organize the code and write comments for each part of the program explaining what they do.
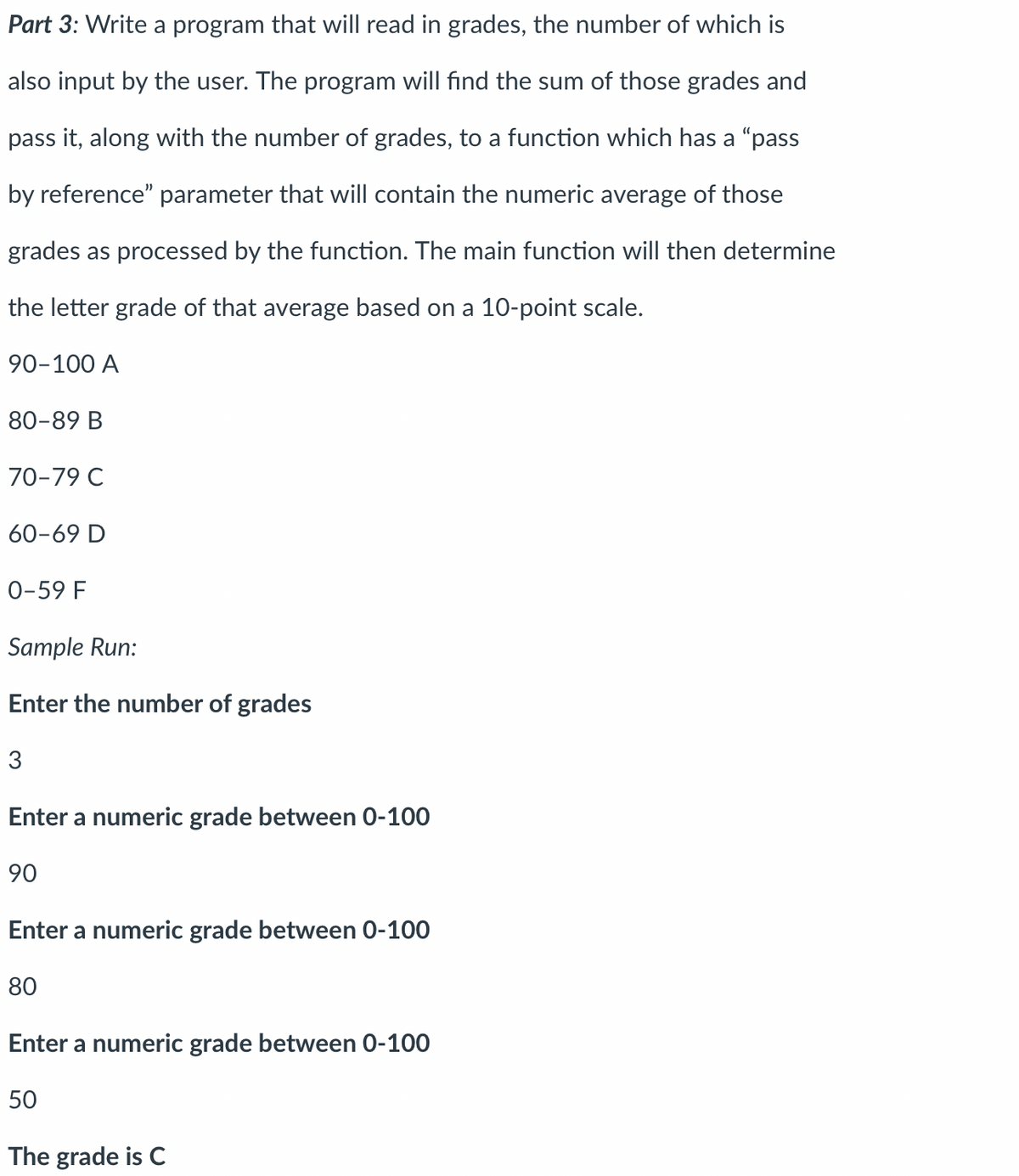

Step by step
Solved in 3 steps with 1 images

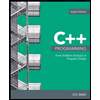
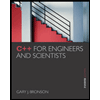
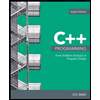
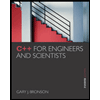